Scalable Multi-Core Counter Implementation
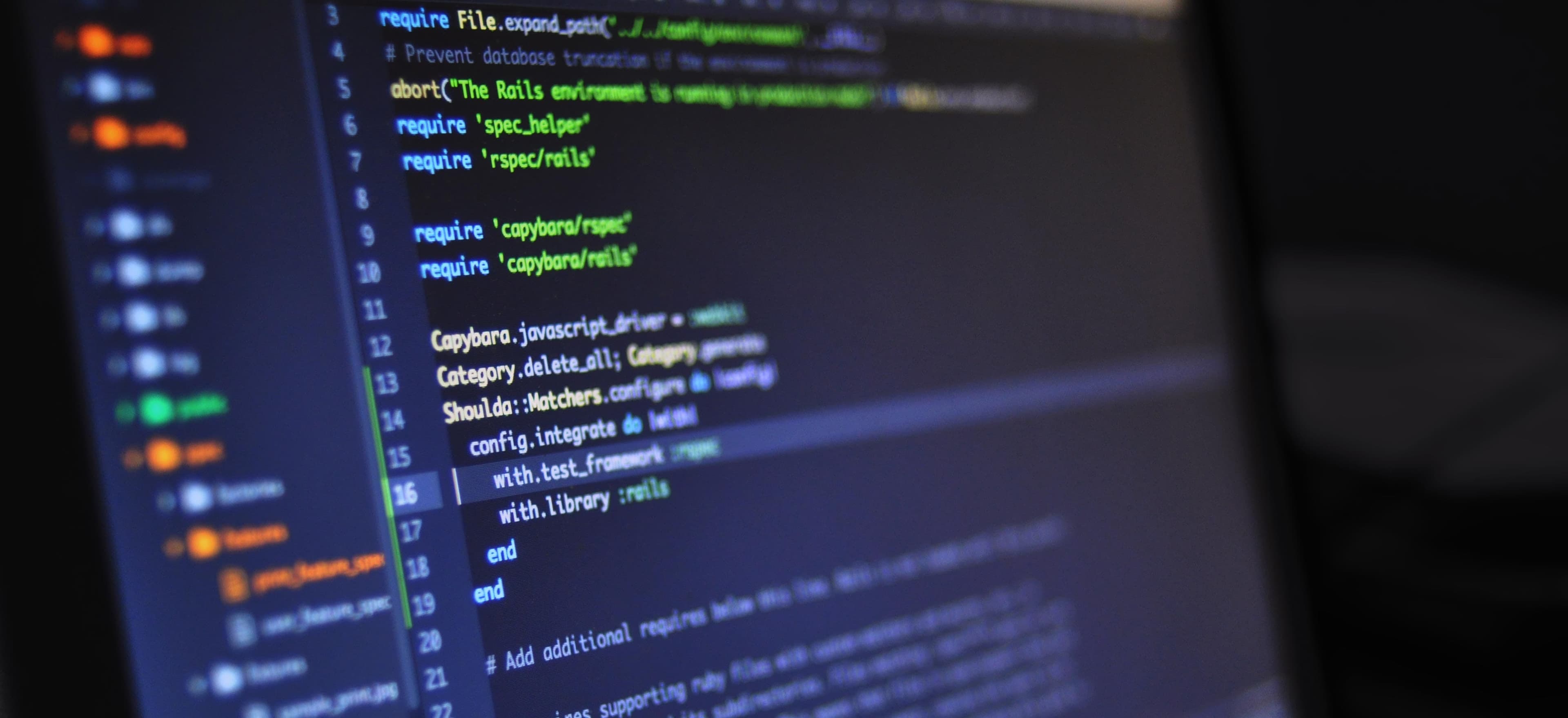
- Published on
Building a Scalable Multi-Core Counter Implementation in Java
In today's computing landscape, multi-core processors have become the norm. To fully leverage this hardware, it's important to design applications that can efficiently distribute work across multiple cores. In this blog post, we'll explore how to implement a scalable multi-core counter in Java, allowing for efficient concurrent operations while maintaining accuracy and performance.
