Demystifying Server-Side Tracking for Enhanced Analytics
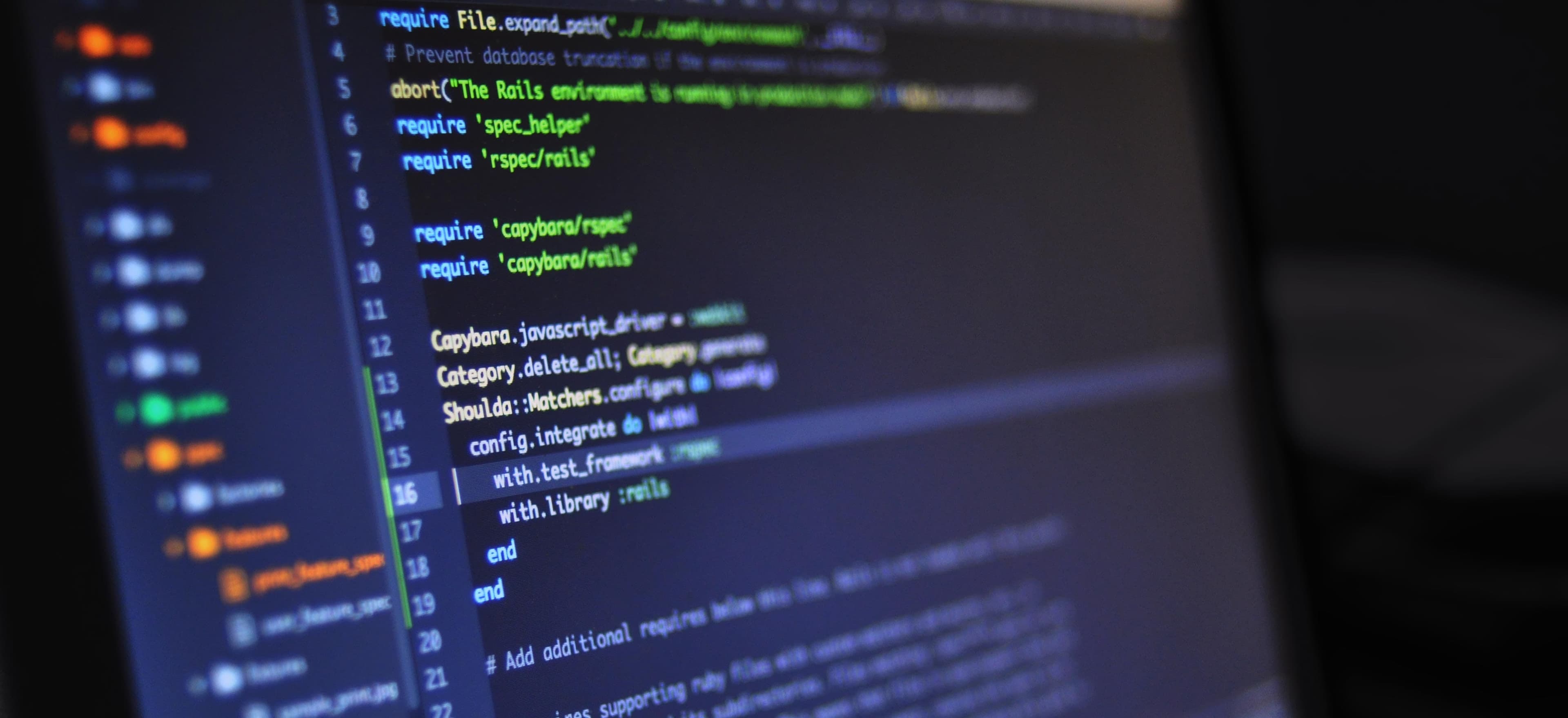
- Published on
Demystifying Server-Side Tracking for Enhanced Analytics
In the realm of web development, server-side tracking is an indispensable tool for collecting valuable data and insights. By leveraging Java for server-side tracking, developers can gain a deeper understanding of user behavior, optimize performance, and enhance the overall user experience.
In this article, we will explore the fundamentals of server-side tracking, delve into the benefits of using Java for this purpose, and provide a comprehensive guide to implementing server-side tracking in Java for enhanced analytics.
Understanding Server-Side Tracking
Server-side tracking involves capturing and analyzing user interactions with a website or application directly on the server. This approach offers several advantages over client-side tracking, including improved data accuracy, enhanced privacy compliance, and the ability to track interactions that may not be captured through client-side methods.
