Effective Strategies for Database Testing
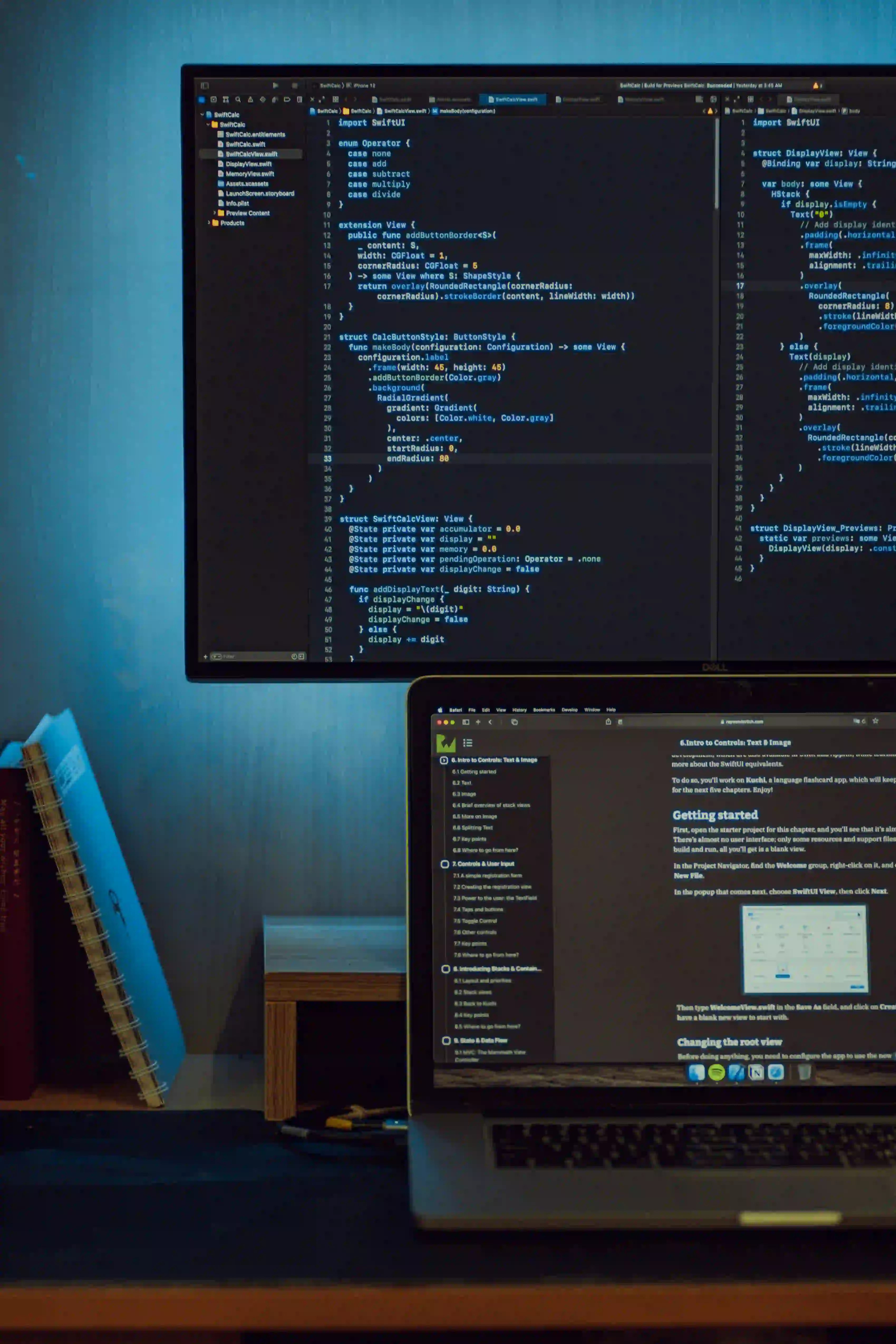
Effective Strategies for Database Testing
When it comes to developing robust software applications, database testing plays a crucial role. It ensures that the data storage and retrieval processes function seamlessly, thereby enhancing the overall application performance. In this blog post, we will delve into the significance of database testing, explore effective strategies, and discuss best practices for implementing database testing in Java applications.
