Improving ActiveMQ Persistent Messaging Performance
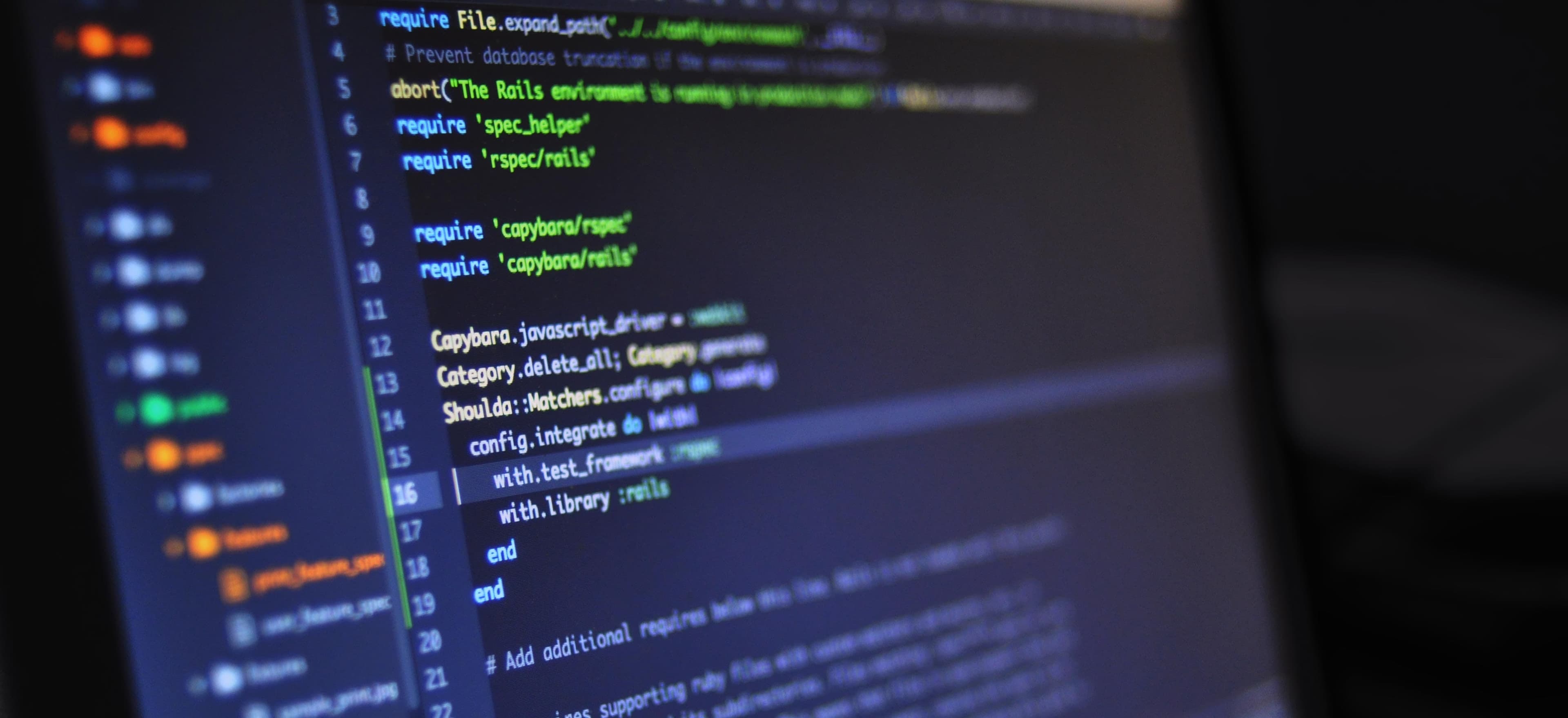
- Published on
Improving ActiveMQ Persistent Messaging Performance
ActiveMQ is a popular open-source message broker that provides robust messaging capabilities for applications. When dealing with persistent messaging, it's crucial to optimize performance to ensure efficient message persistence and retrieval. In this blog post, we'll explore some strategies and best practices for improving ActiveMQ persistent messaging performance using Java.
Understanding Persistent Messaging
First, let's grasp the concept of persistent messaging in ActiveMQ. When a message is sent to a queue or topic, it can be delivered in two modes: persistent and non-persistent. Persistent messages are stored in the broker's underlying storage (usually a database or file system) to ensure they are not lost even if the broker crashes. On the other hand, non-persistent messages are not stored, making them faster but riskier in case of a failure.
Choosing the Right Broker Configuration
The performance of persistent messaging in ActiveMQ heavily depends on the broker's configuration. One crucial configuration is the persistence adapter, which determines how messages are stored. ActiveMQ supports various persistence adapters such as JDBC, KahaDB, and LevelDB. Each adapter has its strengths and weaknesses in terms of performance and reliability.
For instance, the default persistence adapter, KahaDB, offers a good balance between performance and reliability. It's optimized for fast message persistence and retrieval, making it suitable for most use cases. On the other hand, the JDBC persistence adapter provides flexibility by allowing the use of different databases but may introduce additional overhead due to database interactions.
