Optimizing Event Invitations for Seam Integration
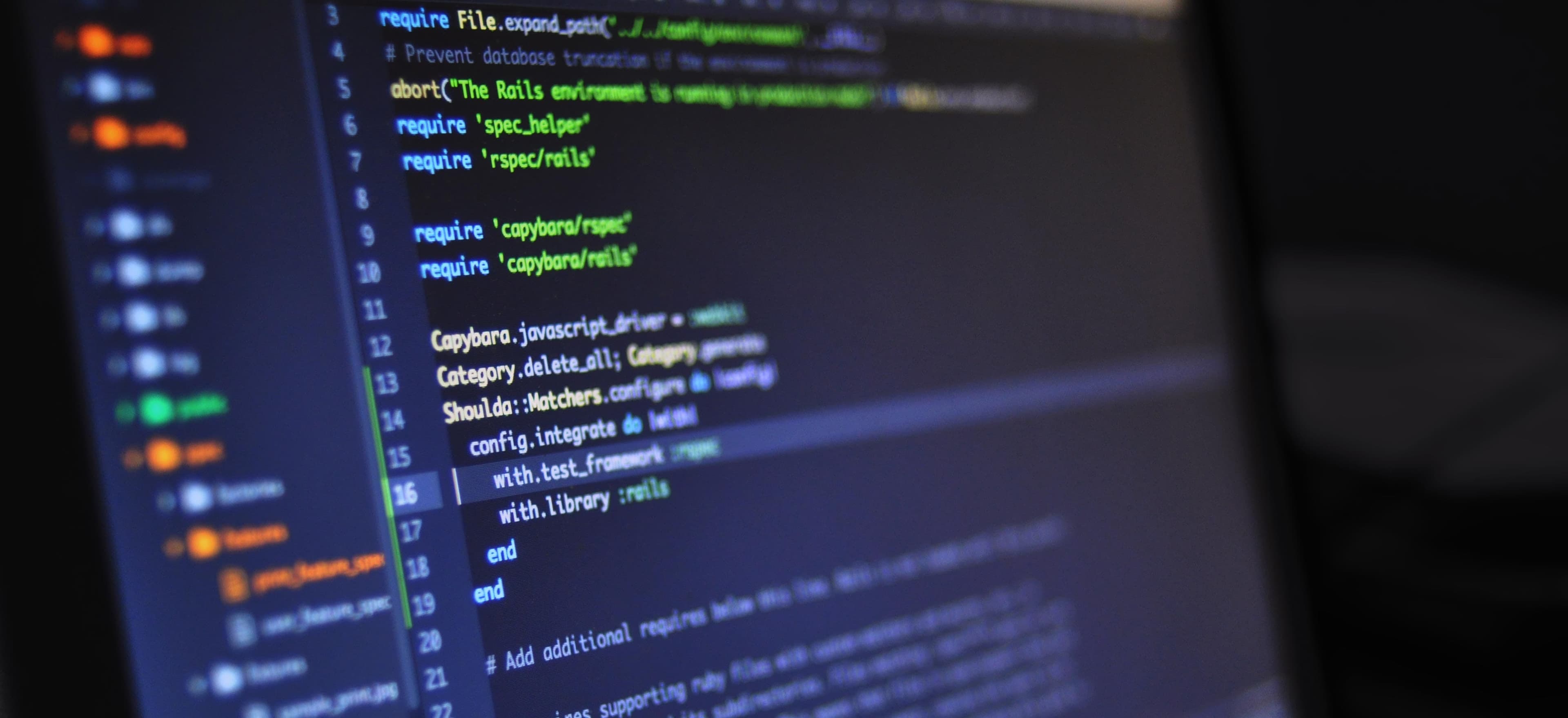
- Published on
Optimizing Event Invitations for Seam Integration
In the world of Java development, Seam Integration plays a vital role in connecting different components of an application to optimize its performance. When it comes to handling event invitations within a Java application, optimizing the process through Seam Integration can significantly enhance the overall efficiency and user experience. In this article, we will delve into the best practices for optimizing event invitations using Seam Integration in Java.
