Calculating Time Differences with Java SE 8
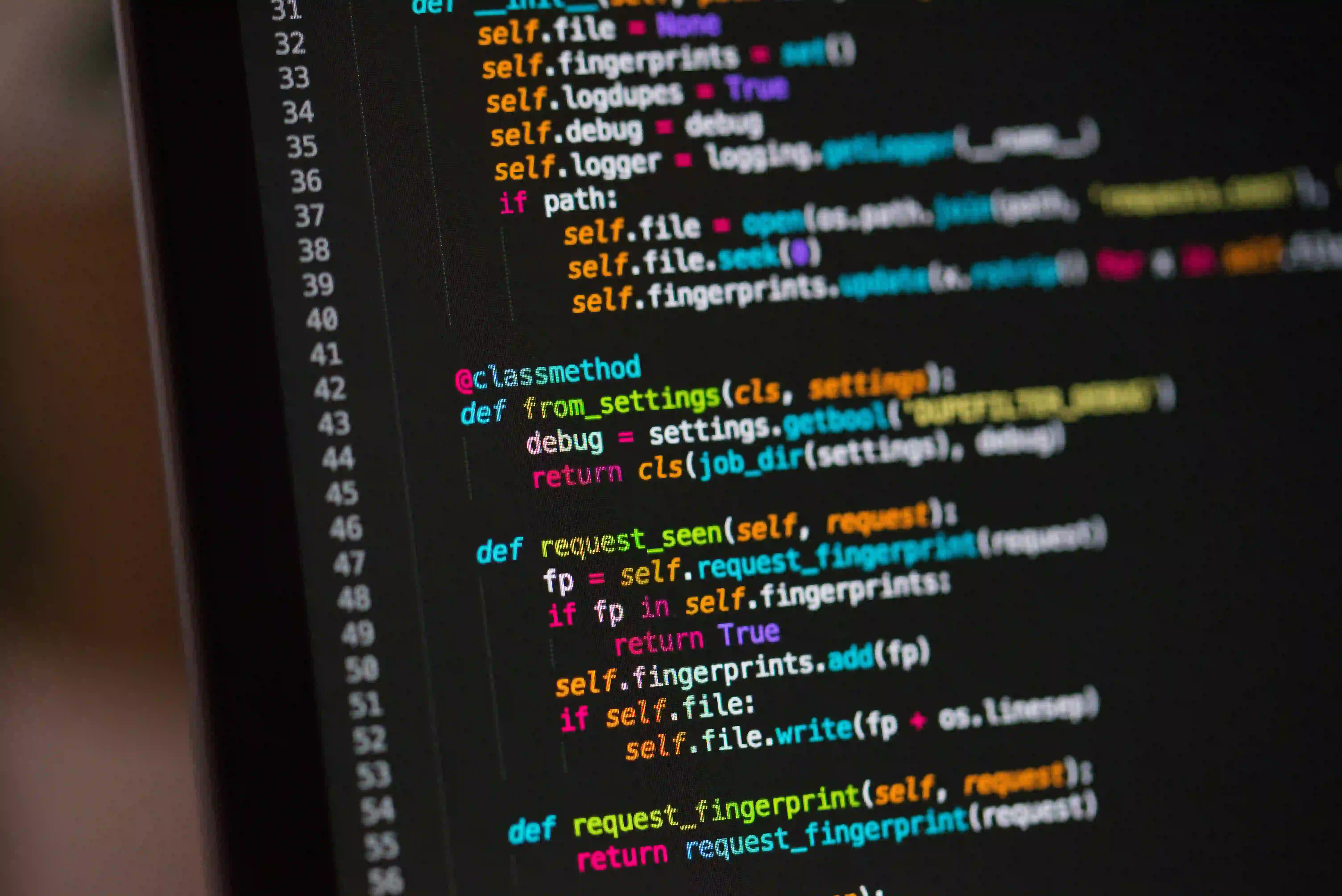
In the realm of software development, working with dates and time can be a complex and occasionally treacherous task. In Java, this can be particularly challenging due to its extensive set of date and time classes with their own intricacies. However, fear not! The introduction of the java.time package in Java SE 8 has dramatically simplified this process. In this post, we'll delve into how to calculate time differences using Java SE 8 and explore some common use cases and best practices.
