Optimizing CSV Data Import in PostgreSQL
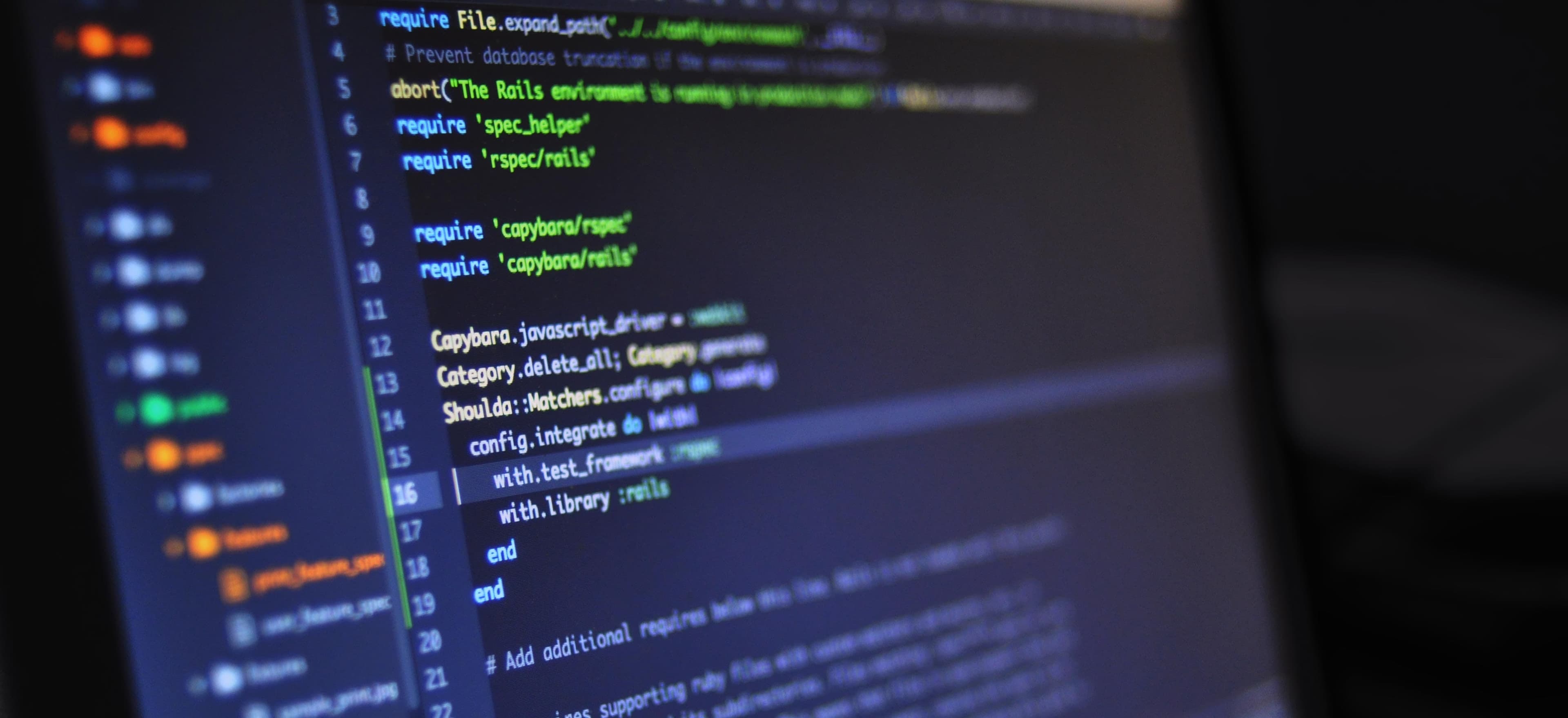
- Published on
Optimizing CSV Data Import in PostgreSQL
When dealing with large datasets, the efficiency of importing data into a PostgreSQL database becomes crucial. In this blog post, we will explore various techniques to optimize the import of CSV data into PostgreSQL using Java.
