Creating Reusable Custom Composite Controls
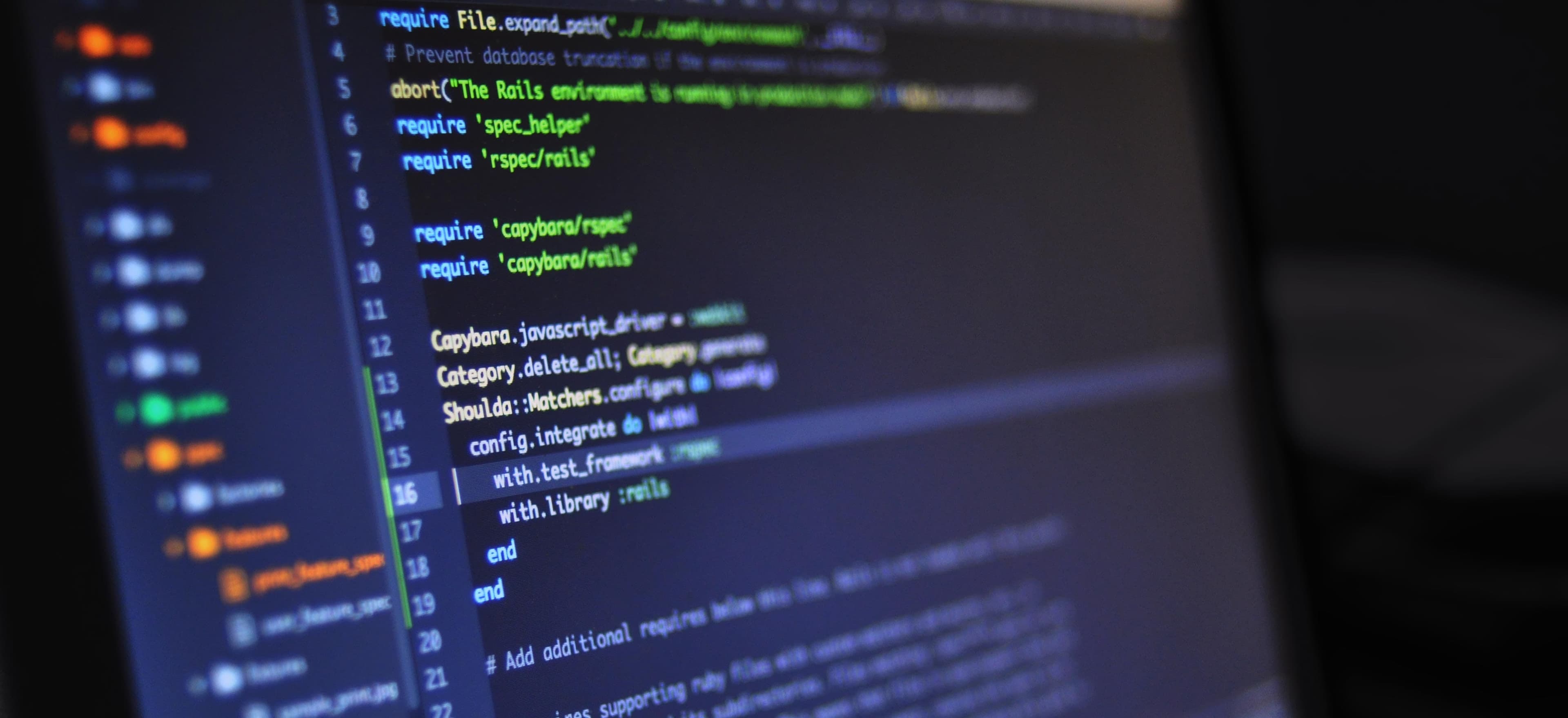
- Published on
Essentials at a Glance
In Java, composite controls refer to a group of UI components that are bundled together to provide a specific functionality. Custom composite controls enable developers to encapsulate a set of functionalities into a single, reusable component. This blog post explores the concept of creating custom composite controls in Java, the benefits they offer, and how to design them effectively.
Why Use Custom Composite Controls?
Custom composite controls offer several advantages:
- Reusability: They can be reused across different parts of an application or even across multiple projects, promoting code reusability and maintainability.
- Abstraction: Composite controls abstract complex functionalities into a single, easy-to-use component, reducing the complexity of individual UI components.
- Consistency: They promote consistency in the UI by encapsulating design patterns and behaviors, ensuring a uniform user experience across the application.
Designing Custom Composite Controls
When designing custom composite controls in Java, there are a few key considerations to keep in mind:
Encapsulation of Functionality
A custom composite control should encapsulate a cohesive set of functionalities that are related to each other. For example, a "file upload" composite control might encapsulate the file selection, upload progress, and completion message.
Configurability
It's important to make the custom composite control configurable to cater to varying requirements. Providing customizable properties and event handlers enhances the flexibility of the control.
Event Handling
Custom composite controls should facilitate communication with the parent container by exposing events that notify the parent when certain actions occur within the control.
Separation of Concerns
Following the principle of separation of concerns, the business logic and the UI presentation should be decoupled within the custom composite control. This separation enhances maintainability and testability.
Implementing Custom Composite Controls in Java
Let's dive into the implementation of a custom composite control in Java. In this example, we'll create a custom composite control for a "Color Picker" component.
Step 1: Create the ColorPicker Control Class
public class ColorPicker extends JPanel {
private JColorChooser colorChooser;
public ColorPicker() {
colorChooser = new JColorChooser();
add(colorChooser);
}
public Color getSelectedColor() {
return colorChooser.getColor();
}
}
In this code snippet, we define a ColorPicker
class that extends the JPanel
class. It contains a JColorChooser
component to enable color selection.
Step 2: Using the ColorPicker Control
public class Main {
public static void main(String[] args) {
JFrame frame = new JFrame("Color Picker Demo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
ColorPicker colorPicker = new ColorPicker();
frame.add(colorPicker);
frame.pack();
frame.setVisible(true);
}
}
In this code snippet, we demonstrate how to use the ColorPicker
control within a JFrame
. We instantiate the ColorPicker
control and add it to the frame.
Wrapping Up
Custom composite controls are a powerful tool for creating reusable, encapsulated components in Java applications. By following best practices in their design and implementation, developers can streamline the development process, improve code maintainability, and ensure a consistent user experience across their applications. Incorporating custom composite controls can significantly enhance the modularity and flexibility of Java applications.
In conclusion, the process of creating custom composite controls involves encapsulating related functionalities, making the control configurable, handling events, and maintaining a clear separation of concerns. With these principles in mind, developers can leverage custom composite controls to build robust, reusable components in their Java applications.
By mastering the art of creating custom composite controls, Java developers can unlock the full potential of code reusability and maintainability, leading to more efficient and scalable applications.
For further reading on the topic of custom composite controls, consider exploring the official Java Swing documentation. Additionally, the Java Custom Components article on Baeldung provides valuable insights into creating custom components in Java.