The java.time Package
Prior to Java SE 8, the predominant date and time classes (java.util.Date and java.util.Calendar) were fraught with issues such as mutability, thread-safety problems, and a lack of human readability. Enter java.time, a new date and time API that addresses these shortcomings.
With the java.time package, the primary classes to be aware of are:
Instant
: Represents a point in time.LocalDate
: Represents a date without time information.LocalTime
: Represents a time without date information.LocalDateTime
: Represents both date and time without time zone information.ZonedDateTime
: Represents a date and time with time zone information.
Calculating Time Differences
Duration
The Duration
class is suitable for calculating time differences in terms of seconds and nanoseconds. Let's take a look at a basic example of using Duration
:
import java.time.Duration;
import java.time.LocalTime;
LocalTime start = LocalTime.of(9, 0);
LocalTime end = LocalTime.of(10, 30);
Duration duration = Duration.between(start, end);
System.out.println("Duration: " + duration);
In this example, we create two LocalTime
instances representing the start and end times, and then use the Duration.between
method to calculate the duration between them. The resulting duration
object can be used to obtain the duration in seconds and further manipulate it as needed.
Period
On the other hand, if you need to deal with date-based quantities (years, months, days), the Period
class is what you want. Consider the following example:
import java.time.LocalDate;
import java.time.Period;
LocalDate start = LocalDate.of(2022, 1, 1);
LocalDate end = LocalDate.of(2022, 12, 31);
Period period = Period.between(start, end);
System.out.println("Period: " + period);
Here, we use the Period.between
method to calculate the period between two LocalDate
instances. The resulting period
object can be used to obtain the period in years, months, and days.
Dealing with Time Zones
Time zone considerations can add another layer of complexity when dealing with time differences. The ZonedDateTime
class comes to the rescue, allowing you to work with date and time with time zone information. Let's explore an example:
import java.time.ZonedDateTime;
import java.time.ZoneId;
ZonedDateTime currentDateTime = ZonedDateTime.now(ZoneId.of("US/Pacific"));
ZonedDateTime futureDateTime = currentDateTime.plusHours(3).plusMinutes(30);
Duration duration = Duration.between(currentDateTime, futureDateTime);
System.out.println("Duration: " + duration);
In this snippet, we obtain the current date and time in the 'US/Pacific' time zone, then calculate a future date and time by adding 3 hours and 30 minutes. Finally, we calculate the duration between the two ZonedDateTime
instances.
Best Practices
When working with time differences in Java SE 8, it's essential to keep a few best practices in mind:
- Always consider time zone information when working with date and time.
- Prefer using the java.time package for new code, as the old date and time classes have been largely supplanted by it.
- Take care when using the
Duration
class for date arithmetic, as it's designed for smaller-scale time differences.
By adhering to these best practices, you can ensure that your time difference calculations are accurate and robust.
In Conclusion, Here is What Matters
In conclusion, Java SE 8's java.time package has greatly simplified the process of calculating time differences. By leveraging classes such as Duration
, Period
, and ZonedDateTime
, you can accurately handle time and date calculations with ease. Remember to pay careful attention to time zone information and embrace the modern java.time API for all your date and time needs. Happy coding!
So there you have it - a concise yet comprehensive guide to calculating time differences with Java SE 8. Whether you’re a seasoned Java developer or just starting out, understanding date and time calculations is crucial for building robust and accurate applications. If you're interested in delving deeper into Java SE 8 and its date and time capabilities, take a look at the official Java SE 8 Documentation.
Always keep in mind that precise and well-managed time calculations can make a critical difference in software systems, especially those involving financial transactions, scheduling, or any domain reliant on time intricacies. So, embrace the power of Java SE 8's java.time package and make time your ally in your next software project!