Understanding Event Invitations
Before we delve into the optimization techniques, let's take a moment to understand what event invitations entail within the context of Java development. In a typical Java application, events play a crucial role in triggering actions or notifications based on specific occurrences or user interactions. Event invitations, in this context, refer to the process of notifying and inviting users or systems to partake in or respond to certain events or actions within the application.
Seam Integration for Event Invitations
Seam Integration, a powerful framework for Java EE applications, provides an efficient way to manage and integrate various components within the application. When it comes to handling event invitations, Seam Integration offers a seamless approach to streamline the process, ensuring optimal performance and reliability.
Optimizing Event Invitations with Seam Integration
Now, let's explore some best practices for optimizing event invitations using Seam Integration in Java.
1. Leveraging Asynchronous Event Processing
In scenarios where event invitations result in time-consuming processes or require external interactions, leveraging asynchronous event processing can significantly enhance the responsiveness of the application. By offloading time-intensive tasks to background processes, the application can continue to function without delays, providing a smoother user experience.
@Asynchronous
public void processEventInvitation(Event event) {
// Perform time-consuming tasks here
}
The @Asynchronous
annotation in Seam Integration allows the designated method to be executed asynchronously, preventing the main thread from being blocked by lengthy operations. This optimization ensures that event invitations do not hinder the overall responsiveness of the application.
2. Implementing Event Queues for Scalability
In scenarios where the application deals with a high volume of event invitations, implementing event queues can optimize the processing and management of these invitations. Seam Integration provides robust support for implementing event queues, allowing for efficient handling of concurrent event invitations and ensuring scalability.
@In
private EventQueue eventQueue;
public void sendEventInvitation(Event event) {
eventQueue.publish(event);
}
By publishing event invitations to a dedicated event queue, the application can handle multiple invitations concurrently without overwhelming the system. This optimization contributes to the scalability and performance of event processing within the application.
3. Utilizing CDI Event Observers
Contexts and Dependency Injection (CDI) in Java EE offers a powerful mechanism for observing and responding to events within the application. By utilizing CDI event observers, the application can optimize the handling of event invitations by decoupling the event generation and processing, leading to more streamlined and maintainable code.
public void onEventInvitationReceived(@Observes Event event) {
// Process the event invitation
}
The @Observes
annotation in CDI allows a method to observe and react to a specific event, enabling efficient and modularized handling of event invitations. This optimization promotes code reusability and simplifies the integration of event processing logic.
4. Applying Event Filters for Precision
In certain scenarios, it is essential to apply filters to event invitations to ensure that only relevant components or systems respond to specific events. Seam Integration enables the application to apply event filters, allowing for precise targeting of event invitations based on specified criteria.
@Inject
private Event<EventPayload> event;
public void sendFilteredEventInvitation(EventPayload payload) {
event.select(new AnnotationLiteral<QualifiedEvent>() {}).fire(payload);
}
By utilizing event filters, the application can optimize the handling of event invitations, ensuring that only designated components or systems respond to events that meet the defined criteria. This optimization enhances the accuracy and relevance of event processing within the application.
To Wrap Things Up
Optimizing event invitations through Seam Integration plays a pivotal role in enhancing the efficiency, scalability, and reliability of event processing within Java applications. By leveraging asynchronous processing, implementing event queues, utilizing CDI event observers, and applying event filters, developers can ensure that event invitations are handled seamlessly and effectively. Incorporating these best practices not only enhances the performance of the application but also contributes to an improved overall user experience.
In conclusion, Seam Integration offers a robust framework for optimizing event invitations in Java applications, empowering developers to streamline event processing and maximize the potential of their applications.
For further insights into Seam Integration and Java EE applications, Oracle's official documentation and Red Hat's developer resources can provide valuable information and guidance.
Remember, optimizing event invitations isn't just about performance – it's about creating a seamless and responsive experience for the end-users.