Custom search providers not working with Apache CXF
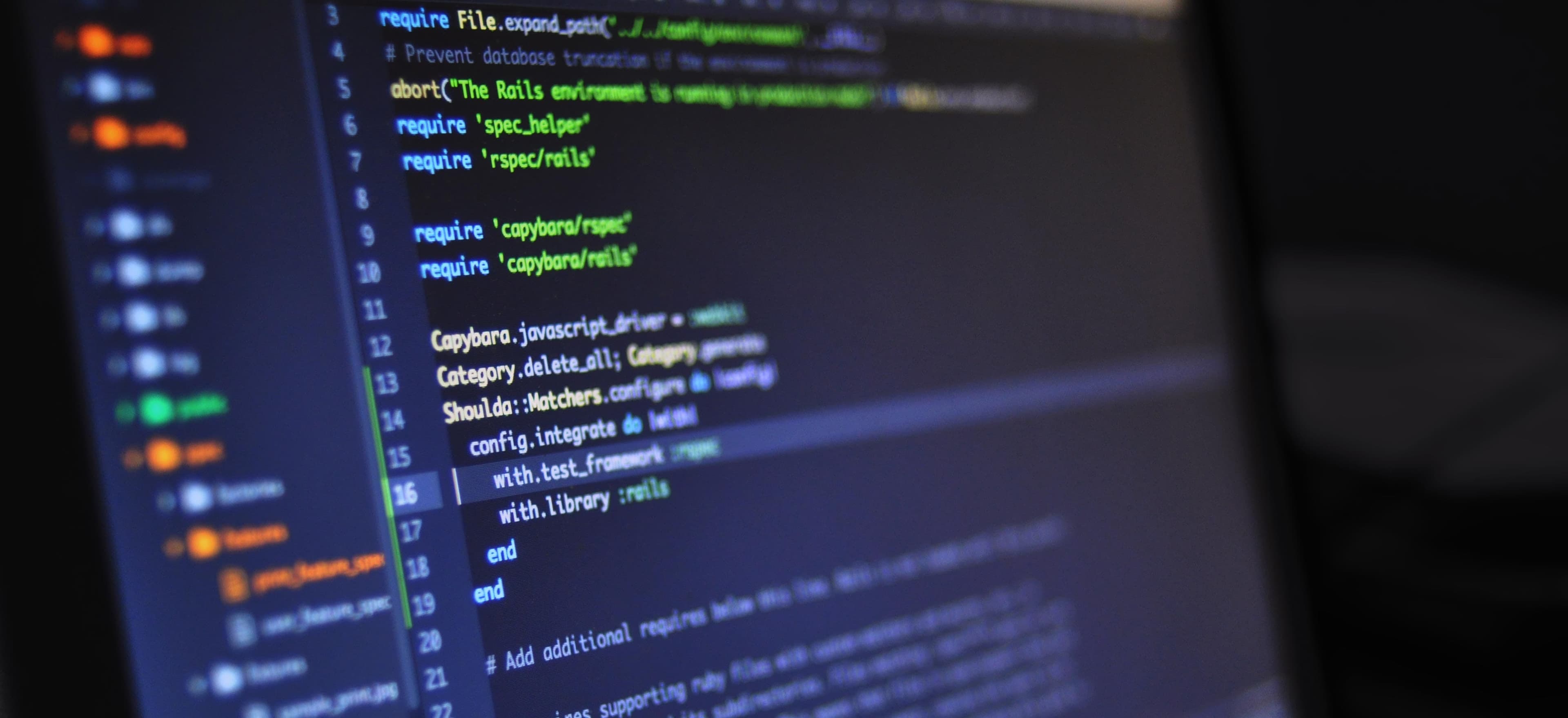
- Published on
Troubleshooting Custom Search Providers in Apache CXF
Apache CXF is a powerful framework for building and consuming web services. One common use case is implementing custom search providers to enhance the functionality of your application. However, there are instances where these custom search providers may not work as expected, leading to frustration and confusion among developers.
In this article, we will explore the potential reasons why custom search providers may not be working with Apache CXF and provide solutions to address these issues.
Understanding Custom Search Providers in Apache CXF
Before delving into the troubleshooting process, it's crucial to understand the concept of custom search providers in Apache CXF. Custom search providers are responsible for handling search queries against a particular data source, such as a database, and returning relevant results to the client.
Implementing a custom search provider involves creating a class that extends the AbstractSearchConditionVisitor
provided by Apache CXF. This class defines the logic for processing search criteria and generating the corresponding query to fetch the desired data.
public class CustomSearchProvider extends AbstractSearchConditionVisitor<MyEntity> {
// Custom logic for processing search criteria
}
Common Issues and Solutions
1. Incorrect Configuration
Issue:
The most common reason for custom search providers not working is incorrect configuration within the Apache CXF application.
Solution:
Ensure that the custom search provider class is properly registered with the CXF JAX-RS server. This can be done by adding the custom provider to the list of providers in the JAX-RS configuration.
MyCustomSearchProvider customSearchProvider = new MyCustomSearchProvider();
JAXRSServerFactoryBean sf = new JAXRSServerFactoryBean();
sf.setProvider(customSearchProvider);
2. Unsupported Search Criteria
Issue:
The custom search provider may not be handling all possible search criteria, leading to unexpected behavior when certain queries are executed.
Solution:
Review the implementation of the custom search provider to ensure that it can handle a wide range of search criteria. Implement support for additional search conditions by extending the provider's logic.
public class CustomSearchProvider extends AbstractSearchConditionVisitor<MyEntity> {
@Override
public void visit(SearchCondition<SomeType> condition) {
// Handle the new search condition
}
}
3. Missing Dependencies
Issue:
In some cases, custom search providers may rely on external libraries or dependencies that are not properly included in the project.
Solution:
Double-check the project's dependencies to ensure that all required libraries for the custom search provider are included in the classpath. Use a build tool like Maven or Gradle to manage dependencies effectively.
4. Inconsistent Data Source Configuration
Issue:
If the custom search provider interacts with a specific data source, such as a database, any inconsistencies or errors in the data source configuration can result in the provider not working as expected.
Solution:
Verify the configuration settings for the data source and ensure that the custom search provider has the necessary access and permissions to retrieve data from the source. Additionally, perform thorough testing to identify any issues with data retrieval.
5. Unhandled Exceptions
Issue:
Uncaught exceptions within the custom search provider can disrupt its functionality, leading to unexpected failures in processing search queries.
Solution:
Implement proper exception handling within the custom search provider to gracefully manage any potential errors that may occur during query processing. This includes handling database connectivity issues, query execution exceptions, and other runtime errors.
To Wrap Things Up
Troubleshooting custom search providers in Apache CXF requires a systematic approach to identify and address potential issues. By understanding the underlying causes and applying the suggested solutions, developers can ensure that their custom search providers function as intended, providing robust search capabilities within their CXF-based applications.
For more in-depth information on Apache CXF and custom search providers, refer to the official documentation. Additionally, engaging with the CXF community through forums and mailing lists can provide valuable insights and assistance in resolving specific issues related to custom search providers.