Optimizing Communication Between MVC Delivery Mechanism and Domain Model
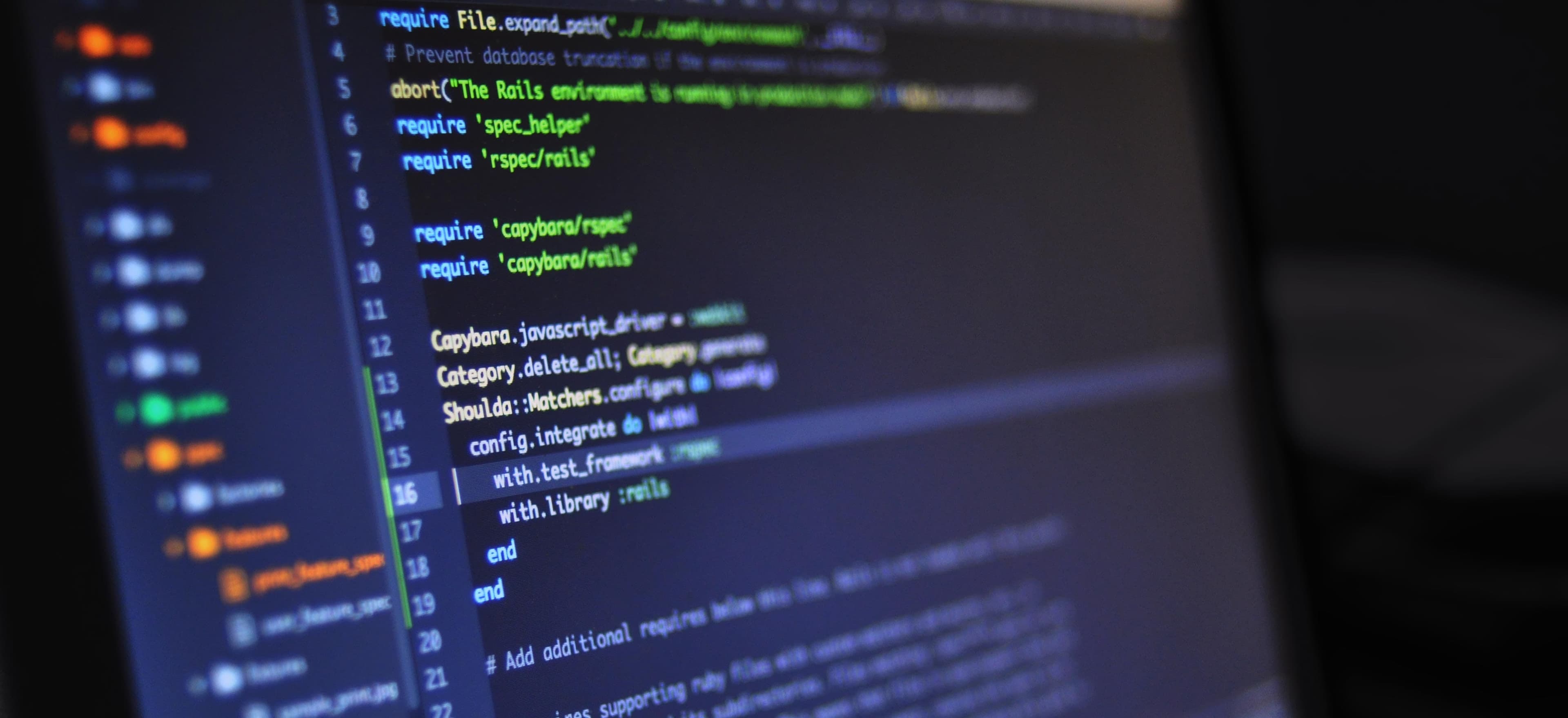
- Published on
Optimizing Communication Between MVC Delivery Mechanism and Domain Model
In a typical Model-View-Controller (MVC) architecture, the communication between the delivery mechanism (Views and Controllers) and the domain model (Models) is crucial for the overall performance and scalability of the application. In this blog post, we will explore various strategies for optimizing this communication in a Java-based MVC application.
