Reducing Bugs Through Advanced Type Checking
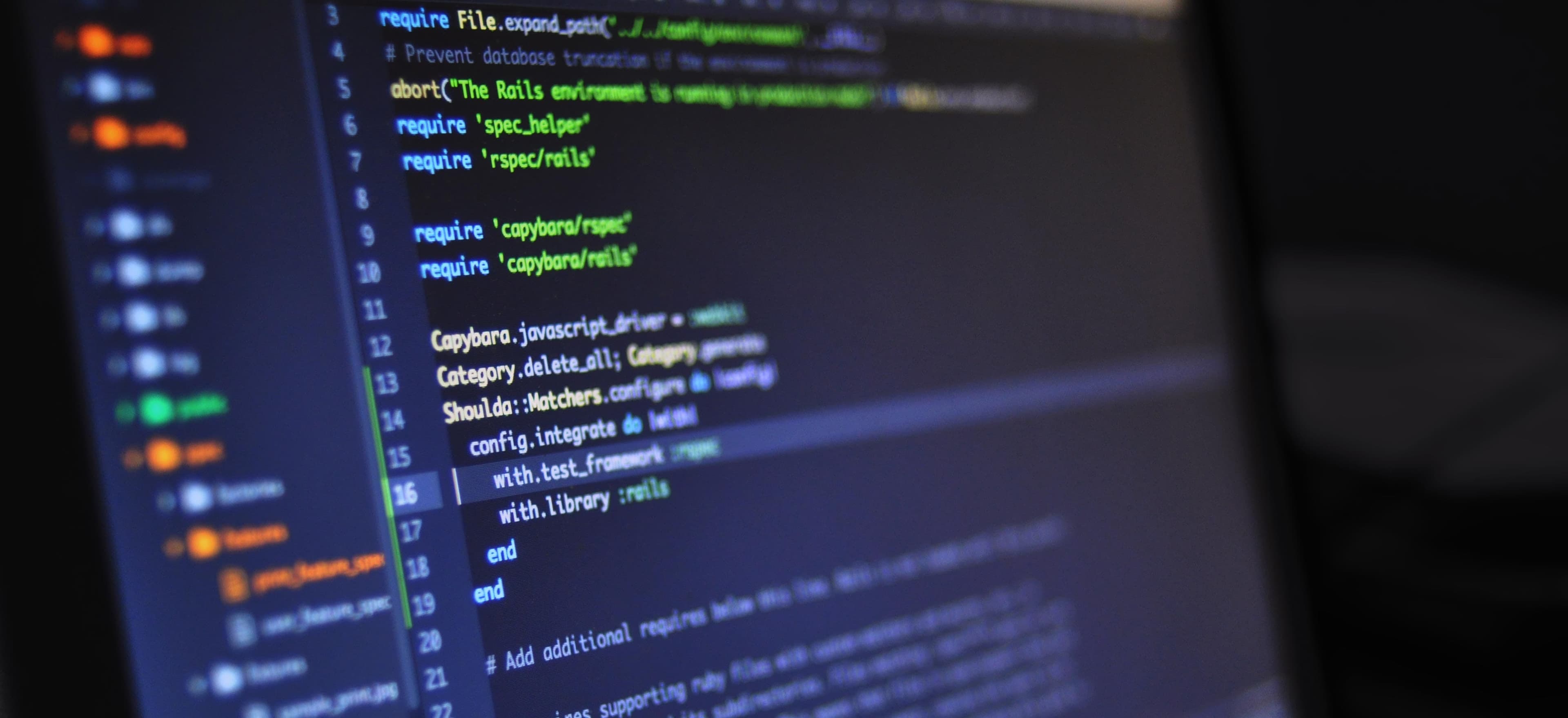
- Published on
Reducing Bugs Through Advanced Type Checking in Java
As developers, we strive to write bug-free code, but we all know that bugs lurk in the shadows, waiting to pounce on the unsuspecting. One of the most common sources of bugs in Java applications is type-related issues. Thankfully, Java provides advanced type checking features that can help us catch these bugs early in the development process.
The Problem with Type-Related Bugs
Type-related bugs occur when we try to use a variable or a method in a way that is not compatible with its declared type. This can lead to runtime errors, unexpected behavior, and, in the worst-case scenario, system crashes. Consider the following example:
List<String> names = new ArrayList<>();
names.add("Alice");
names.add(42); // Oops, adding an integer instead of a string
In this example, we have a list of strings, but we accidentally add an integer to it. This code compiles without any errors, but it will fail at runtime when we try to retrieve the elements as strings.
Enter Advanced Type Checking
To mitigate type-related bugs, Java offers advanced type checking features that can be used to enforce strict type rules at compile time. Let's delve into some of these features and see how they can help us write more robust code.
1. Generics
Generics allow us to define classes, interfaces, and methods with placeholders for the data types they operate on. By using generics, we can make our code more type-safe and reduce the chances of type-related errors.
List<String> names = new ArrayList<>();
names.add("Alice");
names.add(42); // Compilation error: incompatible types
With generics, the compiler can catch the type mismatch at compile time and prevent us from adding an integer to a list of strings.
2. Enum Types
Enum types allow us to define a set of constants with a fixed type. By using enums, we can ensure that certain variables take only specific predefined values, thus reducing the likelihood of runtime errors.
enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY
}
Day today = Day.MONDAY;
today = "Monday"; // Compilation error: incompatible types
In this example, the compiler will catch the type mismatch when we try to assign a string to a variable of type Day, thus preventing a potential runtime error.
3. Type Inference
Java's type inference feature allows the compiler to automatically determine the data type of a variable based on the context in which it is used. By leveraging type inference, we can write more concise code without sacrificing type safety.
Map<String, List<Integer>> numberMap = new HashMap<>();
In this example, we don't have to explicitly specify the types on the right-hand side of the assignment, as the compiler can infer the types based on the declarations on the left-hand side.
Leveraging Advanced Type Checking for Bug Reduction
Now that we've explored some of the advanced type checking features in Java, let's discuss how we can leverage these features to reduce bugs in our code.
-
Compile-Time Safety: By utilizing generics, enum types, and type inference, we can catch type-related bugs at compile time, thus preventing them from surfacing in production.
-
Improved Readability: Using advanced type checking features often leads to more expressive and readable code. By clearly defining types and constraints, we make the code more understandable to fellow developers and easier to maintain.
-
Reduced Debugging Efforts: The earlier we catch bugs, the less time we spend debugging. Advanced type checking helps us identify potential issues before they manifest as runtime errors, saving us valuable development and troubleshooting time.
-
Enhanced Code Quality: By embracing advanced type checking, we inherently raise the quality of our code. This proactive approach to type safety demonstrates a commitment to writing robust, reliable software.
A Final Look
In conclusion, advanced type checking features in Java, such as generics, enum types, and type inference, play a crucial role in reducing bugs and enhancing the overall quality of our code. By leveraging these features, we can catch type-related issues early in the development cycle, leading to more robust, maintainable, and bug-free software.
So, let's embrace advanced type checking and make our Java code more resilient to bugs!
Remember, the fewer bugs there are, the happier developers and users will be.
For further reading on the importance of type safety and advanced type checking, check out Effective Java by Joshua Bloch and Java Generics and Collections by Maurice Naftalin and Philip Wadler.
Happy coding!