Managing Code Complexity with Default Methods
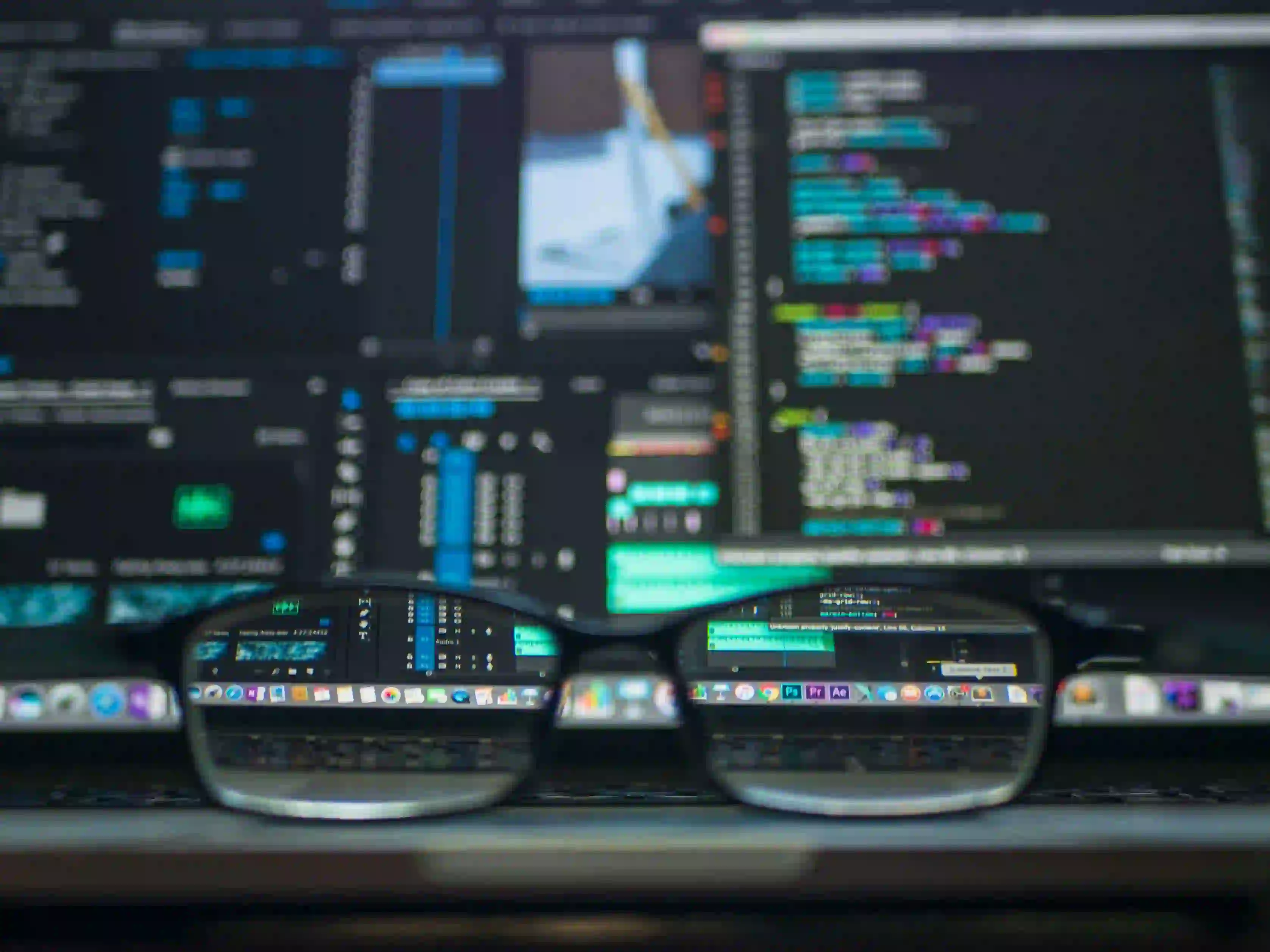
Managing Code Complexity with Default Methods
In Java, managing code complexity is crucial for maintaining a maintainable and scalable codebase. One way to achieve this is by using default methods. In this post, we'll explore how default methods can help in managing code complexity and improving the design of Java classes and interfaces.
What are Default Methods?
Introduced in Java 8, default methods allow interfaces to have method implementations. This feature was added to support backward compatibility and to extend interfaces without breaking existing implementations. Default methods provide a way to add new functionality to interfaces without affecting the classes that already use them.
Managing Code Complexity with Default Methods
1. Mixin-Like Functionality
Default methods enable mixin-like functionality in interfaces. This means that common behavior can be defined in an interface and reused across multiple classes without the need for copy-pasting code.
2. Interface Evolution
Default methods facilitate the evolution of interfaces. By adding new methods using default implementations, existing classes that implement the interface are not required to provide implementations for the new methods. This allows for seamless evolution of interfaces without breaking existing code.
3. Code Reusability
Default methods promote code reusability by allowing the sharing of method implementations across different classes. This reduces code duplication and promotes a more maintainable and modular codebase.
4. Reduction of Boilerplate Code
By providing default implementations for methods in an interface, default methods help reduce the amount of boilerplate code that would otherwise be required in implementing classes. This leads to cleaner and more concise code.
Examples of Default Methods
Let's take a look at a simple example to illustrate how default methods can be used to manage code complexity.
public interface Shape {
double area();
default void printDescription() {
System.out.println("This is a shape.");
}
}
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
}
public class Rectangle implements Shape {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
@Override
public double area() {
return length * width;
}
}
In this example, the Shape
interface defines a default method printDescription
that provides a default implementation for printing the description of a shape. The Circle
and Rectangle
classes implement the Shape
interface and provide their own implementations for the area
method while inheriting the printDescription
default method.
Why Use Default Methods?
1. Interface Extension
Default methods allow for the extension of interfaces without breaking the implementing classes. This is particularly useful when dealing with codebases where adding a new method to an interface might affect a large number of implementing classes.
2. Backward Compatibility
Default methods are designed to ensure backward compatibility. When new methods are added to an interface using default implementations, existing classes that use the interface are not required to provide implementations for the new methods.
3. Code Maintenance
By promoting code reusability and reducing boilerplate code, default methods contribute to better code maintenance. This leads to a more organized and manageable codebase.
The Bottom Line
In conclusion, default methods in Java offer a powerful mechanism for managing code complexity, promoting code reusability, and enabling interface evolution. By leveraging default methods, developers can design more flexible and extensible interfaces while minimizing the impact on implementing classes. When used appropriately, default methods contribute to a more maintainable and scalable codebase.
To delve deeper into the world of default methods, check out the official Oracle documentation on Interfaces and Java 8 Features.