Mastering Random Date Generation in Java: A Simple Guide
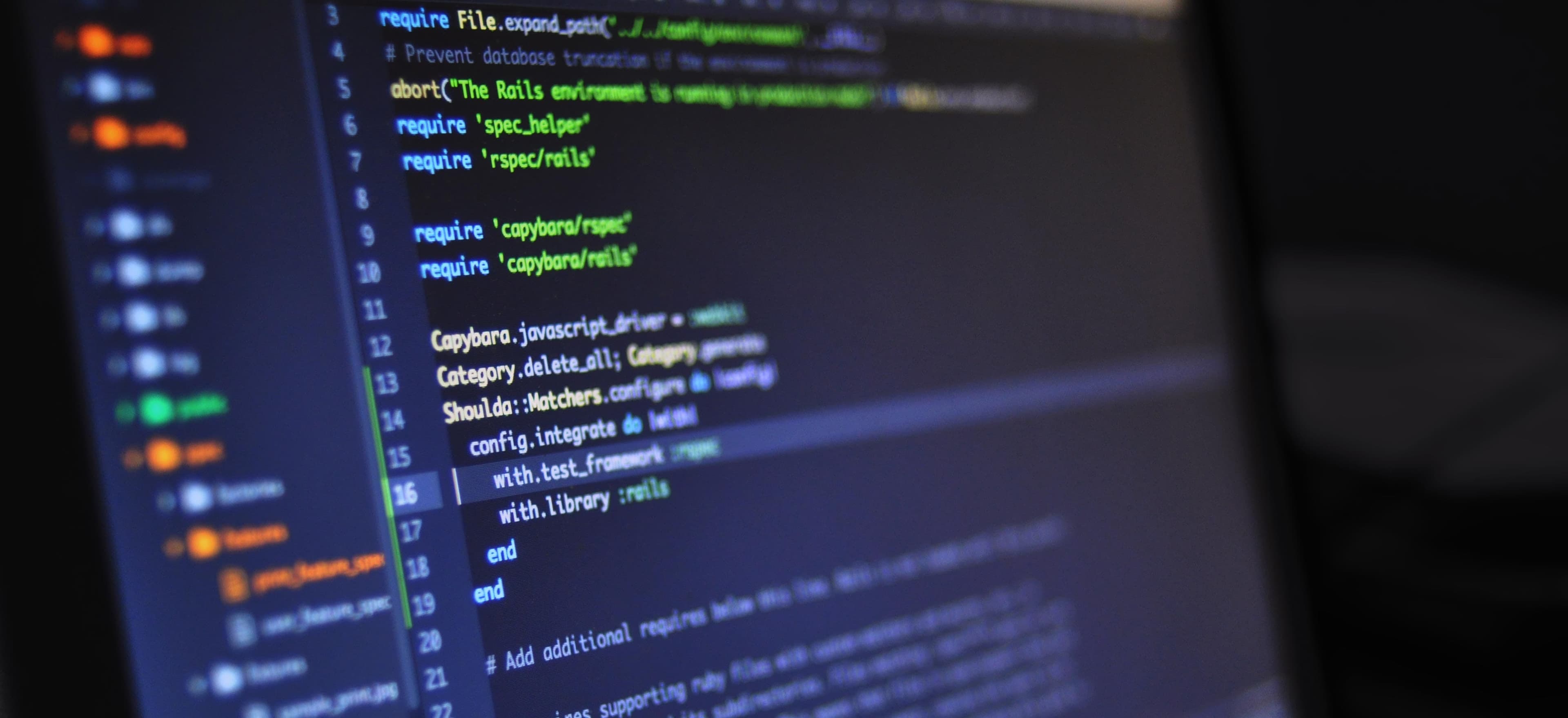
- Published on
Mastering Random Date Generation in Java: A Simple Guide
Generating random dates can prove to be a pivotal task in a variety of programming scenarios, such as testing, data generation, and simulation applications. In this guide, we’ll explore effective methods for generating random dates in Java, shedding light on the underlying mechanisms and potential use cases.
Why Generate Random Dates?
Generating random dates can aid in creating realistic datasets for testing purposes or simulating real-world scenarios. Developers often find themselves needing random data to mimic user activity, analyze behaviors, or even stress-test systems.
In the context of one existing article titled "Generating Random Dates: Overcoming Time Constraints" found at tech-snags.com/articles/generating-random-dates-time-constraints, the focus is on overcoming specific limitations when handling date and time.
This article will build on these principles to give you a comprehensive understanding of creating random dates in Java.
Getting Started with Java Date and Time API
Java provides several classes for handling dates and times. The most modern and flexible option is provided by the java.time
package, introduced with Java 8.