Using COPY Command
One of the most efficient ways to import CSV data into PostgreSQL is by using the COPY
command. This command bypasses the SQL layer and directly reads from or writes to a file, making it significantly faster than using INSERT
statements. Using Java, we can execute the COPY
command using the JDBC driver.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.Statement;
public class PostgresDataImport {
public static void main(String[] args) {
String url = "jdbc:postgresql://localhost:5432/mydatabase";
String user = "username";
String password = "password";
try {
Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement();
String filePath = "/path/to/data.csv";
String tableName = "mytable";
String copyQuery = "COPY " + tableName + " FROM '" + filePath + "' CSV HEADER";
statement.execute(copyQuery);
statement.close();
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
In the above code, we establish a connection to the PostgreSQL database and execute the COPY
command to import data from the CSV file into the specified table. The CSV HEADER
option indicates that the first line of the CSV file contains the column headers.
Batch Insertion
Another approach to optimize data import is by using batch insertion. Instead of executing individual INSERT
statements for each row, we can group multiple rows into a single transaction and execute them together. This reduces the overhead of multiple network round-trips and improves overall performance.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class PostgresBatchInsert {
public static void main(String[] args) {
String url = "jdbc:postgresql://localhost:5432/mydatabase";
String user = "username";
String password = "password";
try {
Connection connection = DriverManager.getConnection(url, user, password);
connection.setAutoCommit(false);
String insertQuery = "INSERT INTO mytable (col1, col2, col3) VALUES (?, ?, ?)";
PreparedStatement statement = connection.prepareStatement(insertQuery);
// Assuming data is read from CSV and stored in a List of arrays
List<Object[]> data = getDataFromCSV();
for (Object[] row : data) {
statement.setObject(1, row[0]);
statement.setObject(2, row[1]);
statement.setObject(3, row[2]);
statement.addBatch();
}
statement.executeBatch();
connection.commit();
statement.close();
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
In the above code, we batch insert data into the PostgreSQL database using PreparedStatement
and addBatch()
method. The setObject()
method is used to set the values for each row before adding it to the batch. Finally, executeBatch()
is called to execute all the batched statements at once.
Indexes and Constraints
When importing large amounts of data, it is essential to consider the presence of indexes and constraints on the target table. While indexes can improve query performance, they also add overhead to data modification operations like insertion. Therefore, it's beneficial to temporarily disable or drop indexes and constraints during the import process and rebuild them once the data import is complete.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.Statement;
public class PostgresIndexConstraints {
public static void main(String[] args) {
String url = "jdbc:postgresql://localhost:5432/mydatabase";
String user = "username";
String password = "password";
try {
Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement();
// Disable indexes and constraints
statement.execute("ALTER TABLE mytable DISABLE TRIGGER ALL");
statement.execute("ALTER TABLE mytable DROP CONSTRAINT ...");
// Perform data import
// ...
// Rebuild indexes and constraints
statement.execute("ALTER TABLE mytable ENABLE TRIGGER ALL");
statement.execute("ALTER TABLE mytable ADD CONSTRAINT ...");
statement.close();
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
In the above code, we use SQL commands to disable triggers and drop constraints before the data import process. Once the import is complete, we re-enable triggers and re-add constraints as required.
The Bottom Line
Optimizing the import of CSV data into PostgreSQL using Java involves leveraging efficient techniques such as the COPY
command, batch insertion, and managing indexes and constraints. By implementing these strategies, you can significantly improve the performance of data import operations, especially when dealing with large datasets.
In summary, when working with PostgreSQL data import, it's essential to consider the most efficient methods and strategies to ensure optimal performance and reliability.
For further reading on PostgreSQL optimization, you can refer to the official PostgreSQL documentation and explore advanced optimization techniques for specific use cases.
Optimizing the import of CSV data in PostgreSQL is crucial for efficient database management, and with the right strategies and tools, you can streamline the process for better performance and scalability.
Checkout our other articles