Managing Dependencies in Your Codebase
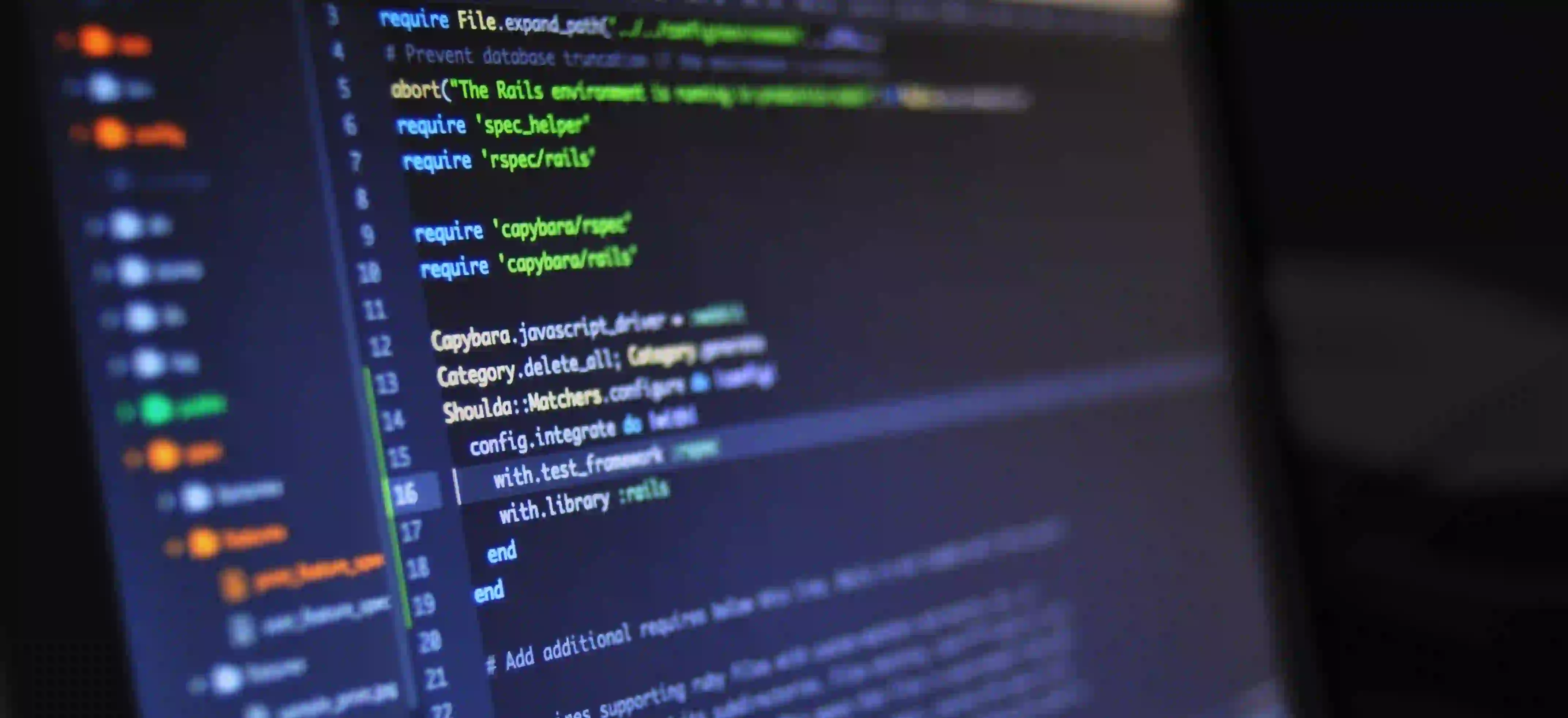
A Guide to Managing Dependencies in Your Java Codebase
As a Java developer, you're likely familiar with the importance of managing dependencies in your codebase. Dependencies are external libraries or modules that your project relies on to function properly. While dependencies can bring great functionality and efficiency to your code, managing them effectively is crucial to the overall health and maintainability of your project.
In this guide, we'll explore best practices for managing dependencies in your Java codebase, including using build tools like Maven and Gradle, understanding transitive dependencies, and ensuring version compatibility.
Using Build Tools for Dependency Management
Maven
Maven is a powerful build tool that provides a comprehensive approach to managing project dependencies. In your pom.xml
file, you can declare your project's dependencies, and Maven will handle the process of downloading the necessary libraries and adding them to your classpath.
Here's an example of declaring a dependency in a Maven project:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
Maven's central repository makes it easy to find and include dependencies from a wide range of libraries, simplifying the process of managing external dependencies in your Java projects.
Gradle
Gradle is another popular build automation tool that offers a flexible approach to dependency management. Using a Groovy or Kotlin DSL, you can define your project's dependencies in the build.gradle
file.
Here's an example of declaring a dependency in a Gradle project:
dependencies {
implementation 'org.apache.commons:commons-lang3:3.12.0'
}
Gradle offers advanced features such as incremental builds and parallel task execution, providing an efficient and customizable way to manage dependencies in your Java codebase.
Understanding Transitive Dependencies
When you declare a dependency for your project, it may have its own dependencies, known as transitive dependencies. These transitive dependencies are brought into your project transitively and must be managed alongside your direct dependencies.
For example, if your project relies on the Apache HttpClient library, which in turn relies on the Apache Commons Logging library, both the HttpClient and Commons Logging libraries become transitive dependencies of your project.
It's essential to have an understanding of transitive dependencies to avoid conflicts and ensure that all required libraries are available in your project.
Ensuring Version Compatibility
As your project grows, you may find yourself relying on multiple libraries that have their own sets of dependencies. In such cases, ensuring version compatibility among the various dependencies becomes crucial to prevent conflicts and runtime errors.
For example, if Library A requires version 2.0 of a particular dependency, and Library B requires version 3.0 of the same dependency, you will need to find a compatible version or use a technique like shading to prevent conflicts.
Excluding Unwanted Transitive Dependencies
There may be scenarios where a transitive dependency brought in by one of your direct dependencies is unnecessary for your project. In such cases, build tools like Maven and Gradle allow you to exclude specific transitive dependencies.
Here's an example of excluding a transitive dependency in a Gradle project:
configurations {
implementation {
exclude group: 'org.unwanted', module: 'unwanted-module'
}
}
By excluding unwanted transitive dependencies, you can keep your project lean and avoid unnecessary bloat.
My Closing Thoughts on the Matter
Effectively managing dependencies in your Java codebase is a crucial aspect of building and maintaining high-quality software. By using build tools like Maven and Gradle, understanding transitive dependencies, ensuring version compatibility, and excluding unwanted dependencies, you can streamline the process of managing external libraries and modules in your projects.
By following best practices and staying proactive about dependency management, you can keep your codebase organized, efficient, and resilient to potential issues that may arise from conflicting or unnecessary dependencies.
Remember, a well-managed dependency strategy is a cornerstone of a successful and maintainable Java codebase.