Enhancing Java Applications with Natural Language Processing
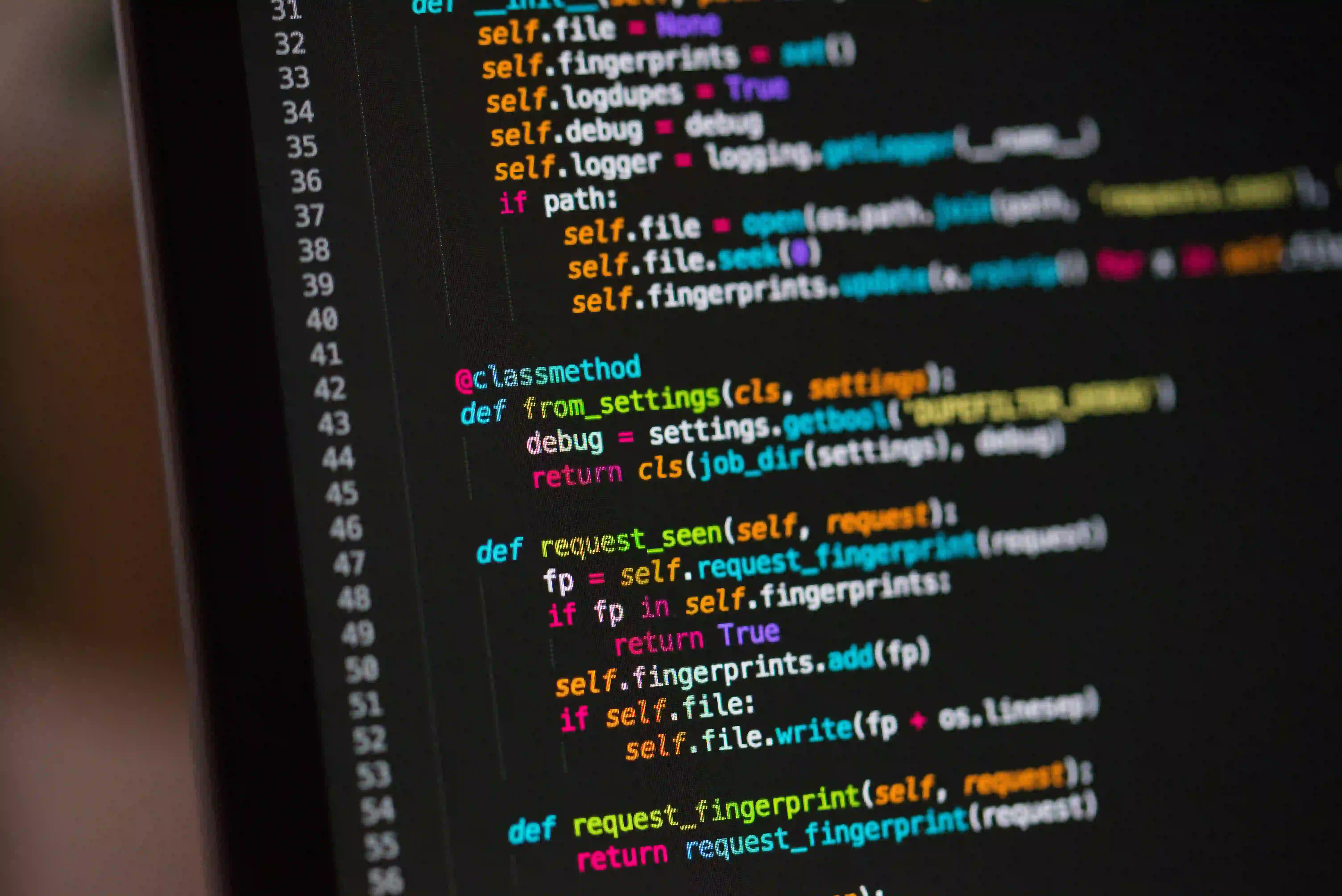
Enhancing Java Applications with Natural Language Processing
Natural Language Processing (NLP) is a cutting-edge technology that allows machines to understand and interpret human language. With the evolution of Java and a plethora of libraries available, developers can now easy integrate NLP capabilities into their applications. In this article, we will explore how to enhance Java applications with NLP, examining key libraries, providing examples, and discussing best practices.
What is Natural Language Processing?
NLP combines computational linguistics and machine learning to enable computers to process and analyze large amounts of natural language data. This technology allows Java applications to perform tasks such as sentiment analysis, text classification, entity recognition, and more.
Key Benefits of NLP in Java Applications:
- Improved User Experience: By understanding and responding to user inputs more naturally.