The Problem Statement
Imagine a scenario where multiple threads need to update a shared counter variable concurrently. Without proper synchronization, this could lead to race conditions and data inconsistencies. Traditional synchronization mechanisms like synchronized
blocks or the synchronized
keyword can introduce contention and limit scalability, especially in scenarios with a high degree of parallelism.
To address this challenge, we'll explore a more scalable approach using the LongAdder
class from Java's java.util.concurrent.atomic
package.
Introducing LongAdder
The LongAdder
class is designed to provide a high-throughput, low-contention alternative to traditional synchronization techniques for managing a single numerical value across multiple threads. It achieves this by partitioning the underlying value into cells, with each cell managing its own partial sum. When multiple threads concurrently update the LongAdder
, each thread operates on a different cell, reducing contention and allowing for greater concurrency.
Implementation
Let's delve into the code and see how we can implement a scalable multi-core counter using LongAdder
.
import java.util.concurrent.atomic.LongAdder;
public class MultiCoreCounter {
private LongAdder count = new LongAdder();
public void increment() {
count.increment();
}
public void decrement() {
count.decrement();
}
public long getCount() {
return count.sum();
}
}
In the above code, we've created a MultiCoreCounter
class that encapsulates a LongAdder
instance to manage the counter. The increment()
and decrement()
methods simply delegate the operations to the underlying LongAdder
, which takes care of the concurrent updates efficiently. The getCount()
method provides a way to retrieve the current count.
Understanding the Implementation
Why LongAdder?
The use of LongAdder
offers several advantages over traditional synchronization techniques like synchronized
blocks or the volatile
keyword. By internally partitioning the counter value, LongAdder
minimizes contention, making it well-suited for scenarios with high levels of concurrent updates. This makes it a scalable choice for applications running on multi-core systems.
Leveraging the Sum Operation
In the getCount()
method, we use the sum()
operation provided by LongAdder
to calculate the current count. This operation efficiently aggregates the partial sums maintained by each cell, providing an accurate and up-to-date result without introducing contention.
Testing the Scalable Multi-Core Counter
Let's write a simple test to validate the concurrent behavior and scalability of our MultiCoreCounter
implementation.
public class MultiCoreCounterTest {
public static void main(String[] args) throws InterruptedException {
MultiCoreCounter counter = new MultiCoreCounter();
int numThreads = 100;
int numIncrements = 10000;
List<Thread> threads = new ArrayList<>();
for (int i = 0; i < numThreads; i++) {
Thread thread = new Thread(() -> {
for (int j = 0; j < numIncrements; j++) {
counter.increment();
}
});
threads.add(thread);
thread.start();
}
for (Thread thread : threads) {
thread.join();
}
System.out.println("Final Count: " + counter.getCount());
}
}
In the test code, we create multiple threads, each responsible for making a large number of increment operations on the MultiCoreCounter
. By running this test, we can observe how the LongAdder
implementation efficiently handles concurrent updates from a high number of threads.
A Final Look
In this blog post, we've explored the need for a scalable multi-core counter implementation and demonstrated how to achieve this using Java's LongAdder
class. By leveraging LongAdder
, we've created a highly concurrent and scalable counter that can efficiently handle updates across multiple threads without introducing contention.
As multi-core systems continue to dominate the computing landscape, it's crucial to design and implement concurrent data structures that can fully exploit the available hardware resources. By understanding and leveraging tools like LongAdder
, developers can build high-performance, concurrent applications that excel in multi-core environments.
By embracing scalable solutions and techniques, we can ensure that our applications not only meet present-day requirements but also remain well-prepared for the increasingly parallel nature of future computing systems.
In summary, the LongAdder
class provides a valuable tool for building scalable, concurrent applications in Java, and understanding its usage is essential for developers aiming to harness the full potential of multi-core systems.
To learn more about concurrent programming in Java and leveraging the power of multi-core systems, check out the official Java documentation on Concurrency.