Solving Java’s JDBC Bind Issues with Date and Float Types
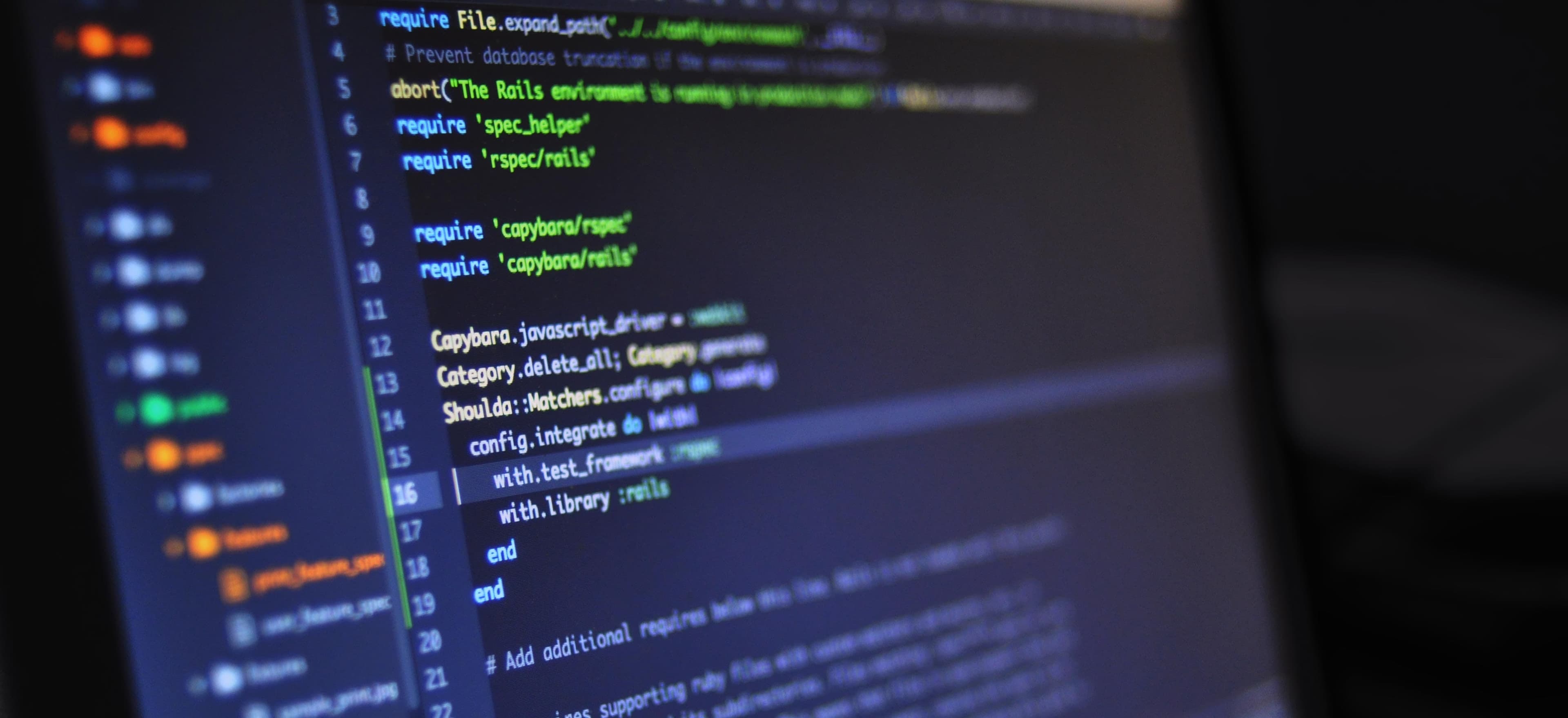
- Published on
Solving Java’s JDBC Bind Issues with Date and Float Types
When working with Java Database Connectivity (JDBC), developers often encounter challenges similar to the ones faced in PHP’s PDO when handling data types like Date
and Float
. These challenges can hinder seamless database operations, particularly in applications that demand precise data handling. In this blog, we will explore effective strategies for solving JDBC bind issues related to these data types, while providing examples to illustrate best practices.