When optimizing performance, it's essential to choose the right persistence adapter based on your specific requirements and workload characteristics. It's also crucial to tune the adapter's configuration parameters such as journal file size, checkpoint interval, and index directory to align with your application's needs.
Efficient Message Production
In a messaging system, message producers play a significant role in determining overall performance. When sending persistent messages, producers should aim for efficient message production to minimize overhead and optimize throughput.
Here's a Java code snippet demonstrating efficient message production in ActiveMQ using the JMS API:
// Create a connection factory
ConnectionFactory factory = new ActiveMQConnectionFactory("tcp://localhost:61616");
// Create a connection
Connection connection = factory.createConnection();
connection.start();
// Create a session
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
// Create a destination
Destination destination = session.createQueue("example.queue");
// Create a producer
MessageProducer producer = session.createProducer(destination);
// Enable message persistence
producer.setDeliveryMode(DeliveryMode.PERSISTENT);
// Create a message
TextMessage message = session.createTextMessage("This is a persistent message");
// Send the message
producer.send(message);
// Clean up resources
session.close();
connection.close();
In this code snippet, we create a persistent message producer that sets the delivery mode to PERSISTENT
, ensuring that the message is persisted by the broker. It's crucial to minimize unnecessary object creation and reuse resources such as connections and sessions to improve performance.
Optimizing Consumer Configuration
Efficient message consumption is equally important for achieving optimal performance in ActiveMQ. When consuming persistent messages, consumers should be configured to handle messages efficiently without introducing unnecessary processing overhead.
Consider the following Java code snippet illustrating an optimized message consumer in ActiveMQ using the JMS API:
// Create a connection factory
ConnectionFactory factory = new ActiveMQConnectionFactory("tcp://localhost:61616");
// Create a connection
Connection connection = factory.createConnection();
connection.start();
// Create a session
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
// Create a destination
Destination destination = session.createQueue("example.queue");
// Create a consumer
MessageConsumer consumer = session.createConsumer(destination);
// Implement the message listener
consumer.setMessageListener(message -> {
if (message instanceof TextMessage) {
try {
String text = ((TextMessage) message).getText();
// Process the message
System.out.println("Received persistent message: " + text);
} catch (JMSException e) {
e.printStackTrace();
}
}
});
// Clean up resources (Note: The connection and session should be kept open for message consumption)
In this code snippet, we create a message consumer using a message listener, which asynchronously processes incoming messages. Asynchronous message processing can significantly improve throughput and reduce latency, especially in high-volume scenarios. Additionally, it's crucial to handle message acknowledgment and error handling appropriately to ensure reliable message processing.
Utilizing Batch Message Operations
When dealing with high-throughput persistent messaging, leveraging batch message operations can greatly enhance performance. ActiveMQ provides support for batch message operations, allowing producers and consumers to send and receive messages in batches rather than individually.
Let's take a look at a Java code snippet demonstrating batch message operations for improved performance:
// Create a connection factory
ConnectionFactory factory = new ActiveMQConnectionFactory("tcp://localhost:61616");
// Create a connection
Connection connection = factory.createConnection();
connection.start();
// Create a session with transacted mode and auto acknowledgment
Session session = connection.createSession(true, Session.AUTO_ACKNOWLEDGE);
// Create a destination
Destination destination = session.createQueue("example.queue");
// Create a producer
MessageProducer producer = session.createProducer(destination);
// Enable message persistence
producer.setDeliveryMode(DeliveryMode.PERSISTENT);
// Create multiple messages
List<Message> messages = new ArrayList<>();
for (int i = 0; i < 100; i++) {
TextMessage message = session.createTextMessage("Batch message " + i);
messages.add(message);
}
// Send the messages in a batch
for (Message message : messages) {
producer.send(message);
}
// Commit the session to send the messages
session.commit();
// Clean up resources
session.close();
connection.close();
In this code snippet, we create a batch message producer that accumulates multiple messages in a list and sends them in a single batch. Batch message operations can significantly reduce the overhead associated with individual message sends, leading to improved throughput and reduced latency.
Closing the Chapter
In conclusion, optimizing the performance of persistent messaging in ActiveMQ is crucial for ensuring efficient message persistence and retrieval in Java applications. By carefully configuring the broker, implementing efficient message production and consumption, and leveraging batch message operations, developers can achieve significant performance improvements.
When working with ActiveMQ, it's essential to consider the specific requirements and characteristics of your application's workload and tailor the performance optimization strategies accordingly. By following the best practices outlined in this blog post, developers can enhance the performance of their ActiveMQ persistent messaging infrastructure and deliver a seamless messaging experience for their applications.
For more in-depth information on ActiveMQ, you can refer to the ActiveMQ documentation and explore additional performance optimization techniques to further enhance your messaging infrastructure.
Remember, performance optimization is an ongoing process, and it's essential to continuously monitor and fine-tune your ActiveMQ setup to adapt to evolving workloads and usage patterns.
Happy messaging!