Understanding Hibernate Query Cache
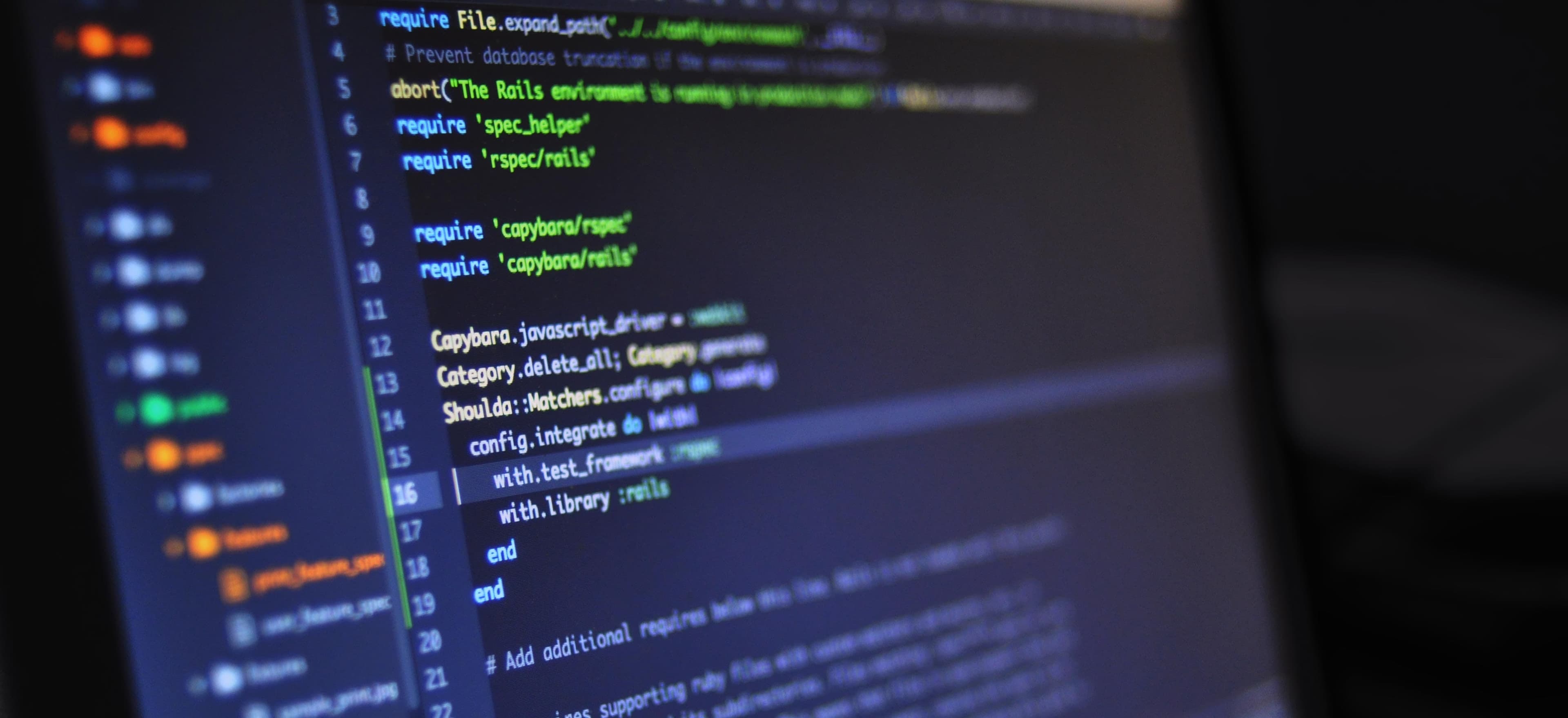
- Published on
Understanding Hibernate Query Cache
When working with Hibernate, performance optimization is crucial, especially when dealing with frequently accessed data. One way to improve performance is by utilizing the Hibernate Query Cache. This cache stores the results of queries, thus reducing the need to hit the database repeatedly for the same data. In this article, we will dive into the details of Hibernate Query Cache, its benefits, considerations, and how to use it effectively.
What is Hibernate Query Cache?
In Hibernate, the Query Cache is a second-level cache that caches query results. When a query is executed, the Query Cache stores the result set along with the query criteria. If the same query is executed again with the same parameters, Hibernate can retrieve the result from the cache instead of querying the database again. This can significantly improve the application's performance, especially for frequently executed queries.
Benefits of Hibernate Query Cache
-
Improved Performance: By caching query results, the time-consuming process of fetching data from the database can be avoided for repeated queries.
-
Reduced Database Load: With the query results cached, the database server is relieved of processing identical queries repeatedly, leading to a reduction in database load.
-
Scalability: Caching query results can improve the scalability of the application by reducing the load on the database, allowing it to handle a larger number of concurrent users.
Considerations before Using Hibernate Query Cache
Before enabling the Hibernate Query Cache, it's essential to consider the following aspects:
-
Nature of Data: Evaluate the nature of the data being queried and determine if caching query results will indeed lead to performance improvement. For data that rarely changes or is frequently accessed, caching can be beneficial.
-
Memory Usage: Caching query results consumes memory. It's crucial to assess the available memory and the potential impact of caching on the overall application performance.
-
Cache Invalidation: Hibernate Query Cache needs to be properly invalidated when the underlying data changes. Consider the cache invalidation strategy to ensure that stale data is not returned.
Using Hibernate Query Cache
To utilize the Hibernate Query Cache, several configurations and considerations need to be addressed.
Enable the Second-Level Cache
To enable the second-level cache in Hibernate, the hibernate.cache.use_second_level_cache
property needs to be set to true
in the Hibernate configuration file (hibernate.cfg.xml
or persistence.xml
for JPA).
<property name="hibernate.cache.use_second_level_cache">true</property>
Enable the Query Cache
Next, the Query Cache itself needs to be enabled using the hibernate.cache.use_query_cache
property.
<property name="hibernate.cache.use_query_cache">true</property>
Set Cacheable Attribute
In the query execution code, specify that the query results are cacheable by using the setCacheable(true)
method.
List results = session.createQuery("from EntityName")
.setCacheable(true)
.list();
Cache Region
Optionally, a cache region name can be assigned to the specific query results using the setCacheRegion("regionName")
method. This allows for more precise control over caching.
List results = session.createQuery("from EntityName")
.setCacheable(true)
.setCacheRegion("entityCacheRegion")
.list();
Cache Invalidation
Cache invalidation is a crucial aspect of using the Hibernate Query Cache. Stale data must be invalidated to ensure that the cached results remain accurate. Hibernate provides several mechanisms for cache invalidation.
Timestamp-Based Cache Invalidation
Hibernate supports timestamp-based cache invalidation, where each entity within the cache is associated with a timestamp. When the data is updated, the associated timestamp is also updated, and the cache is invalidated accordingly.
@org.hibernate.annotations.Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
public class EntityName {
// Entity mapping details
}
By using the @Cache
annotation with CacheConcurrencyStrategy.READ_WRITE
, Hibernate manages the cache invalidation based on timestamps.
Manual Cache Invalidation
For more specific cache invalidation needs, Hibernate provides the Evict
and UpdateTimestampsCache
APIs to manually evict entities or update timestamps in the cache.
sessionFactory.getCache().evictEntityRegion(EntityName.class);
sessionFactory.getCache().updateTimestampsCache(Collections.singleton("entityCacheRegion"), timestamp);
The Bottom Line
In conclusion, the Hibernate Query Cache is a powerful tool for improving application performance by caching frequently accessed query results. By carefully considering the nature of the data, memory usage, and cache invalidation strategies, the Query Cache can be effectively utilized. When used in conjunction with the second-level cache and proper cache invalidation mechanisms, the Hibernate Query Cache can significantly enhance the overall responsiveness and scalability of Hibernate-based applications.
By understanding and effectively utilizing the Hibernate Query Cache, developers can ensure optimal performance for applications that involve frequent database interactions.
To delve deeper into Hibernate Query Cache, you can refer to the official Hibernate documentation on Query Cache.
Remember, when implementing Hibernate Query Cache, always measure the performance impact with and without caching to ensure that it indeed improves the application's performance.