Optimizing Indexing and Searching in Apache Lucene 5.0
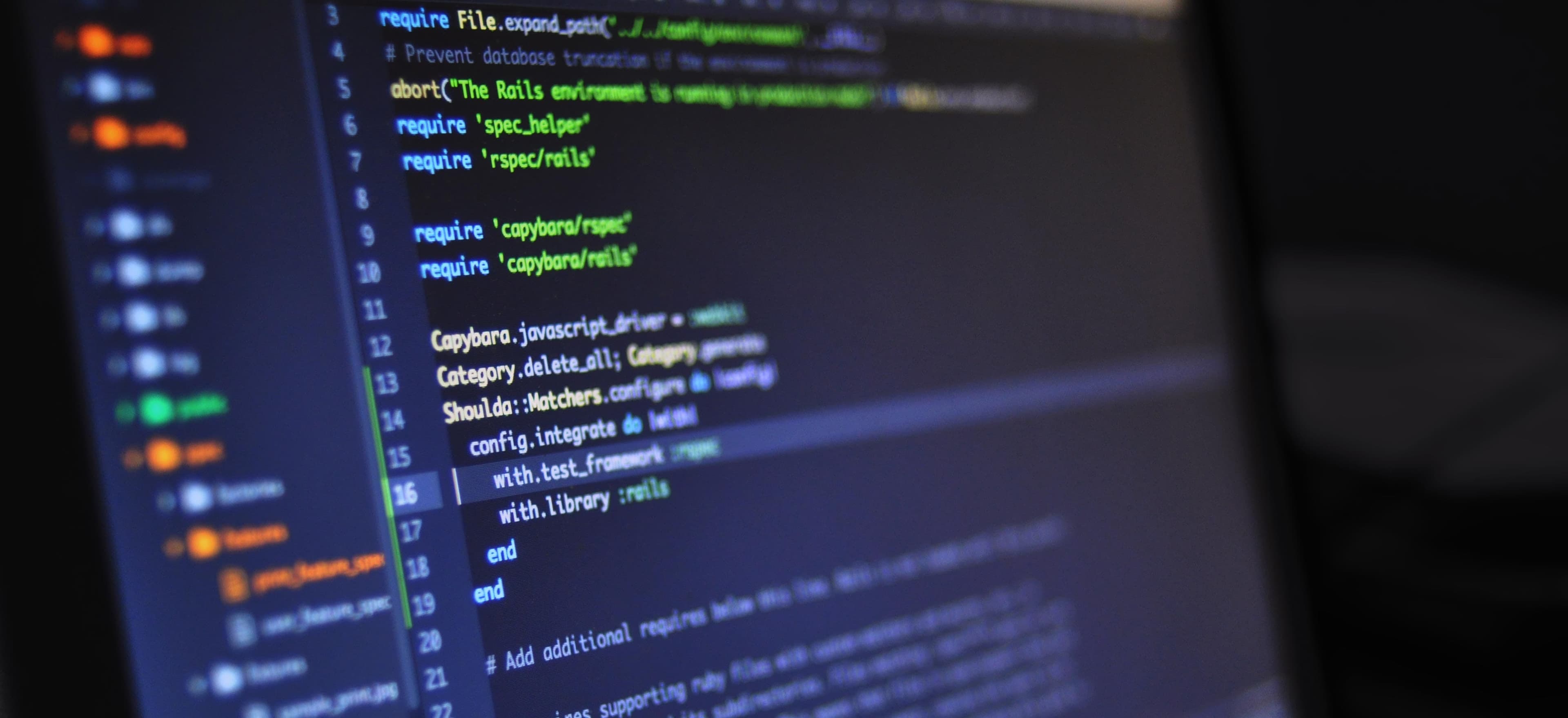
- Published on
Optimizing Indexing and Searching in Apache Lucene 5.0
When it comes to efficient and powerful search capabilities, Apache Lucene 5.0 stands out as a robust and high-performance indexing and searching library for Java. In this blog post, we will explore some key techniques and best practices for optimizing indexing and searching in Apache Lucene 5.0, to ensure that your search applications are not only fast but also scalable and reliable.
