Overcoming the Challenges of Agile Product Roadmap
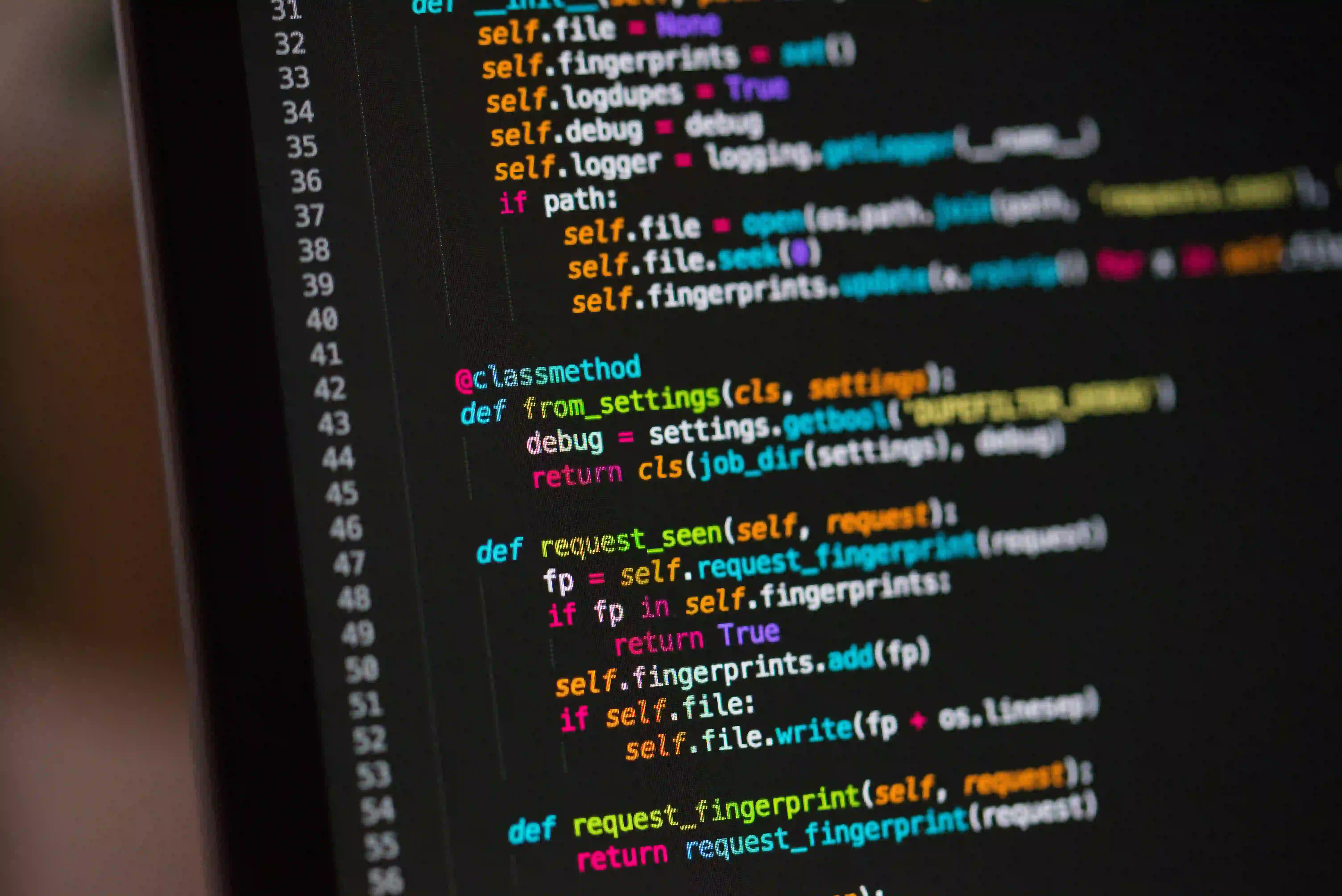
How to Create a Flexible Agile Product Roadmap using Java
In the world of software development, an agile product roadmap is crucial for guiding teams toward the successful delivery of products. However, creating and maintaining an agile product roadmap comes with its own set of challenges. Fortunately, Java provides powerful tools and libraries that can help developers overcome these challenges and build a flexible, adaptive, and efficient product roadmap.
