Optimizing MongoDB Queries for Better Performance
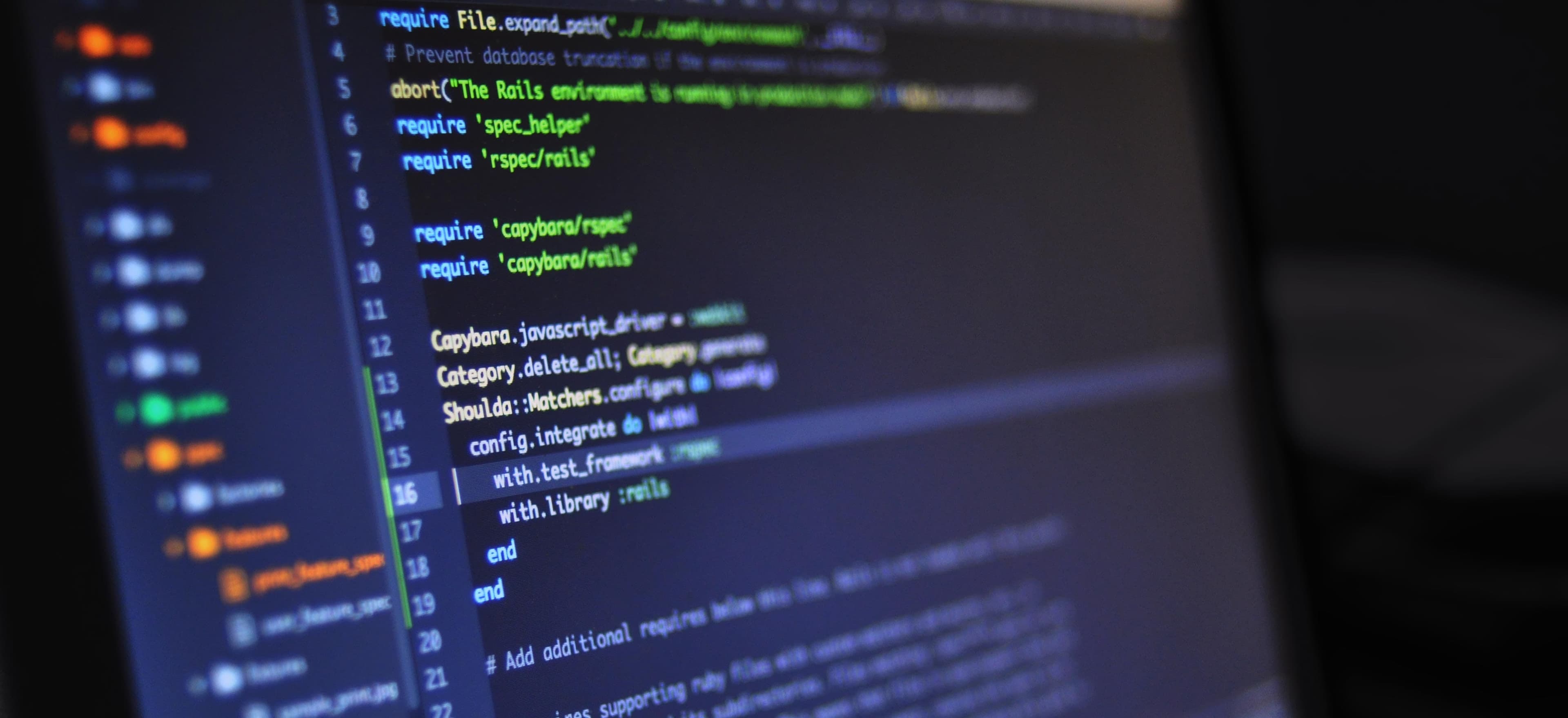
- Published on
Optimizing MongoDB Queries for Better Performance
When it comes to building efficient and high-performing applications, optimizing database queries is paramount. MongoDB is a popular NoSQL database that offers flexibility and scalability, but writing efficient queries is crucial for achieving optimal performance. In this article, we'll delve into various strategies and best practices for optimizing MongoDB queries to boost the overall performance of your applications.