Understanding JDBC Basics
At its core, JDBC provides a standard API for Java applications to interact with relational databases. This functionality is crucial for any Java developer, especially when building data-driven applications. However, utilizing JDBC can be tricky due to various data type incompatibilities, leading to unexpected results or runtime exceptions.
One common roadblock is dealing with Java's handling of dates and floating-point numbers. Let's dive into these issues, starting with dates.
JDBC Date Types: A Common Pitfall
When working with dates in JDBC, developers must differentiate between the SQL DATE
, TIME
, and TIMESTAMP
types. Consider the following straightforward example of how to insert a date using JDBC:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.sql.Date;
public class DateExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
String insertSQL = "INSERT INTO my_table (date_column) VALUES (?)";
try (Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement(insertSQL)) {
// Converting java.util.Date to java.sql.Date
java.util.Date utilDate = new java.util.Date();
Date sqlDate = new Date(utilDate.getTime());
pstmt.setDate(1, sqlDate); // Set the SQL Date
pstmt.executeUpdate(); // Execute the insert
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Why Use java.sql.Date
?
In the above example, we convert a java.util.Date
object to a java.sql.Date
object. This conversion is necessary because JDBC is designed to work best with java.sql.Date
, java.sql.Time
, and java.sql.Timestamp
types. Using these specific SQL types ensures that date values are correctly interpreted by the database.
Common Mistakes
-
Using
java.util.Date
Directly: Directly passingjava.util.Date
toPreparedStatement
can lead to unexpected behavior. Always convert it tojava.sql.Date
. -
Time Zone Issues: Be aware of time zone discrepancies when inserting dates as the database might interpret them differently than you expect.
Related Resource
For developers familiar with similar issues in PHP’s PDO, there’s a helpful article titled "Fix PDO BindParam Issues with MySQL Date & Float Types" that provides valuable insights into binding parameters for floating-point and date types.
Handling Float Types in JDBC
Handling floating-point numbers such as FLOAT
and DOUBLE
in JDBC can also present challenges. Let’s consider the correct way to bind these types:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class FloatExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
String insertSQL = "INSERT INTO my_table (float_column) VALUES (?)";
try (Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement(insertSQL)) {
float floatValue = 123.45f;
pstmt.setFloat(1, floatValue); // Set the float value
pstmt.executeUpdate(); // Execute the insert
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Why Use pstmt.setFloat()
?
Using pstmt.setFloat()
allows JDBC to handle the conversion between Java's primitive float
and the underlying database representation properly. This ensures precision when storing floating-point numbers in the database.
Common Pitfalls with Floats
-
Precision Loss: Floating-point arithmetic can lead to precision issues. For highly accurate financial calculations, consider using
BigDecimal
instead. -
Wrong Data Types: Ensure the database column type matches the Java type. Using
DOUBLE
in the database but binding aFLOAT
in Java might lead to issues.
Benefits of Correct Binding
-
Error Reduction: Properly binding types reduces the chances of SQL exceptions, making your database interactions smoother.
-
Performance: Correct data types can improve query performance, as the database doesn’t have to do unnecessary type conversions.
Summary
In this article, we explored the complexities developers face with JDBC when binding Date
and Float
types. We examined the proper ways to handle Java's date and float types with JDBC while emphasizing the importance of using the correct SQL types.
As a recap:
- Always convert
java.util.Date
tojava.sql.Date
. - When dealing with floating-point numbers, ensure you use the appropriate methods to avoid common pitfalls.
- Consult related resources like the article on PHP PDO for more insights on binding issues.
By ensuring that the correct data types are used while binding parameters, you can significantly enhance the reliability and efficiency of your Java applications. Happy coding!
Checkout our other articles