Maximizing Productivity in Mobile App Development
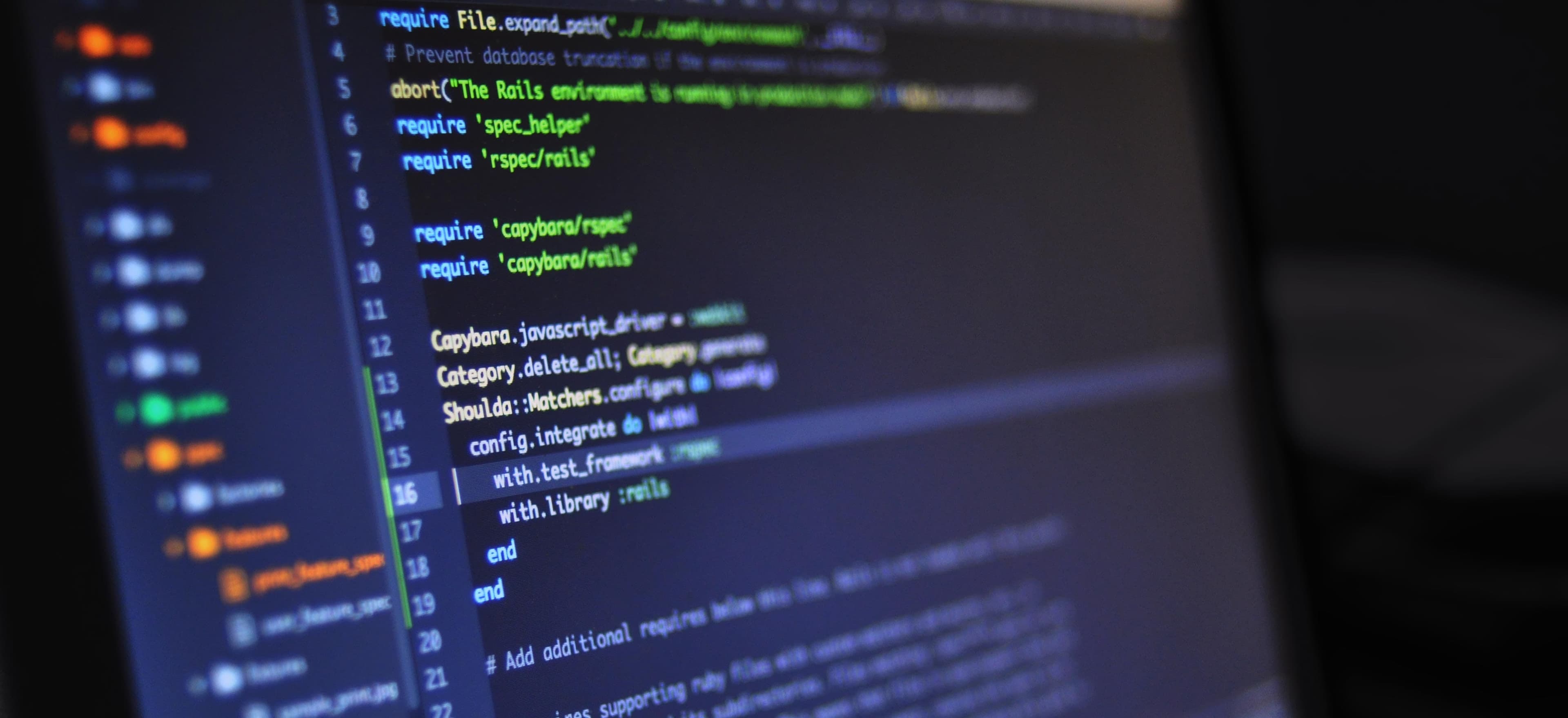
- Published on
Maximizing Productivity in Mobile App Development
Mobile app development has become an essential aspect of modern business, and Java remains a popular language of choice for Android app development. With its versatility and robustness, Java empowers developers to build efficient and powerful mobile applications. In this blog post, we will explore various techniques and best practices for maximizing productivity in Java-based mobile app development.
