Optimizing Java Code with Groovy: A Walkthrough
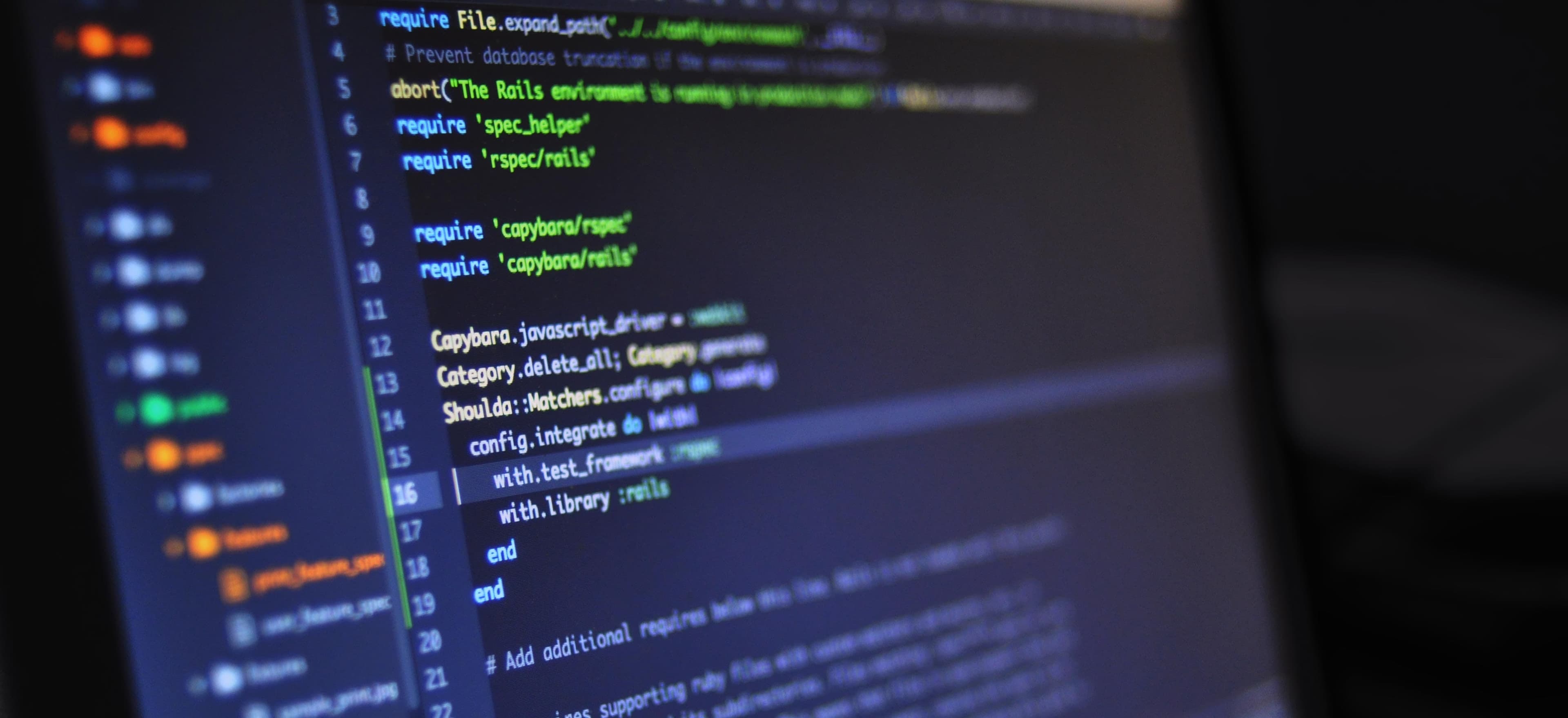
- Published on
Optimizing Java Code with Groovy: A Walkthrough
In the world of software development, optimization is a crucial aspect of writing efficient and performant code. Java, being a popular and powerful programming language, offers a myriad of ways to optimize code for better performance. In this blog post, we will explore how we can leverage Groovy, a powerful scripting language that runs on the Java Virtual Machine, to optimize Java code.
What is Groovy?
Groovy is a powerful, optionally-typed and dynamic language that seamlessly integrates with Java. It provides features such as closures, dynamic typing, and seamless Java integration, making it an attractive choice for scripting tasks and rapid application development. Groovy's syntax is concise and expressive, allowing for more streamlined code compared to Java.
Let's dive into a few ways we can optimize Java code using Groovy:
1. Using Groovy's Collection Methods
Java Code:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = 0;
for (int number : numbers) {
sum += number;
}
double average = (double) sum / numbers.size();
Groovy Code:
def numbers = [1, 2, 3, 4, 5]
def sum = numbers.sum()
def average = numbers.sum() / numbers.size()
In the Java code, we have to manually iterate through the list of numbers to calculate the sum. In Groovy, we can achieve the same result using the sum()
method, which is concise and more expressive. This showcases how Groovy's collection methods can simplify code and make it more readable.
2. Working with Files and I/O
Java Code:
try (BufferedReader br = new BufferedReader(new FileReader(file))) {
String line;
while ((line = br.readLine()) != null) {
// Process the line
}
}
Groovy Code:
file.eachLine { line ->
// Process the line
}
In the Java code, we have to open a BufferedReader
and manually read lines from the file. Groovy provides the eachLine
method, which simplifies the process of reading lines from a file, making the code more concise and expressive.
3. Leveraging Groovy's Closures
Java Code:
List<String> words = Arrays.asList("apple", "banana", "cherry");
words.forEach(word -> {
System.out.println(word);
});
Groovy Code:
def words = ["apple", "banana", "cherry"]
words.each { word ->
println word
}
In the Java code, we use a lambda expression to iterate through the list of words. Groovy's closures provide a more concise and expressive way to achieve the same result, making the code more readable.
4. Simplifying String Manipulation
Java Code:
String prefix = "Hello, ";
String name = "John";
String message = prefix + name + "!";
Groovy Code:
def prefix = "Hello, "
def name = "John"
def message = "$prefix$name!"
In the Java code, string manipulation involves using concatenation to build the final message. Groovy provides string interpolation, allowing for more concise and readable string manipulation.
5. Exploiting Groovy's Metaprogramming Features
Java Code:
public class MyClass {
public void doSomething() {
System.out.println("Doing something");
}
}
MyClass obj = new MyClass();
Groovy Code:
class MyClass {
def doSomething() {
println "Doing something"
}
}
def obj = new MyClass()
In the Java code, we have to define a method using an explicit return type and use System.out.println
for output. Groovy allows us to define methods using def
for dynamic typing and provides concise output using println
, showcasing the power of Groovy's metaprogramming features.
Bringing It All Together
In this blog post, we have explored various ways of optimizing Java code using the power of Groovy. From simplifying collection operations to leveraging Groovy's dynamic typing and metaprogramming features, there are numerous opportunities to streamline and optimize Java code using Groovy. By incorporating Groovy into our Java projects, we can write more expressive, concise, and efficient code, ultimately leading to improved performance and maintainability.
So, next time you find yourself writing Java code, consider harnessing the capabilities of Groovy to optimize your code and enhance your development experience.
Start incorporating Groovy into your Java projects today and experience the power of optimized code firsthand!
Checkout our other articles