Understanding the Communication Flow
Before diving into the optimization techniques, let's first understand the typical communication flow between the MVC delivery mechanism and the domain model.
- The user interacts with the View (UI components).
- The Controller receives the user's input and processes it.
- The Controller interacts with the Domain Model to retrieve or update data.
- The Domain Model performs the required business logic and interacts with the data storage (e.g., database).
- The Domain Model returns the results to the Controller.
- The Controller updates the View with the latest data.
This communication flow forms the backbone of the MVC architecture and optimizing each step is essential for better performance.
Optimizing Communication
1. Efficient Data Transfer Objects (DTOs)
When transferring data between the Controller and the Domain Model, it's crucial to use efficient Data Transfer Objects (DTOs). Instead of transferring entire domain objects, only transfer the required data to minimize the payload.
Example:
// Inefficient DTO
public class InefficientDTO {
private DomainObject domainObject;
// Other fields and getters/setters
}
// Efficient DTO
public class EfficientDTO {
private Long id;
private String name;
// Other required fields and getters/setters
}
In the above example, the EfficientDTO
only contains the required fields, leading to reduced data transfer overhead.
2. Utilizing Lazy Loading
In scenarios where the View does not immediately require certain data from the Domain Model, consider utilizing lazy loading. This can prevent unnecessary data retrieval from the storage layer, improving overall performance.
Example:
// Using lazy loading in Hibernate
@Entity
public class ParentEntity {
// Other fields
@OneToMany(mappedBy = "parent", fetch = FetchType.LAZY)
private List<ChildEntity> children;
// Getter and Setter
}
In this example, the children
collection will be lazily fetched when accessed, reducing the initial data retrieval overhead.
3. Caching Mechanisms
Introducing caching mechanisms, such as using Java's ConcurrentHashMap or third-party libraries like Ehcache, can significantly enhance the communication between the delivery mechanism and the domain model by reducing the need for frequent data retrieval operations.
Example:
// Using ConcurrentHashMap for caching
ConcurrentMap<String, String> cache = new ConcurrentHashMap<>();
cache.put("key", "value");
String cachedValue = cache.get("key");
In the above snippet, the ConcurrentHashMap
is used to cache key-value pairs for efficient data retrieval.
4. Asynchronous Communication
In scenarios where certain operations in the Domain Model are time-consuming and do not directly impact the View rendering, leveraging asynchronous communication can improve responsiveness.
Example:
// Using CompletableFuture for asynchronous operations
CompletableFuture.supplyAsync(() -> performLongRunningOperation())
.thenAccept(result -> handleResult(result));
Here, the CompletableFuture
allows the long-running operation to be executed asynchronously, preventing the blocking of the main thread.
5. Minimizing Network Overhead
When communicating between the delivery mechanism and the domain model in a distributed environment, minimizing network overhead becomes critical. Consider using protocols like Protocol Buffers or Apache Avro for efficient serialization and deserialization of data.
Closing the Chapter
Optimizing the communication between the MVC delivery mechanism and the domain model is essential for creating high-performing Java applications. By employing efficient DTOs, lazy loading, caching, asynchronous communication, and minimizing network overhead, developers can significantly enhance the overall responsiveness and scalability of the application.
Incorporating these optimization strategies not only improves the user experience but also ensures that the MVC architecture operates smoothly, providing a solid foundation for complex enterprise applications.
By understanding and implementing these optimization techniques, developers can elevate their Java MVC applications to new heights of performance and efficiency.
For further reading, check out the following resources:
- Java Concurrency in Practice by Brian Goetz
- Effective Java by Joshua Bloch
- Hibernate Documentation for in-depth understanding of lazy loading in Hibernate
Optimizing communication between MVC delivery mechanisms and domain models is an ongoing process, and staying updated with the latest industry best practices is crucial for continuously improving application performance.