Optimizing JOOQ queries for improved performance
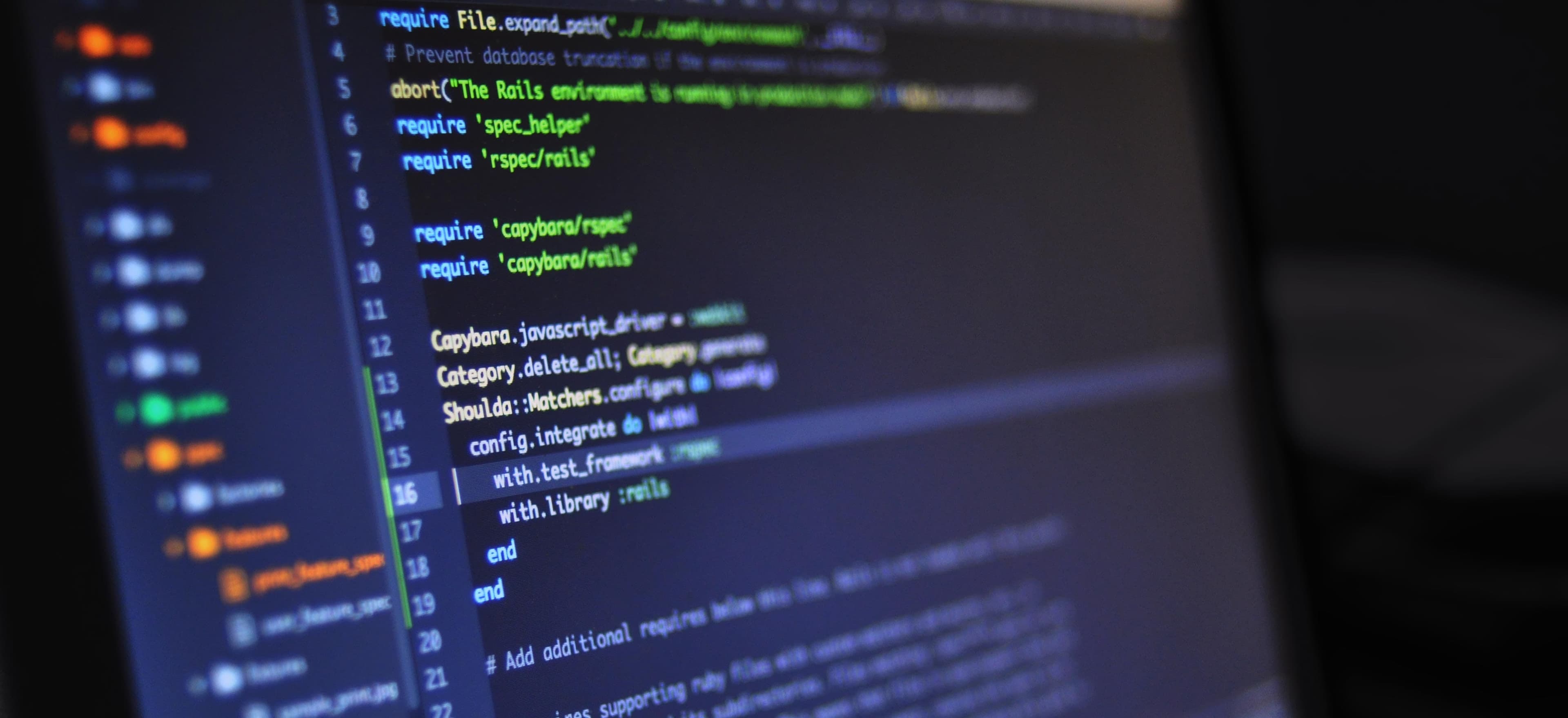
- Published on
Optimizing JOOQ Queries for Improved Performance
When working with databases in Java applications, developers often turn to Object-Relational Mapping (ORM) tools like JOOQ to interact with the database in a more object-oriented way. While JOOQ simplifies database access, writing efficient queries is crucial for achieving optimal performance. In this article, we'll explore various techniques to optimize JOOQ queries for improved performance.
1. Understanding Query Execution
Before delving into optimization techniques, it's essential to understand how JOOQ queries are executed. When you create a query using JOOQ, it generates SQL code to interact with the database. This SQL code is then executed against the database, and the results are returned to the application.
2. Avoiding N+1 Query Problem
One common pitfall when using JOOQ is the N+1 query problem, which occurs when a query inside a loop results in N additional queries to fetch related data. This can lead to a large number of queries being executed, causing performance issues. To mitigate this problem, you can use JOOQ's fetch and fetchOne methods to eagerly fetch related data, reducing the number of queries.
// Avoid N+1 query problem by using fetch
List<AuthorRecord> authors = create.selectFrom(AUTHOR).fetch();
List<BookRecord> books = create.selectFrom(BOOK).fetch();
In the above code snippet, we use JOOQ's fetch method to eagerly fetch all authors and books in a single query, avoiding the N+1 problem.
3. Utilizing Query Joins
Another way to optimize JOOQ queries is by utilizing query joins to fetch related data in a single query instead of making multiple queries. This reduces the round trips to the database and improves performance.
// Using query joins to fetch related data
Result<Record> result = create.select()
.from(AUTHOR)
.join(BOOK).on(AUTHOR.ID.eq(BOOK.AUTHOR_ID))
.fetch();
In the above example, we use a query join to fetch authors along with their books in a single query, improving the query's efficiency.
4. Indexing for Performance
Proper indexing of database tables is essential for efficient query execution. JOOQ allows you to specify indexes directly in your code using the Index and IndexHint classes. By leveraging indexes, you can significantly improve query performance for frequently accessed columns.
// Specifying index hints for query optimization
Result<Record> result = create.select()
.from(AUTHOR)
.hint("INDEX", "index_name")
.fetch();
By adding index hints to your queries, you can guide the database optimizer to use specific indexes, leading to improved query execution.
5. Batch Operations for Efficiency
When dealing with large datasets, performing batch operations can greatly improve performance. JOOQ provides support for batch inserts, updates, and deletes, allowing you to execute multiple operations in a single database round trip.
// Executing batch insert operation using JOOQ
create.batchInsertInto(BOOK, BOOK.ID, BOOK.TITLE)
.values(1, "First Book")
.values(2, "Second Book")
.execute();
By leveraging JOOQ's batch operations, you can minimize the number of round trips to the database, resulting in improved performance when dealing with multiple database operations.
6. Limiting Result Sets
Fetching large result sets can impact performance and consume significant memory. To mitigate this, you can use JOOQ's limit and offset clauses to restrict the number of rows returned by a query.
// Limiting the number of rows returned by a query
Result<Record> result = create.selectFrom(BOOK)
.orderBy(BOOK.ID)
.limit(10)
.offset(5)
.fetch();
By using the limit and offset clauses, you can control the size of result sets, preventing excessive memory consumption and improving query performance.
Closing the Chapter
Optimizing JOOQ queries is essential for achieving optimal performance in Java applications. By understanding query execution, avoiding the N+1 query problem, utilizing query joins, indexing for performance, employing batch operations, and limiting result sets, you can significantly improve the efficiency of your JOOQ queries. Applying these optimization techniques will lead to faster query execution and better overall application performance.
In conclusion, by fine-tuning JOOQ queries, developers can ensure that their Java applications interact with the database in the most efficient manner possible, resulting in improved performance and a smoother user experience.
For further insights into optimizing database performance, you can refer to the official JOOQ documentation and JOOQ best practices.