Embracing Modularization with Java 9 and Later
Java 9 introduced a module system that allows developers to better organize their code and dependencies. By modularizing your mobile app, you can improve maintainability, reusability, and collaboration among team members. Let's take a look at how modularization can be implemented in a mobile app project.
// module-info.java
module com.example.myapp {
requires java.base;
requires transitive java.sql;
exports com.example.myapp.core;
}
In the above code snippet, we define a module-info.java file that specifies the dependencies and exports of our app module. By breaking down the app into smaller, manageable modules, developers can work on isolated components, leading to increased productivity and code quality.
By embracing modularization, mobile app developers can also take advantage of improved performance and security, as well as better support for larger codebases.
Leveraging Dependency Injection for Decoupling
Dependency injection is a powerful design pattern that promotes decoupling and testability in Java applications. When building mobile apps, leveraging dependency injection frameworks such as Dagger 2 can significantly improve productivity and code maintainability.
// Using Dagger 2 for dependency injection
@Component
public interface CarComponent {
Car getCar();
}
// Usage
CarComponent component = DaggerCarComponent.create();
Car car = component.getCar();
car.drive();
In the above code snippet, we define a CarComponent interface using Dagger 2, which handles the dependency injection of the Car object. By decoupling the creation and usage of dependencies, developers can focus on building individual app components without being tightly coupled to their dependencies.
Embracing Reactive Programming with RxJava
Reactive programming has gained popularity in the mobile app development space due to its ability to handle asynchronous events and data streams. RxJava, a widely used library for reactive programming in Java, allows developers to write asynchronous, event-based code in a more concise and readable manner.
// Using RxJava for asynchronous operations
Observable.fromCallable(() -> fetchDataFromNetwork())
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(data -> handleData(data));
In the above code snippet, we use RxJava to perform asynchronous network operations and observe the results on the main thread. By embracing reactive programming, developers can write more responsive and scalable mobile apps, leading to improved productivity and user experience.
Automated Testing for Quality Assurance
Testing is a critical aspect of mobile app development, and automated testing frameworks such as JUnit and Espresso can greatly enhance productivity by enabling developers to quickly validate app behavior and performance.
// Unit testing with JUnit
@Test
public void addition_isCorrect() {
assertEquals(4, 2 + 2);
}
// UI testing with Espresso
@Test
public void validateLoginButton() {
onView(withId(R.id.login_button)).check(matches(isDisplayed()));
}
In the above code snippets, we demonstrate a simple unit test using JUnit and a UI test using Espresso for Android. By incorporating automated testing into the development workflow, developers can catch bugs early, ensure code reliability, and iterate on app features with confidence.
Leveraging IDE Features and Plugins
Modern Integrated Development Environments (IDEs) such as IntelliJ IDEA and Android Studio offer a wide range of features and plugins that can significantly boost developer productivity. Leveraging code refactoring tools, debugging utilities, and version control integration can streamline the app development process.
Additionally, plugins such as Lombok for Java can automate repetitive code boilerplate, while SonarLint can help identify and fix code quality issues early in the development cycle.
Final Thoughts
In conclusion, maximizing productivity in Java-based mobile app development requires a combination of best practices, tools, and mindset. Embracing modularization, leveraging dependency injection, adopting reactive programming, prioritizing automated testing, and making the most of IDE features and plugins are all essential aspects of building high-quality, efficient mobile applications.
By incorporating these techniques and best practices into your mobile app development workflow, you can streamline development processes, improve code quality, and deliver exceptional user experiences.
Remember, productivity is not just about writing code quickly, but also about writing maintainable, reliable, and scalable code that stands the test of time. It's about building a solid foundation for your mobile app that allows for future growth and innovation.
So, embrace the best practices, use the right tools, and stay informed about the latest trends and advancements in the mobile app development space. This holistic approach will undoubtedly set you on the path to maximizing productivity and success in your Java-based mobile app development endeavors.
And as always, happy coding!