Optimizing TensorFlow Model Deployment with Kafka for Data Scientists
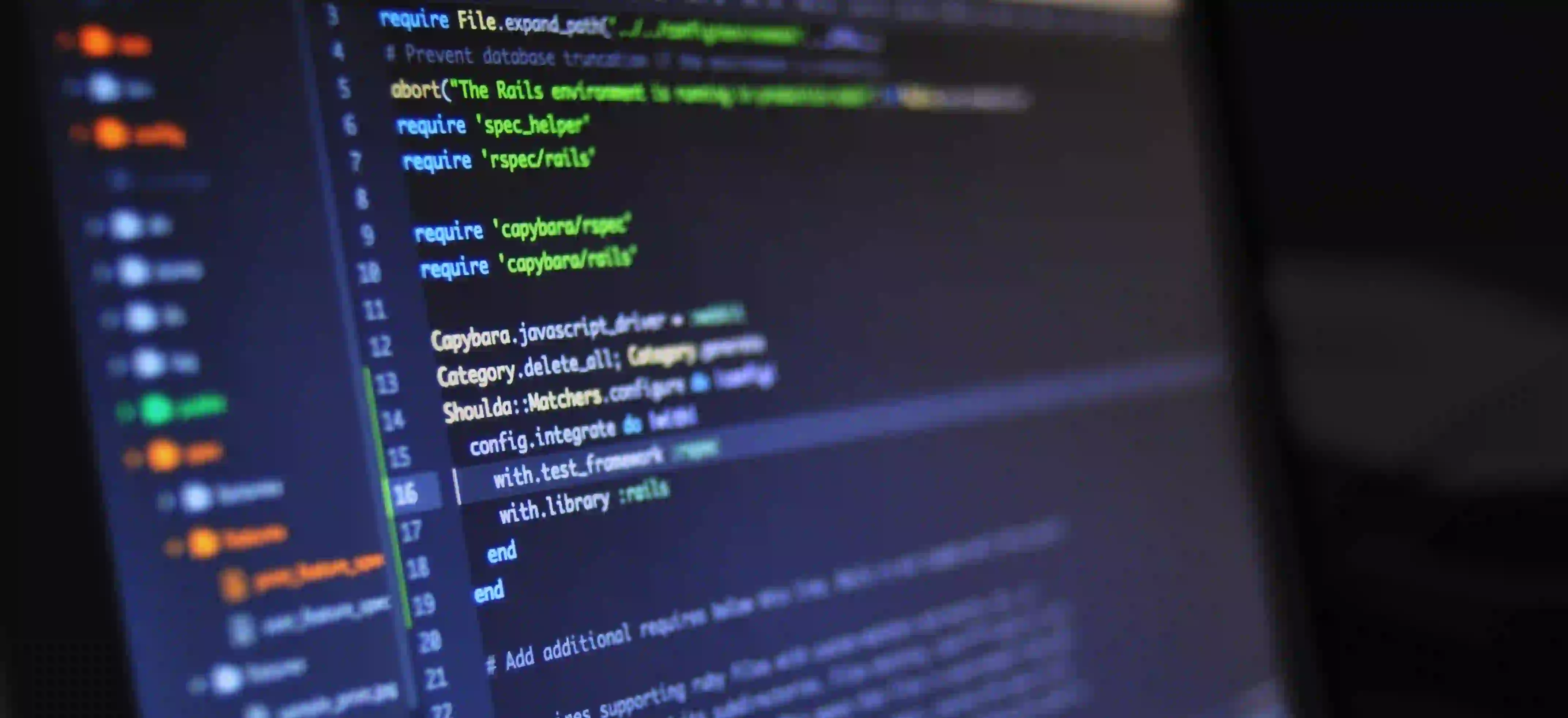
Optimizing TensorFlow Model Deployment with Kafka for Data Scientists
In the current scenario, data scientists are constantly seeking ways to optimize model deployment, blending effectiveness with efficiency. One promising approach involves integrating TensorFlow models with Kafka, a distributed streaming platform.
Understanding TensorFlow and Kafka
TensorFlow, an open-source machine learning framework, provides a comprehensive ecosystem for building and deploying machine learning models. On the other hand, Kafka facilitates the building of real-time data pipelines and streaming applications. By combining these two technologies, data scientists can streamline the deployment of TensorFlow models in a distributed and efficient manner.
Integrating TensorFlow with Kafka
Let's delve into the integration of TensorFlow with Kafka, leveraging the advantages offered by both technologies.
