Optimizing Java Collections with Filtering and Transformation
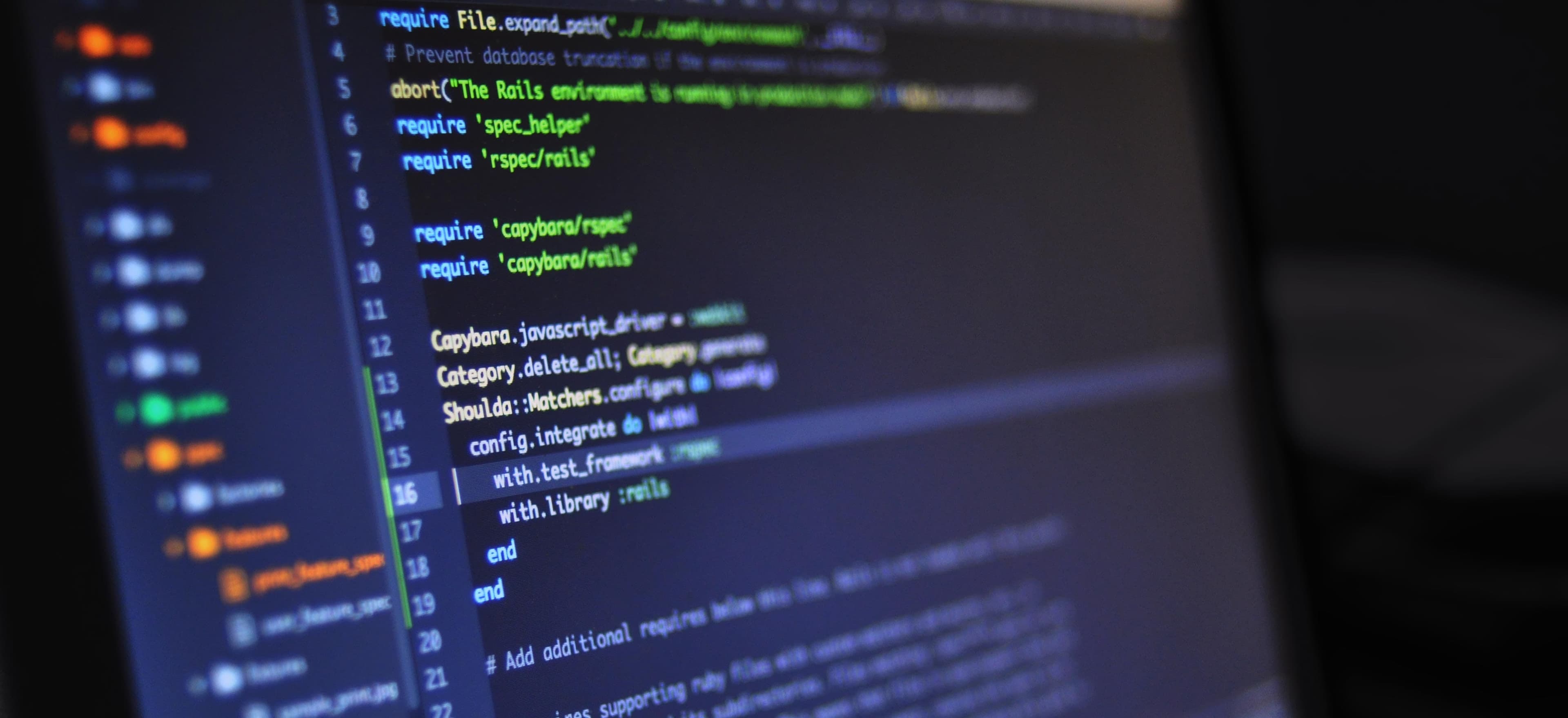
- Published on
Optimizing Java Collections with Filtering and Transformation
When working with Java collections, it's vital to optimize their performance while maintaining the code's readability. In this blog post, we'll explore how to use filtering and transformation techniques to achieve efficient and clean code using Java streams and lambdas.
Filtering Collections
Filtering a collection means selecting specific elements based on certain criteria. Traditionally, this would involve writing loops and conditional statements. However, with Java 8 streams, the process is more streamlined and expressive.
Let's consider a scenario where we have a list of Person
objects and we want to filter out the adults (persons older than 18 years).
List<Person> people = ... // Populate the list
List<Person> adults = people.stream()
.filter(person -> person.getAge() > 18)
.collect(Collectors.toList());
In this example, we utilize the filter
method of the stream to select only the Person
objects that satisfy the given condition. The resulting List
contains only the adult individuals, without the need for explicit iteration or condition checks.
Transformation of Collections
In addition to filtering, transforming a collection involves modifying elements based on certain criteria. This can be achieved elegantly using Java streams and lambda expressions.
Let's say we want to transform a list of Person
objects into a list of their respective names.
List<Person> people = ... // Populate the list
List<String> names = people.stream()
.map(Person::getName)
.collect(Collectors.toList());
In this case, we use the map
method to transform each Person
object into their names, resulting in a List
of strings containing the names of all the individuals in the original list.
Combining Filtering and Transformation
Often, we may need to perform both filtering and transformation in a single operation. With Java streams, this can be achieved concisely.
Consider a scenario where we need to filter the adult Person
objects and then transform the filtered list into a list of their ages.
List<Person> people = ... // Populate the list
List<Integer> adultAges = people.stream()
.filter(person -> person.getAge() > 18)
.map(Person::getAge)
.collect(Collectors.toList());
Here, we first filter the Person
objects based on the age condition and then map the filtered objects to retrieve their ages, resulting in a List
of integers containing the ages of the adult individuals.
Chaining Operations
Java streams allow us to chain multiple operations together, leading to concise and expressive code. This approach is not only efficient but also enhances the readability of the code.
List<String> result = someList.stream()
.filter(...)
.map(...)
.distinct()
.sorted()
.collect(Collectors.toList());
In this example, we demonstrate chaining operations such as filtering, mapping, removing duplicates, sorting, and collecting the results into a new list. This chain clearly expresses the sequence of operations performed on the collection.
When to Opt for Streams
It's essential to note that while streams provide a succinct and expressive way to handle collections, they may not always be the most performant option, especially for smaller collections or simple operations. In such cases, traditional iteration may be more efficient.
When working with large collections and complex operations, streams offer parallel processing, taking advantage of multi-core processors for enhanced performance. However, it's crucial to measure and compare the performance of stream operations versus traditional iterations for your specific use case.
Final Considerations
In this blog post, we've explored how filtering and transformation can be efficiently achieved using Java streams and lambdas. By leveraging these techniques, we can write cleaner and more maintainable code while optimizing the performance of our collections.
Integrating filtering, transformation, and chaining operations not only improves code readability but also enables us to express our logic in a more declarative and functional style. As always, it's important to consider the trade-offs and performance implications when choosing between traditional iteration and stream operations.
By mastering these techniques, Java developers can ensure their code is both efficient and elegant, delivering the best of both worlds in terms of performance and maintainability.
For further insights into Java collections and stream operations, you may explore the official Java Collections documentation and the Java Stream API documentation.