Understanding Apache Lucene
Apache Lucene is a full-featured text search engine library written entirely in Java. It provides a rich set of APIs for creating and searching indexes, handling complex search queries, and scoring relevant search results. Lucene is widely used in applications such as e-commerce platforms, content management systems, and enterprise search solutions.
Efficient Indexing
Indexing is a crucial step in the search process, as it directly impacts the speed and accuracy of search queries. Here are some best practices for efficient indexing in Apache Lucene:
1. Use Batch Indexing
When dealing with large amounts of data, consider using batch indexing to improve indexing performance. This involves breaking down the indexing process into smaller batches, reducing memory overhead and optimizing disk I/O.
// Example of batch indexing in Lucene
IndexWriterConfig config = new IndexWriterConfig(analyzer);
config.setOpenMode(OpenMode.CREATE);
IndexWriter indexWriter = new IndexWriter(directory, config);
for (Document doc : documents) {
indexWriter.addDocument(doc);
}
indexWriter.commit();
indexWriter.close();
2. Optimize Index Structure
Lucene offers various configurations for index storage and structure. Choosing the appropriate index options, such as merge policy and index format, can significantly impact indexing performance and index size. Understanding the trade-offs between different index settings is crucial for optimization.
3. Monitor Memory Usage
Monitoring and controlling memory usage during the indexing process is essential to prevent out-of-memory errors and ensure smooth indexing performance. This involves managing memory allocation for caches, buffers, and document fields, especially when dealing with large datasets.
Enhanced Searching
Efficient searching is the hallmark of a high-performance search application. Here are some techniques to enhance searching performance in Apache Lucene:
1. Query Optimization
Crafting efficient and precise queries is paramount for fast search performance. Use optimized query parsers and analyzers to ensure that search queries are parsed and analyzed accurately, leading to relevant search results.
// Example of optimized query parsing in Lucene
QueryParser queryParser = new QueryParser("content", analyzer);
Query query = queryParser.parse("Apache Lucene");
2. Index Segmentation
Lucene organizes its indexes into segments for faster search operations. Monitoring and optimizing index segmentation, which involves merging and compacting index segments, can significantly improve search performance, especially in high-write scenarios.
3. Utilize Caching
Leverage query result caching and filter caching to store and reuse query results and filters, reducing the computation overhead for frequently occurring search queries and improving response times.
Scalability and Reliability
In addition to performance optimization, ensuring scalability and reliability of search applications is critical. Here are some practices to achieve this in Apache Lucene:
1. Distributed Search
For scalable search solutions, consider using Apache Solr, which is built on top of Lucene and provides distributed indexing and searching capabilities. Solr enables horizontal scaling, fault tolerance, and distributed search across multiple nodes.
2. Monitoring and Tuning
Regular monitoring of indexing and searching performance is crucial for identifying bottlenecks and potential issues. Utilize tools like Apache JMeter and Lucene/Solr performance analytics to measure and tune the performance of your search application.
3. Optimize Data Structures
Choosing the right data structures for indexing and searching, such as field types and data formats, can significantly impact the overall performance and reliability of the search application. Understanding the characteristics and usage patterns of the indexed data is essential for optimizing data structures.
To Wrap Things Up
Apache Lucene 5.0 provides a robust foundation for building high-performance search applications. By understanding and implementing efficient indexing, enhanced searching techniques, and scalability and reliability practices, you can optimize the performance and reliability of your search applications, delivering fast and accurate search results to your users.
Incorporating these best practices and techniques into your Apache Lucene-based search applications will not only improve the overall user experience but also ensure that your search infrastructure can handle increasing volumes of data and search queries with ease.
Remember, optimizing indexing and searching in Apache Lucene is an iterative process, and continuous monitoring, tuning, and adaptation to evolving requirements are essential for maintaining peak performance.
For further in-depth understanding of Lucene, check out the official Lucene documentation and Lucene indexing best practices.
Now, go ahead and optimize your Apache Lucene-based search applications for blazing-fast performance and reliability!