Managing Event Dependencies: A How-to Guide
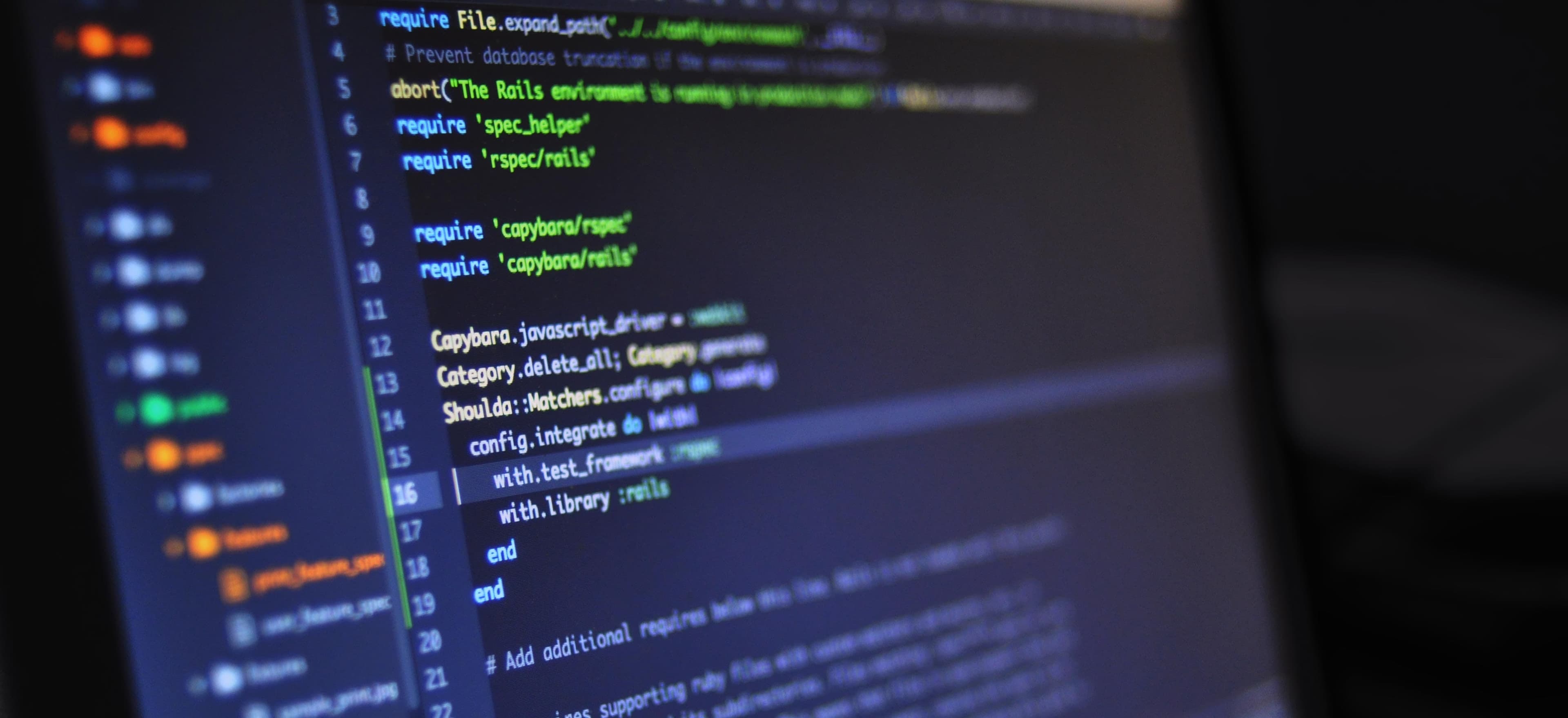
- Published on
Managing Event Dependencies: A How-to Guide
In the realm of software development, event-driven architecture has become increasingly popular due to its flexibility and scalability. However, managing event dependencies can often pose a significant challenge. In this article, we'll explore the concept of event dependencies and discuss effective strategies for managing them in Java applications.
Understanding Event Dependencies
Event dependencies occur when the processing of an event is reliant on the occurrence or outcome of another event. In other words, the execution of one event is dependent on the successful completion of another event. Failing to manage these dependencies can result in race conditions, inconsistent data, and overall system instability.
Let's delve into a common scenario to better illustrate event dependencies. Consider an e-commerce application where the processing of an order depends on the inventory being updated. Here, the 'order placed' event is dependent on the 'inventory updated' event. If the inventory is not updated before the order is processed, it could lead to overselling of products.
Managing Event Dependencies in Java
Java, with its robust ecosystem and mature libraries, provides several approaches to effectively manage event dependencies. Here are some key strategies:
1. Asynchronous Event Handling
Asynchronous event handling allows events to be processed independently, reducing the risk of blocking the main thread. The CompletableFuture
class in Java simplifies asynchronous programming by representing a future result of an asynchronous computation.
CompletableFuture<Void> inventoryUpdateFuture = CompletableFuture.runAsync(() -> updateInventory());
CompletableFuture<Void> orderProcessingFuture = inventoryUpdateFuture.thenRunAsync(() -> processOrder());
In this example, the thenRunAsync
method ensures that the order processing doesn't start until the inventory update is successfully completed.
2. Event Sourcing
Event sourcing involves capturing all changes to an application state as a sequence of events. These events can then be replayed to rebuild state or derive new views. The Axon Framework, a popular Java framework, provides support for event-sourced aggregates and event-driven architectures.
@Aggregate
public class InventoryAggregate {
@AggregateIdentifier
private String productId;
private int availableQuantity;
@CommandHandler
public InventoryAggregate(UpdateInventoryCommand command) {
apply(new InventoryUpdatedEvent(command.getProductId(), command.getQuantity()));
}
@EventSourcingHandler
public void on(InventoryUpdatedEvent event) {
this.availableQuantity += event.getQuantity();
}
}
By leveraging event sourcing, dependencies between events can be explicitly managed within the aggregate's state transitions.
3. Using Message Brokers
Message brokers such as Apache Kafka and RabbitMQ play a vital role in managing event dependencies by enabling asynchronous communication between decoupled components. These brokers provide features like message persistence, partitioning, and replication, ensuring reliable event delivery.
// Using Apache Kafka as a message broker to handle event dependencies
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
producer.send(new ProducerRecord<>("inventory_updates", "product1", "10 units added"));
producer.send(new ProducerRecord<>("order_placed", "order123", "product1"));
By leveraging a message broker, events can be reliably produced and consumed, ensuring that dependent events are processed in the correct order.
Closing Remarks
Effectively managing event dependencies is crucial for building robust, scalable, and consistent systems. In the context of Java applications, leveraging asynchronous handling, event sourcing, and message brokers can significantly enhance the management of event dependencies.
By implementing these strategies, developers can mitigate the risks associated with event-dependent processing and ensure the overall stability and reliability of their applications.
To delve deeper into event-driven architecture and event dependency management in Java, consider exploring the following resources:
- Java CompletableFuture Documentation
- Axon Framework Official Website
- Apache Kafka Documentation
- RabbitMQ Tutorials
Now that you have a solid understanding of managing event dependencies in Java applications, it's time to apply these concepts to your own projects and witness the benefits firsthand. Happy coding!