Here’s a simple overview of essential classes:
LocalDate
: Represents a date without time-zone; suitable for date-only calculations.LocalDateTime
: Represents date-time without a time-zone; ideal when you need both date and time.ZonedDateTime
: Contains a date-time with a time-zone.
To generate a random date, we can primarily utilize the LocalDate
class since we often need just the date for general use cases.
Basic Random Date Generation
First, let's look at a straightforward example of generating a random LocalDate
within a specific range.
import java.time.LocalDate;
import java.time.temporal.ChronoUnit;
import java.util.Random;
public class RandomDateGenerator {
private static final Random random = new Random();
public static LocalDate generateRandomDate(LocalDate startDate, LocalDate endDate) {
long daysBetween = ChronoUnit.DAYS.between(startDate, endDate);
long randomDays = random.nextInt((int) daysBetween + 1); // +1 to include end date
return startDate.plusDays(randomDays);
}
public static void main(String[] args) {
LocalDate startDate = LocalDate.of(2020, 1, 1);
LocalDate endDate = LocalDate.of(2023, 12, 31);
LocalDate randomDate = generateRandomDate(startDate, endDate);
System.out.println("Generated Random Date: " + randomDate);
}
}
Breakdown of the Code:
-
ChronoUnit: The
ChronoUnit.DAYS.between(startDate, endDate)
calculates the number of days between the specified dates. -
Random Index: The
random.nextInt((int) daysBetween + 1)
generates a random index that effectively picks a day from the range. -
Final Date: The
startDate.plusDays(randomDays)
function finalizes the random date selected, maintaining clarity and precision.
Extending Functionality
Generating Random DateTimes
If your application requires generating a date and time, the next step would be to incorporate LocalDateTime
. Here's an example:
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.time.format.DateTimeFormatter;
import java.util.Random;
public class RandomDateTimeGenerator {
private static final Random random = new Random();
public static LocalDateTime generateRandomDateTime(LocalDateTime startDateTime, LocalDateTime endDateTime) {
long secondsBetween = java.time.Duration.between(startDateTime, endDateTime).getSeconds();
long randomSeconds = random.nextInt((int) secondsBetween + 1);
return startDateTime.plusSeconds(randomSeconds);
}
public static void main(String[] args) {
LocalDateTime startDateTime = LocalDateTime.of(2020, 1, 1, 0, 0);
LocalDateTime endDateTime = LocalDateTime.of(2023, 12, 31, 23, 59);
LocalDateTime randomDateTime = generateRandomDateTime(startDateTime, endDateTime);
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
System.out.println("Generated Random DateTime: " + randomDateTime.format(formatter));
}
}
Explanation of the New Elements
-
LocalDateTime Creation: We specify both dates and times with
LocalDateTime.of
to create start and end times. -
Duration Between Dates: Here, we switch to
Duration.between(...).getSeconds()
, enabling us to work with all time units (seconds). -
Formatted Output: The
DateTimeFormatter
allows for better presentation of the generated datetime, essential for user-facing applications.
Potential Applications for Random Date Generation
Here are a few scenarios where generating random dates can be beneficial:
-
Test Data Generation: Random dates allow developers to populate databases with realistic data for testing purposes.
-
Event Simulation: In a simulation context, random dates can help model real-world events (e.g., user sign-ups, purchases around holiday seasons).
-
Data Analysis: Analysts often require datasets with temporal aspects to gauge trends, behaviors, and seasonality; random dates can augment existing data.
Best Practices for Random Date Generation
While random date generation can be straightforward, certain best practices maximally enhance its effectiveness:
1. Define Clear Parameters
Always define the appropriate start and end dates based on the specific context of use. For instance, if conducting analysis on current trends, your date range would differ than testing older historical data.
2. Consider Edge Cases
Account for scenarios like leap years or daylight saving changes if the generation involves specific dates related to seasonal behaviors.
3. Validating Generated Dates
Implement validation checks to ensure the generated dates are within the desired parameters.
4. Utilizing Libraries
For complex applications, consider libraries like Apache Commons Lang that enhance date utilities.
Final Considerations
Generating random dates in Java is both practical and straightforward. Whether you require a quick solution for testing or more detailed simulations, understanding Java's date and time classes helps you fulfill your application needs effectively.
By mastering the techniques discussed, you'll be well-equipped to generate realistic datasets that mimic user behaviors and outcomes in your development projects.
If you're interested in overcoming time constraints in date generation, don't forget to check the detailed guide on that topic at tech-snags.com/articles/generating-random-dates-time-constraints.
Happy coding!
Checkout our other articles