- Data Insights: Extracting valuable insights from unstructured text data.
- Automation: Streamlining tasks like document review or customer service interactions.
Popular Java Libraries for NLP
Several libraries facilitate the integration of NLP features in Java applications. Some of the most popular ones include:
- Apache OpenNLP
- Stanford NLP
- spaCy (via Java API)
- LingPipe
- Java NLP (Javanlp)
In this article, we will focus on Apache OpenNLP and Stanford NLP, as they are widely adopted and offer comprehensive features.
Apache OpenNLP
Apache OpenNLP is a machine learning-based toolkit for processing natural language text. It offers functionalities for tasks such as tokenization, sentence splitting, part-of-speech tagging, named entity recognition, and more.
Example: Tokenization with OpenNLP
import opennlp.tools.tokenize.SimpleTokenizer;
public class TokenizationExample {
public static void main(String[] args) {
String input = "Natural Language Processing enhances Java applications.";
// Create an instance of the SimpleTokenizer
SimpleTokenizer tokenizer = SimpleTokenizer.INSTANCE;
// Tokenize the input text
String[] tokens = tokenizer.tokenize(input);
System.out.println("Tokens:");
for (String token : tokens) {
System.out.println(token);
}
}
}
Why this code matters: Tokenization is the first step in many NLP tasks. It splits text into individual tokens, making it easier to analyze and process. By utilizing Apache OpenNLP, you gain efficiency and accuracy in parsing human language.
Stanford NLP
Stanford NLP provides a rich set of NLP tools and models. Its Java-based library is known for supporting various NLP tasks, including dependency parsing, sentiment analysis, and coreference resolution.
Example: Sentiment Analysis with Stanford NLP
import edu.stanford.nlp.pipeline.*;
import java.util.Properties;
public class SentimentAnalysisExample {
public static void main(String[] args) {
// Set up the Stanford NLP pipeline
Properties props = new Properties();
props.setProperty("annotators", "sentiment");
props.setProperty("outputFormat", "text");
StanfordCoreNLP pipeline = new StanfordCoreNLP(props);
// Input text for sentiment analysis
String text = "I absolutely love using Java for Natural Language Processing!";
// Create an Annotation object
Annotation annotation = new Annotation(text);
pipeline.annotate(annotation);
// Display sentiment analysis results
System.out.println("Sentiment: " + annotation.get(CoreAnnotations.SentimentClass.class));
}
}
Why this code is relevant: The ability to analyze sentiment provides significant insights into user feedback or social media interactions. This example illustrates how easy it is to integrate complaint analysis within your Java applications using Stanford NLP.
Real-World Applications of NLP in Java
NLP has a wide variety of applications. Some common use cases include:
- Chatbots: Automating customer support using NLP-powered chatbots can significantly lower response time and increase customer satisfaction.
- Content Recommendation Systems: Analyzing user preferences through text data can help tailor content to enhance user engagement.
- Sentiment Analysis in Reviews: Analyze sentiment from product reviews on e-commerce platforms, helping businesses improve their products.
You can explore the nuances of how NLP enhances user experiences by referencing related topics such as the article on "Gewürznelken: Kleine Kostbarkeiten mit großer Wirkung" (accessible via auraglyphs.com). Just as the article discusses the significant benefits of a spice, NLP enriches Java applications, enabling them to perform complex language tasks.
Best Practices for Using NLP in Java
While integrating NLP into your Java applications is beneficial, it's essential to follow best practices:
- Choose the Right Library: Select a library that aligns with your project's requirements. Consider factors like language support, community engagement, and ease of use.
- Optimize Performance: NLP tasks can be resource-intensive. Employ performance optimizations, such as using multi-threading or caching results.
- Use Pre-trained Models: Many libraries provide pre-trained models that can save time. Fine-tune these models according to your application's specific needs.
- Continuously Train Your Models: Keep evaluating and retraining your models to adapt to the changing language and user preferences. This is vital for maintaining accuracy.
The Closing Argument
Incorporating NLP into Java applications opens up a world of possibilities—from enriching user interactions to enhancing data processing capabilities. With libraries like Apache OpenNLP and Stanford NLP, developers can easily implement complex language features. Emphasizing best practices will ensure that the integration works effectively and yields the best outcomes.
As technology continues to evolve, so will the potential for NLP within Java applications. Dive into this exciting field, and transform the way users interact with your applications. For more insights on related topics, be sure to check additional resources, like the one linked earlier on "Gewürznelken: Kleine Kostbarkeiten mit großer Wirkung" (auraglyphs.com). Happy coding!