By implementing server-side tracking, developers can gather comprehensive data about user behavior, such as page views, button clicks, form submissions, and other custom events. This data serves as a foundation for in-depth analytics, enabling businesses to make informed decisions and optimize their digital properties.
The Power of Java for Server-Side Tracking
Java's robust and versatile nature makes it an ideal choice for server-side tracking. With its strong emphasis on performance, scalability, and reliability, Java provides a solid foundation for implementing tracking mechanisms that can handle large volumes of data with ease.
Additionally, Java's rich ecosystem of libraries and frameworks, such as Spring and Apache Kafka, simplifies the process of integrating server-side tracking into existing web applications. These tools offer comprehensive support for data processing, storage, and analysis, empowering developers to build sophisticated tracking systems with minimal effort.
Implementing Server-Side Tracking in Java
Now, let's dive into the practical aspects of implementing server-side tracking in Java. We'll walk through the key steps involved in setting up a basic tracking system using Java and demonstrate how to capture and process user interactions for enhanced analytics.
Step 1: Setting Up the Tracking Infrastructure
The first step is to establish the necessary infrastructure for tracking user interactions. This involves configuring the data storage, defining the tracking schema, and integrating any required dependencies, such as database connectors and messaging queues.
// Example: Setting up a database connection pool using HikariCP
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/tracking_db");
config.setUsername("username");
config.setPassword("password");
HikariDataSource dataSource = new HikariDataSource(config);
In this example, we use HikariCP to create a connection pool for interacting with a MySQL database, which will serve as the storage backend for our tracking data.
Step 2: Capturing User Interactions
Once the infrastructure is in place, we can start capturing user interactions within our application. This typically involves instrumenting the code to record relevant events and attributes, such as timestamps, user identifiers, and event types.
// Example: Capturing a page view event
public void trackPageView(String userId, String pageName, long timestamp) {
// Store the event data in the database
try (Connection connection = dataSource.getConnection()) {
String insertQuery = "INSERT INTO page_views (user_id, page_name, timestamp) VALUES (?, ?, ?)";
try (PreparedStatement statement = connection.prepareStatement(insertQuery)) {
statement.setString(1, userId);
statement.setString(2, pageName);
statement.setLong(3, timestamp);
statement.executeUpdate();
}
} catch (SQLException e) {
// Handle the exception
}
}
In this example, we define a method for tracking page views, which inserts the relevant data into the database for later analysis.
Step 3: Processing and Analyzing Tracking Data
With the user interactions being captured, we can now focus on processing and analyzing the tracking data to derive meaningful insights. This may involve running periodic batch jobs, leveraging real-time stream processing, or utilizing analytics tools to gain actionable intelligence from the collected data.
// Example: Performing analysis on page view data
public void analyzePageViews() {
// Query the database for page view statistics
try (Connection connection = dataSource.getConnection()) {
String query = "SELECT page_name, COUNT(*) AS view_count FROM page_views GROUP BY page_name";
try (PreparedStatement statement = connection.prepareStatement(query)) {
try (ResultSet resultSet = statement.executeQuery()) {
while (resultSet.next()) {
String pageName = resultSet.getString("page_name");
int viewCount = resultSet.getInt("view_count");
// Process the statistics
}
}
}
} catch (SQLException e) {
// Handle the exception
}
}
In this example, we demonstrate how to analyze page view data by querying the database and processing the results to derive valuable statistics.
To Wrap Things Up
In conclusion, server-side tracking in Java offers a powerful mechanism for gathering and analyzing user interactions, enabling businesses to gain valuable insights and optimize their digital experiences. By leveraging Java's strengths in performance, scalability, and ecosystem support, developers can implement sophisticated tracking systems that drive data-driven decision-making and foster continuous improvement.
Incorporating server-side tracking into web applications is essential for obtaining a comprehensive view of user behavior and performance metrics. With the right tools and practices in place, businesses can harness the full potential of server-side tracking to drive their success in the digital landscape.
By embracing server-side tracking in Java, developers and organizations can unlock a wealth of actionable insights that fuel informed decision-making and drive business growth.
For further reading on server-side tracking and Java development, consider exploring the Spring Framework and Apache Kafka for comprehensive support in building robust tracking systems.