Optimizing Memory Usage in Java Collections
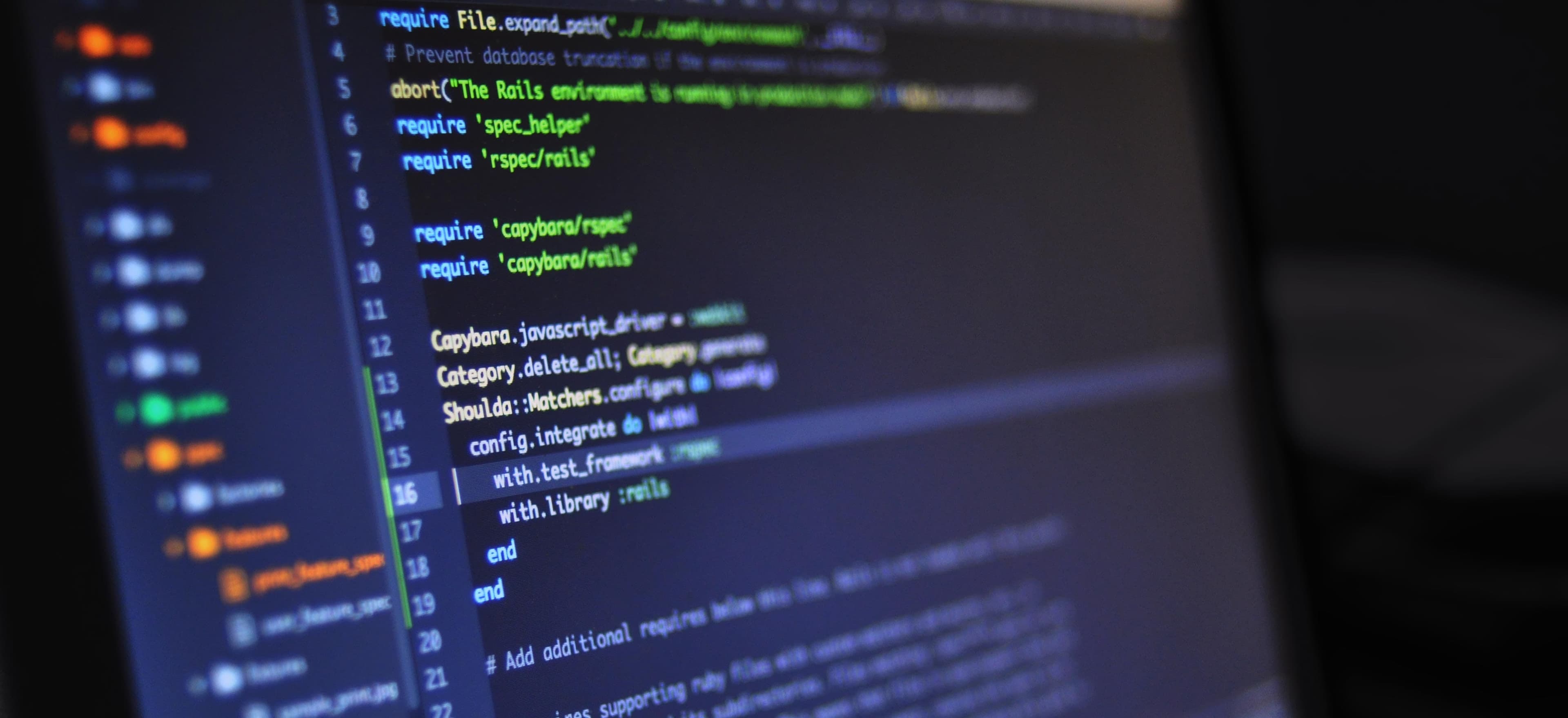
- Published on
Optimizing Memory Usage in Java Collections
When it comes to writing efficient and high-performing Java applications, optimizing memory usage plays a crucial role. In Java, collections are fundamental data structures that are extensively used in a wide range of applications. However, inefficient use of collections can lead to increased memory consumption and performance issues. In this article, we will explore some best practices for optimizing memory usage in Java collections.
Use the Right Collection Type
Choosing the right collection type is essential for optimizing memory usage. Different collection types have different memory footprints and performance characteristics. For example, if you only need a simple key-value mapping and don't require duplicate keys, using HashMap
instead of TreeMap
can save memory and improve performance.