Understanding the Importance of Database Testing
Database testing validates the integrity and reliability of data stored in databases. It ensures that the data manipulation operations such as insert, update, and delete work as expected and do not compromise the consistency of the database. By performing database testing, developers can identify anomalies, optimize queries, and enhance the overall efficiency of database operations.
Strategies for Effective Database Testing in Java
1. Use Mocking Frameworks
Mocking frameworks such as Mockito and PowerMockito are invaluable for simulating database interactions during testing. They allow developers to create mock objects that mimic the behavior of real database components, enabling comprehensive testing without actually accessing the database. This approach enhances test efficiency and reduces the dependency on the actual database during the development phase.
// Example of using Mockito for mocking database interaction
@Test
public void testDatabaseInteraction() {
// Create a mock database connection
DatabaseConnection mockConnection = Mockito.mock(DatabaseConnection.class);
// Define mock behavior for database interaction
Mockito.when(mockConnection.executeQuery("SELECT * FROM users"))
.thenReturn(mockResultSet);
// Invoke the method that interacts with the database
// and assert the expected outcome
...
}
2. Implement Integration Testing
Integration testing involves validating the interactions between the application and the database. In Java applications, tools like JUnit and TestNG can be leveraged to perform integration testing by executing test cases that cover database connectivity, data retrieval, and transaction management. Integration testing helps identify issues related to data access, query execution, and overall database functionality within the application ecosystem.
// Example of integration testing using JUnit
@Test
public void testDatabaseIntegration() {
// Set up test data and execute database operations
...
// Validate the outcome and assert expected results
...
}
3. Leverage In-Memory Databases
In-Memory databases such as H2 and HSQLDB provide lightweight, efficient alternatives to traditional database systems for testing purposes. These databases can be instantiated within the testing environment, allowing for rapid execution of test cases without the overhead of external dependencies. In Java, frameworks like Spring Boot enable seamless integration of in-memory databases for comprehensive testing of data access and manipulation logic.
// Example of using H2 in-memory database for testing
@Test
public void testUsingH2Database() {
// Initialize an H2 in-memory database
...
// Execute test cases involving database operations
...
}
4. Employ Data-Driven Testing
Data-driven testing involves testing scenarios using a variety of input data sets to validate the behavior of database operations. In Java, frameworks like TestNG and JUnit support parameterized tests, enabling developers to execute test cases with different input values. By incorporating data-driven testing, developers can ensure that their database operations are resilient to diverse data sets and edge cases.
// Example of data-driven testing with JUnit and TestNG
@ParameterizedTest
@CsvSource({ "1, 'John'", "2, 'Doe'", "3, 'Smith'" })
public void testDatabaseOperations(int userId, String userName) {
// Execute database operations using the input data
...
// Verify the expected outcome for each data set
...
}
5. Monitor and Optimize Query Performance
Database testing should encompass performance evaluation to identify inefficient queries and bottlenecks. Tools like Hibernate Profiler and JProfiler provide insights into query execution times, resource utilization, and overall database performance. By analyzing query execution plans and optimizing slow queries, developers can enhance the overall responsiveness and scalability of their database-driven Java applications.
Best Practices for Successful Database Testing
-
Isolate Test Environment: Ensure that the testing environment is separate from the production environment to prevent unintended data modifications or corruption.
-
Automate Testing Processes: Leverage automation tools such as Selenium, Cucumber, or Apache JMeter to streamline database testing and integrate it into the continuous integration/continuous deployment (CI/CD) pipeline.
-
Use Test Data Generators: Generate diverse and realistic test data to cover a wide range of scenarios, ensuring comprehensive test coverage and uncovering potential edge cases.
-
Secure Sensitive Data: When using real data for testing, obfuscate or encrypt sensitive information to adhere to data privacy regulations and best security practices.
-
Version Control Test Data: Maintain versioned test data sets to facilitate reproducibility and traceability of test results across different iterations of the application.
Lessons Learned
Database testing is a critical aspect of ensuring the reliability and performance of Java applications that rely on data storage and retrieval. By employing effective strategies such as mocking frameworks, integration testing, in-memory databases, data-driven testing, and query performance optimization, developers can bolster the robustness of their database operations. Embracing best practices and leveraging automation tools further contributes to the successful implementation of database testing in Java applications.
In the dynamic landscape of software development, the role of database testing in maintaining data integrity and application resilience cannot be overstated. As technology continues to evolve, embracing a comprehensive approach to database testing will be paramount in delivering high-quality, scalable Java applications.
References: