Handling Case Sensitivity in Scala Programming
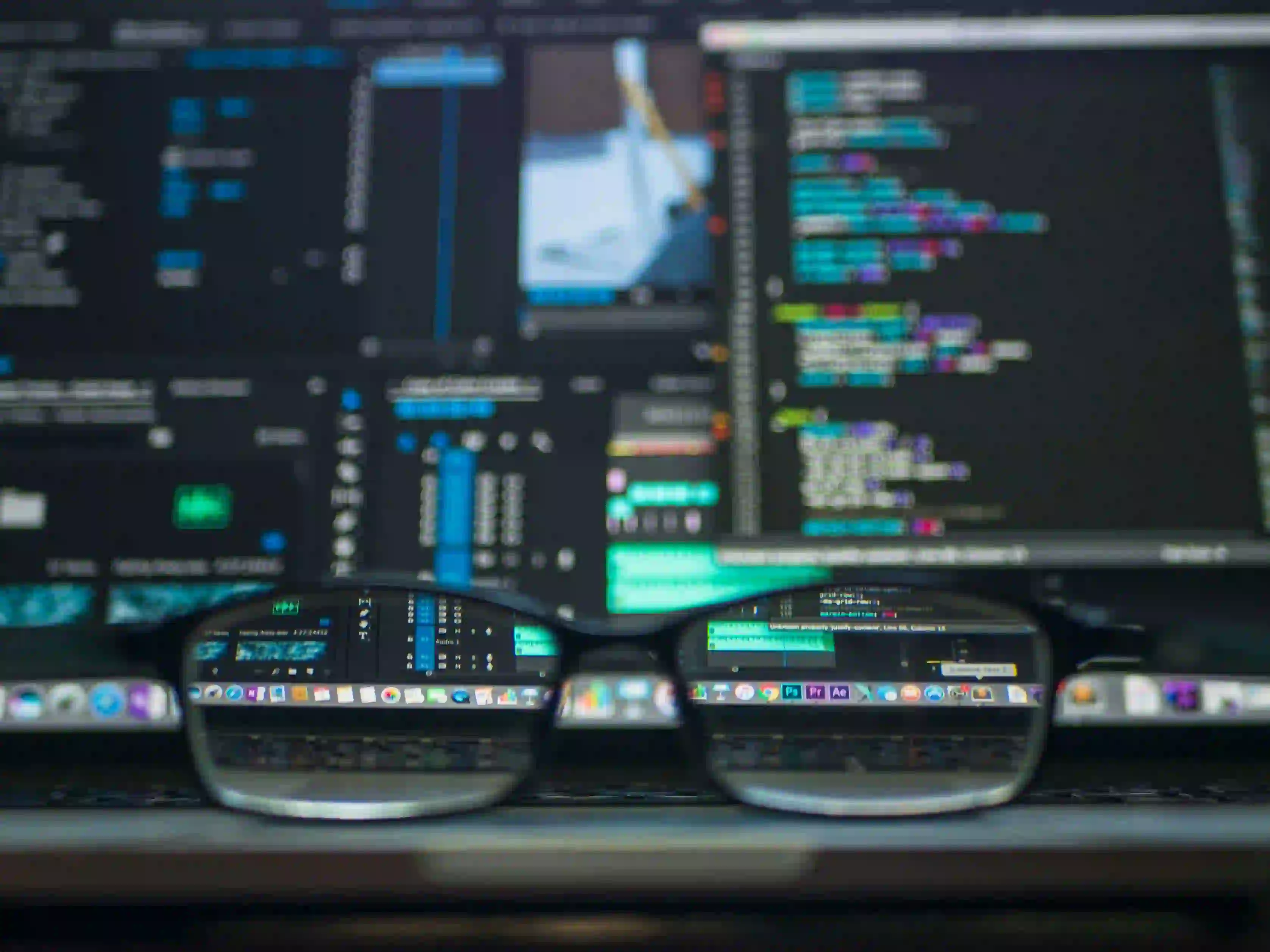
The Importance of Handling Case Sensitivity in Java Programming
Java is a powerful and versatile language widely used in software development. One important aspect to consider when working with Java is case sensitivity. Java is case-sensitive, meaning it distinguishes between uppercase and lowercase letters. This characteristic affects various aspects of Java programming, including variable naming, method calling, and package importing.
Variables and Identifiers
In Java, variable names and identifiers are case-sensitive. This means that myVariable
and myvariable
would be treated as two different variables. It's essential to maintain consistent naming conventions throughout your code to avoid potential errors related to case sensitivity.
int myVariable = 10;
int myvariable = 20; // This is a different variable than myVariable
When working with Java, it's a good practice to use camelCase for variable names, where the first word is lowercase and subsequent words are capitalized. Adhering to a consistent naming convention helps improve code readability and maintainability.
Method Invocations
When invoking methods in Java, it's crucial to adhere to the exact method name, including its case. Failure to do so can result in compilation errors or unexpected program behavior.
String message = "Hello, World!";
System.out.println(message); // Correct usage of println() method
System.out.Println(message); // This will result in a compilation error due to incorrect method name
Package Names
Package names in Java are also case-sensitive. This means that when importing packages, you must specify the exact case used in the package declaration. Failing to do so will lead to compilation errors.
import java.util.ArrayList;
import import Java.Util.ArrayList; // Incorrect package name due to mismatch in case
Handling Case Sensitivity in Java
Now that we understand the significance of case sensitivity in Java, let's explore some best practices for handling it effectively in our code:
Consistent Naming Conventions
Adopting consistent naming conventions for variables, methods, and packages is crucial in Java programming. By following a standard naming style, such as camelCase for variables and methods, and lowercase for package names, you can mitigate the risk of case sensitivity-related issues.
Code Reviews and Linting
Incorporating code reviews and automated code linting tools can help identify and rectify case sensitivity errors early in the development process. Code reviews allow peers to provide feedback on naming conventions and potential case sensitivity issues, while linters can flag inconsistencies in naming styles.
IDE Support
Modern Integrated Development Environments (IDEs) provide features to assist in handling case sensitivity effectively. IDEs often include functionalities such as auto-completion and on-the-fly error highlighting, which can aid in maintaining consistent naming conventions and detecting case-related issues.
Documentation and Training
Educating team members about the significance of case sensitivity and enforcing best practices through comprehensive documentation and training sessions can significantly reduce the occurrence of case sensitivity-related errors in Java codebases.
Key Takeaways
In conclusion, understanding and effectively managing case sensitivity is paramount in Java programming. By adhering to consistent naming conventions, leveraging code reviews and IDE support, and emphasizing the importance of case sensitivity through documentation and training, developers can minimize the occurrence of errors stemming from case sensitivity in their Java projects.
Remember, in Java, attention to detail matters—including the casing of your code. By implementing best practices and staying mindful of case sensitivity, you can ensure the robustness and reliability of your Java applications.
For more in-depth insights into Java programming, check out Oracle's Java documentation, where you can explore comprehensive resources on Java best practices and language specifications.
Happy coding!