Setting Up Kafka
First, it’s imperative to set up Kafka and create a topic where the data will be produced and consumed. Install Kafka and create a topic using the following commands:
# Start Zookeeper
bin/zookeeper-server-start.sh config/zookeeper.properties
# Start Kafka
bin/kafka-server-start.sh config/server.properties
# Create a topic
bin/kafka-topics.sh --create --topic my_topic --bootstrap-server localhost:9092 --partitions 1 --replication-factor 1
Producing Data to Kafka
Next, data needs to be produced to the Kafka topic using a simple Python script or any framework of choice. The script can publish data from TensorFlow models to the Kafka topic.
Consuming Data from Kafka
In the deployment environment, a consumer application can be set up to receive data from the Kafka topic, where the data can then be consumed and utilized for inference or further processing.
Benefits of TensorFlow-Kafka Integration
The integration of TensorFlow with Kafka offers several benefits:
-
Real-time Model Deployment: By leveraging Kafka's real-time data streaming capabilities, TensorFlow models can be deployed and updated in real-time, ensuring the most current models are in use.
-
Scalability: Kafka’s distributed nature allows for scalability, enabling the deployment of TensorFlow models across multiple nodes and scaling to handle increased inference workloads.
-
Fault Tolerance: Kafka’s fault-tolerant architecture ensures that data is not lost in case of node failures, providing reliability for model deployment.
Java Implementation for TensorFlow-Kafka Integration
Now, let's delve into a Java implementation of the TensorFlow-Kafka integration. Below is a simple example demonstrating how to produce data from a TensorFlow model and consume it using a Kafka consumer in Java.
Producing Data to Kafka in Java
The following Java code snippet demonstrates how to produce data from a TensorFlow model to a Kafka topic:
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerRecord;
import org.apache.kafka.clients.producer.ProducerConfig;
import org.apache.kafka.common.serialization.StringSerializer;
import java.util.Properties;
public class TensorFlowKafkaProducer {
public static void main(String[] args) {
String topicName = "my_topic";
Properties props = new Properties();
props.put(ProducerConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
props.put(ProducerConfig.KEY_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName());
props.put(ProducerConfig.VALUE_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName());
KafkaProducer<String, String> producer = new KafkaProducer<>(props);
// Generate data from TensorFlow model
String dataFromModel = "sample_data_from_tensorflow_model";
ProducerRecord<String, String> record = new ProducerRecord<>(topicName, dataFromModel);
producer.send(record);
}
}
In this code, we create a Kafka producer and send data generated from a TensorFlow model to the Kafka topic.
Consuming Data from Kafka in Java
Now, let's look at how to consume the data from the Kafka topic using a Java consumer:
import org.apache.kafka.clients.consumer.KafkaConsumer;
import org.apache.kafka.clients.consumer.ConsumerRecords;
import org.apache.kafka.clients.consumer.ConsumerRecord;
import org.apache.kafka.clients.consumer.ConsumerConfig;
import org.apache.kafka.common.serialization.StringDeserializer;
import java.util.Collections;
import java.util.Properties;
public class TensorFlowKafkaConsumer {
public static void main(String[] args) {
String topicName = "my_topic";
Properties props = new Properties();
props.put(ConsumerConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
props.put(ConsumerConfig.GROUP_ID_CONFIG, "group1");
props.put(ConsumerConfig.KEY_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class.getName());
props.put(ConsumerConfig.VALUE_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class.getName());
KafkaConsumer<String, String> consumer = new KafkaConsumer<>(props);
consumer.subscribe(Collections.singletonList(topicName));
while(true){
ConsumerRecords<String, String> records = consumer.poll(100);
for(ConsumerRecord<String, String> record : records){
// Consume data and perform necessary processing
System.out.println("Received data: " + record.value());
// Perform TensorFlow model inference or further processing
}
}
}
}
In this code, we create a Kafka consumer that subscribes to the topic and consumes the data from the Kafka topic. The consumed data can then be utilized for TensorFlow model inference or any further processing.
The Last Word
In conclusion, the integration of TensorFlow with Kafka offers a powerful solution for optimizing model deployment by combining real-time streaming capabilities with machine learning inference. Data scientists can leverage this integration to deploy TensorFlow models efficiently in distributed environments while ensuring real-time updates and scalability.
By utilizing the Java implementation provided, data scientists can seamlessly integrate TensorFlow models with Kafka for streamlined and efficient deployment, opening up new avenues for real-time machine learning applications.