// Bad practice: Using TreeMap when a HashMap will suffice
Map<String, String> badMap = new TreeMap<>();
// Good practice: Using HashMap for better memory usage
Map<String, String> goodMap = new HashMap<>();
In the above example, using HashMap
instead of TreeMap
can result in better memory optimization, especially for large datasets.
Use the Right Initial Capacity
Java collections allow you to specify an initial capacity when creating instances. Providing an appropriate initial capacity can help reduce memory overhead by avoiding frequent rehashing and resizing. However, setting the initial capacity too high can also waste memory. It's essential to strike a balance based on the expected size of the collection.
// Bad practice: Setting initial capacity too low
Map<String, String> badInitialCapacityMap = new HashMap<>(10);
// Good practice: Setting initial capacity based on expected size
Map<String, String> goodInitialCapacityMap = new HashMap<>(1000);
By setting the initial capacity based on the expected size, unnecessary rehashing and resizing can be minimized, leading to optimized memory usage.
Minimize Auto-Boxing
Auto-boxing, the automatic conversion of primitive types to their corresponding wrapper types, can lead to increased memory usage and reduced performance. When working with collections, it's crucial to minimize auto-boxing by using the appropriate collection types that support primitives directly, such as TIntArrayList
from the Trove library or IntArrayList
from the Eclipse Collections library.
// Bad practice: Using ArrayList with auto-boxing for primitive int
List<Integer> badList = new ArrayList<>();
badList.add(10);
// Good practice: Using specialized collection for primitive int
TIntArrayList goodList = new TIntArrayList();
goodList.add(10);
By using specialized collections for primitives, unnecessary object creation and memory overhead associated with auto-boxing can be avoided, resulting in optimized memory usage.
Choose Immutable Collections Wisely
Immutable collections, such as those provided by Google's Guava library or Java 9's List.of()
, Set.of()
, and Map.of()
, offer several benefits, including thread safety and minimal memory overhead. However, it's essential to choose immutable collections wisely and consider their memory implications, especially when dealing with large datasets.
// Bad practice: Using immutable collection for a large dataset
List<String> badImmutableList = ImmutableList.copyOf(largeList);
// Good practice: Choosing immutable collections judiciously for memory efficiency
List<String> goodImmutableList = largeList.subList(0, largeList.size());
While immutable collections offer benefits such as thread safety, excessive use of these collections for large datasets can result in increased memory consumption. Choosing immutable collections judiciously by considering memory implications is essential for optimization.
Avoid Unnecessary Object Creation
Creating unnecessary objects within collections can contribute to increased memory usage and garbage collection overhead. It's crucial to avoid unnecessary object creation, especially within loops and when dealing with complex data structures.
// Bad practice: Creating unnecessary String objects within a loop
List<String> badList = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
badList.add(new String("value" + i));
}
// Good practice: Reusing existing String objects within a loop
List<String> goodList = new ArrayList<>();
String commonValue = "value";
for (int i = 0; i < 1000; i++) {
goodList.add(commonValue + i);
}
By reusing existing objects and avoiding unnecessary object creation, memory overhead can be reduced, leading to improved memory usage and overall performance.
Use Off-Heap Persistence for Large Datasets
In scenarios where large datasets need to be stored persistently, leveraging off-heap persistence mechanisms such as Apache Chronicle Map can be beneficial. Off-heap memory storage can reduce the impact on the garbage collector and allow for efficient memory usage, especially for very large collections.
// Initializing an off-heap persisted collection with Apache Chronicle Map
ChronicleMap<Integer, String> persistedMap = ChronicleMap
.of(Integer.class, String.class)
.averageKeySize(4)
.entries(1000)
.createPersistedTo(new File("persistedMap.dat"));
By utilizing off-heap persistence for large datasets, the impact on the garbage collector can be minimized, leading to optimized memory usage and improved application performance.
Profile and Analyze Memory Usage
Profiling and analyzing memory usage using tools like Java VisualVM and YourKit Java Profiler can provide valuable insights into the memory footprint of Java collections within your application. By identifying memory hotspots and inefficient memory usage patterns, targeted optimizations can be applied to improve memory efficiency.
In conclusion, optimizing memory usage in Java collections is essential for building high-performance applications. By choosing the right collection types, providing appropriate initial capacities, minimizing auto-boxing, choosing immutable collections judiciously, avoiding unnecessary object creation, leveraging off-heap persistence for large datasets, and profiling memory usage, you can effectively optimize memory usage in Java collections and enhance the overall performance of your Java applications.
Remember, efficient memory usage not only leads to better performance but also contributes to a more sustainable and resource-efficient application environment.