Handling Concurrent Execution of Scala Futures
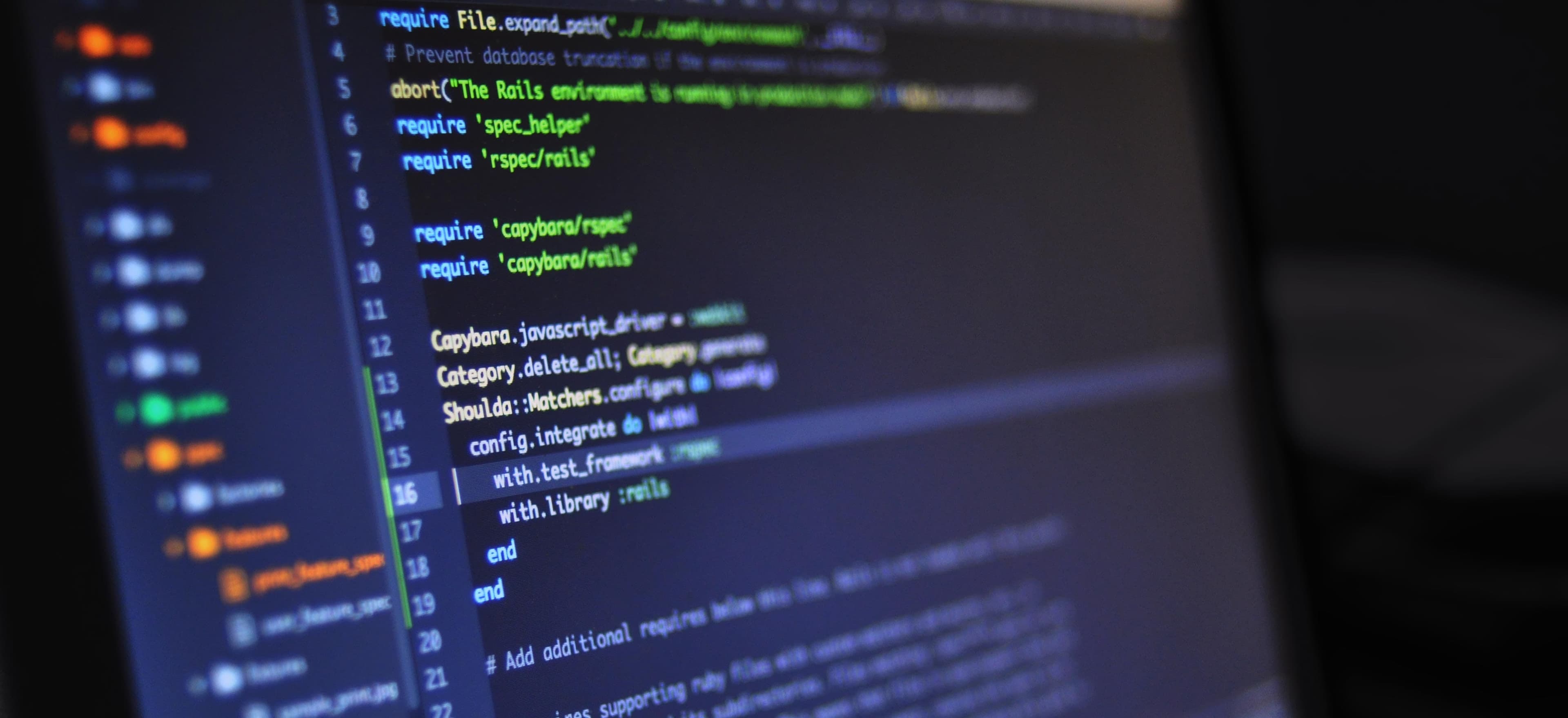
- Published on
Managing Concurrency with Scala Futures
In concurrent programming, managing asynchronous operations is crucial for building responsive and scalable applications. Scala, with its powerful concurrency and parallelism features, provides a convenient abstraction for managing concurrent tasks - Scala Futures.
What are Scala Futures?
In Scala, a Future is a placeholder object for a value that may be available at some point. It represents a computation that may not have been completed yet. Futures are often used to manage asynchronous operations, such as network requests, file I/O, or any task that may take time to complete.
Creating a Future
Creating a Future in Scala is straightforward. You can use the Future
object from the scala.concurrent
package to kick off a computation that will be executed asynchronously.
import scala.concurrent.Future
import scala.concurrent.ExecutionContext.Implicits.global
val futureResult: Future[Int] = Future {
// Perform some time-consuming computation
42
}
In this example, a Future is created to compute the value 42. The result of the computation is encapsulated within the Future
object.