Understanding Query Performance
Before diving into optimization techniques, it's essential to understand the key factors that influence query performance in MongoDB. Some of the primary factors include:
-
Indexes: Indexes play a crucial role in query performance. They allow MongoDB to locate documents quickly, significantly speeding up query execution.
-
Query Structure: The way queries are structured can have a significant impact on performance. Understanding how MongoDB processes and executes queries is essential for writing efficient ones.
-
Data Model: The schema design and data model have implications on query performance. A well-designed data model can lead to better query performance.
Now that we have a clear understanding of the factors that affect query performance, let's explore some optimization techniques.
Indexing Strategies
Create Indexes Based on Query Patterns
One of the most effective ways to optimize MongoDB queries is by creating indexes based on the query patterns of your application. By analyzing the most frequent and performance-sensitive queries, you can create indexes that directly address the fields and patterns used in those queries.
db.collection.createIndex({ field: 1 });
Why: Indexes improve query performance by allowing MongoDB to swiftly identify the documents that match the query conditions. The choice of index type (single field, compound, multi-key, etc.) depends on the specific query patterns and the nature of the data.
Analyze Query Execution Plans
MongoDB provides a explain
method that allows you to view the query execution plans. This can provide valuable insights into how MongoDB executes queries and help identify areas for optimization.
db.collection.find({ field: value }).explain("executionStats");
Why: Understanding the query execution plan helps in identifying whether an index is being used efficiently, the number of documents scanned, and the overall query performance. This information is crucial for optimizing queries.
Query Structure and Optimization
Utilize Query Projection
When querying data, it's common not to need all fields from the documents. Utilizing query projection allows you to retrieve only the necessary fields, minimizing the amount of data transferred and improving query performance.
db.collection.find({ field: value }, { field1: 1, field2: 1 });
Why: By retrieving only the required fields, you reduce the amount of data that needs to be transferred over the network, thus improving query performance.
Avoiding Large Result Sets
Fetching a large number of documents can severely affect query performance. It's crucial to limit the result set by using pagination, which retrieves documents in smaller, manageable chunks.
db.collection.find({}).skip(20).limit(10);
Why: By paginating the query results, you avoid overloading the network and the application with a large volume of data, ultimately enhancing query performance.
Data Modeling Best Practices
Embedded Data Models for Performance
In MongoDB, embedded data models can often outperform normalized models for certain use cases. By embedding related data within a single document, you can retrieve the entire dataset with a single query, leading to significant performance improvements.
{
_id: "123",
title: "Sample Post",
author: {
name: "John Doe",
email: "john@example.com"
}
}
Why: Embedded data models eliminate the need for costly joins and reduce the number of queries required to fetch related data, thus enhancing performance.
Use Aggregation Pipeline for Complex Data Manipulation
The aggregation pipeline in MongoDB is a powerful tool for manipulating and processing data within the database. It allows for complex transformations and computations to be performed server-side, reducing the amount of data transferred over the network and improving overall performance.
db.collection.aggregate([
{ $match: { status: "active" } },
{ $group: { _id: "$category", total: { $sum: "$quantity" } } }
]);
Why: By utilizing the aggregation framework, you can perform complex data manipulations and computations within the database, thereby reducing the amount of data that needs to be transferred to the application for processing.
A Final Look
Optimizing MongoDB queries is essential for achieving optimal performance in your applications. By utilizing effective indexing strategies, optimizing query structure, and employing best practices in data modeling, you can significantly enhance the overall performance of your MongoDB-powered applications. Understanding the nuances of MongoDB query optimization empowers developers to build robust and high-performing systems.
In conclusion, by incorporating these optimization techniques and best practices, you can ensure that your MongoDB queries are efficient, and your applications perform at their best.
Remember, query performance is not a one-time task. Regular monitoring, analysis, and fine-tuning are key to maintaining optimal query performance as data volumes and application usage evolve over time.
So, apply these strategies, keep monitoring, and keep optimizing for the best performance of your MongoDB queries.