What is an Agile Product Roadmap?
An agile product roadmap is a high-level visual summary that maps out the vision and direction of a product over time. It aligns the stakeholders and the development team by providing a strategic overview of where the product is headed and why. An effective agile product roadmap should be flexible, adaptable, and responsive to change, reflecting the iterative and collaborative nature of agile development.
Challenges of Agile Product Roadmap
1. Flexibility and Adaptability
- Agile environments demand frequent changes to the product roadmap. Maintaining flexibility while keeping the roadmap on track can be challenging.
2. Communication and Alignment
- Ensuring that all stakeholders understand and align with the product roadmap can be complex, especially when changes occur frequently.
3. Visual Representation
- Creating a visual representation that effectively communicates the roadmap's vision in a clear and understandable manner to all stakeholders can be daunting.
Overcoming Challenges with Java
Leveraging Java Libraries
Java offers a plethora of libraries and tools that can aid in creating and managing a flexible and visual agile product roadmap. Let's explore how Java can be used to overcome the aforementioned challenges.
Flexibility and Adaptability
Java's Spring Framework provides robust support for creating RESTful APIs, allowing for seamless integration with databases and other systems. By leveraging Spring Boot, developers can build microservices that are inherently flexible and adaptable, enabling the product roadmap to evolve as requirements change.
@RestController
@RequestMapping("/roadmap")
public class RoadmapController {
@Autowired
private RoadmapService roadmapService;
@GetMapping("/{id}")
public Roadmap getRoadmap(@PathVariable Long id) {
return roadmapService.getRoadmapById(id);
}
// Additional endpoints for updating and modifying the roadmap
}
In the above code snippet, the use of Spring's @RestController
and @Autowired
annotations demonstrates how Java, and specifically Spring Boot, can facilitate the creation of flexible and adaptable endpoints for managing the agile product roadmap.
Communication and Alignment
Effective communication and alignment can be achieved through the use of Java's WebSocket API, which enables real-time, bi-directional communication between clients and the server. By implementing WebSocket in conjunction with a messaging protocol such as STOMP (Simple Text Oriented Messaging Protocol), stakeholders can receive instant updates, fostering alignment and understanding of the product roadmap.
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfig extends AbstractWebSocketMessageBrokerConfigurer {
@Override
public void registerStompEndpoints(StompEndpointRegistry registry) {
registry.addEndpoint("/roadmap-updates").withSockJS();
}
@Override
public void configureMessageBroker(MessageBrokerRegistry registry) {
registry.enableSimpleBroker("/topic");
registry.setApplicationDestinationPrefixes("/app");
}
}
The code snippet above demonstrates how the WebSocket API can be configured using Java's WebSocketConfig class to enable real-time communication for sharing agile product roadmap updates.
Visual Representation
JavaFX, a powerful Java library for creating desktop applications, provides a comprehensive set of APIs for building interactive and visually appealing graphical user interfaces. By leveraging JavaFX, developers can design intuitive and visually compelling representations of the agile product roadmap, enhancing stakeholder understanding and buy-in.
public class RoadmapUI extends Application {
@Override
public void start(Stage stage) {
// Code for creating the visual representation of the roadmap
}
// Additional methods for updating and modifying the visual representation
}
In the above code snippet, the use of JavaFX's Application class exemplifies how Java can be utilized to create a visually engaging representation of the agile product roadmap.
The Closing Argument
Building a flexible and adaptive agile product roadmap is essential for navigating the dynamic landscape of software development. By harnessing the power of Java and its associated libraries, developers can overcome the challenges of flexibility, communication, and visual representation, ultimately paving the way for the successful implementation of an agile product roadmap.
While Java provides a robust foundation for addressing these challenges, it's essential to stay updated with the latest advancements in Java development. Incorporating best practices and exploring new libraries and frameworks will further enhance the capabilities of Java in the context of agile product roadmap management.
By combining the principles of agile development with the strengths of Java, teams can effectively steer their product development efforts towards success, embracing change and delivering value to stakeholders in an ever-evolving market landscape.