Composing Futures
One of the powerful features of Scala Futures is the ability to compose and combine them to create more complex asynchronous workflows.
Chaining Futures with map and flatMap
You can use map
and flatMap
to chain the result of one Future to another. This allows you to perform sequential computations on the results of async operations.
val futureResult: Future[Int] = Future {
// Perform some time-consuming computation
42
}
val transformedFuture: Future[String] = futureResult.map(result => s"The answer is $result")
In this example, the map
function is used to transform the result of futureResult
into a new Future that holds a String.
Running Futures in Parallel with for-comprehension
Scala provides a convenient syntax for running Futures in parallel using for-comprehension.
val futureResult1: Future[Int] = Future {
// Perform some time-consuming computation
42
}
val futureResult2: Future[String] = Future {
// Perform another time-consuming computation
"Scala"
}
val combinedFuture: Future[String] = for {
result1 <- futureResult1
result2 <- futureResult2
} yield s"$result2 is the answer to $result1"
In this example, for-comprehension
allows futureResult1
and futureResult2
to execute concurrently, and the result is combined into a new Future.
Handling Future Completions
When working with Futures, it's essential to handle the completion of the asynchronous computation.
Callbacks with onComplete
You can use the onComplete
callback to perform actions once the Future completes, regardless of whether it succeeded or failed.
val futureResult: Future[Int] = Future {
// Perform some time-consuming computation
42
}
futureResult.onComplete {
case Success(result) => println(s"The result is $result")
case Failure(exception) => println(s"An error occurred: ${exception.getMessage}")
}
In this example, the onComplete
callback is used to print the result or the failure message.
Handling Errors with recover
The recover
method allows you to provide a fallback value in case the Future fails.
val futureResult: Future[Int] = Future {
// Perform some time-consuming computation that may fail
if (someCondition) throw new RuntimeException("Failed computation")
else 42
}
val resultWithRecovery: Future[Int] = futureResult.recover {
case e: RuntimeException => 0 // Fallback value
}
Here, recover
ensures that even if the computation fails, the result will be a valid Future containing the fallback value.
Managing Concurrency
When working with concurrent operations, it's important to control the degree of parallelism and manage the underlying execution context.
Controlling Parallelism with Future.sequence
The Future.sequence
method allows you to convert a Seq[Future[T]]
to a Future[Seq[T]]
, effectively running multiple Futures concurrently and combining their results.
val futureResults: Seq[Future[Int]] = Seq(
Future {
// Perform some time-consuming computation
42
},
Future {
// Perform another time-consuming computation
10
}
)
val combinedFuture: Future[Seq[Int]] = Future.sequence(futureResults)
By using Future.sequence
, you can execute all the futureResults
concurrently and obtain a single Future containing all the results.
Specifying Execution Context
The execution context is responsible for executing the computation within a Future. Scala provides an implicit global execution context, but you can also define custom execution contexts tailored to your specific requirements.
import scala.concurrent.ExecutionContext.Implicits.global
import scala.concurrent.ExecutionContext
val customExecutionContext: ExecutionContext = ExecutionContext.fromExecutor(Executors.newFixedThreadPool(10))
In this example, a custom execution context is created using a fixed thread pool with 10 threads.
Final Thoughts
Scala Futures provide a powerful abstraction for managing concurrent tasks in a simple and expressive manner. By leveraging operations like composition, error handling, and concurrency control, you can build responsive and efficient asynchronous workflows. Understanding how to handle concurrent execution with Scala Futures is crucial for developing robust and scalable applications.
In conclusion, mastering the usage of Scala Futures empowers developers to harness the full potential of concurrent and parallel programming, paving the way for high-performance and responsive applications.
Learning how to effectively manage asynchronous operations with Scala Futures is a valuable skill for any Scala developer, and mastering these techniques will undoubtedly elevate the quality and performance of concurrent Scala applications.
By incorporating best practices and understanding the nuances of Scala Futures, developers can build scalable, responsive, and fault-tolerant systems, thus unlocking the true potential of concurrent programming in the Scala ecosystem.
To delve deeper into concurrent programming with Scala, consider exploring Akka, a powerful toolkit and runtime for building highly concurrent, distributed, and resilient message-driven applications on the JVM.
For a comprehensive reference on Scala Futures and concurrent programming, the official Scala documentation provides in-depth insights and examples for further learning and exploration.