Mastering Date Manipulation in Java for Dynamic Solutions
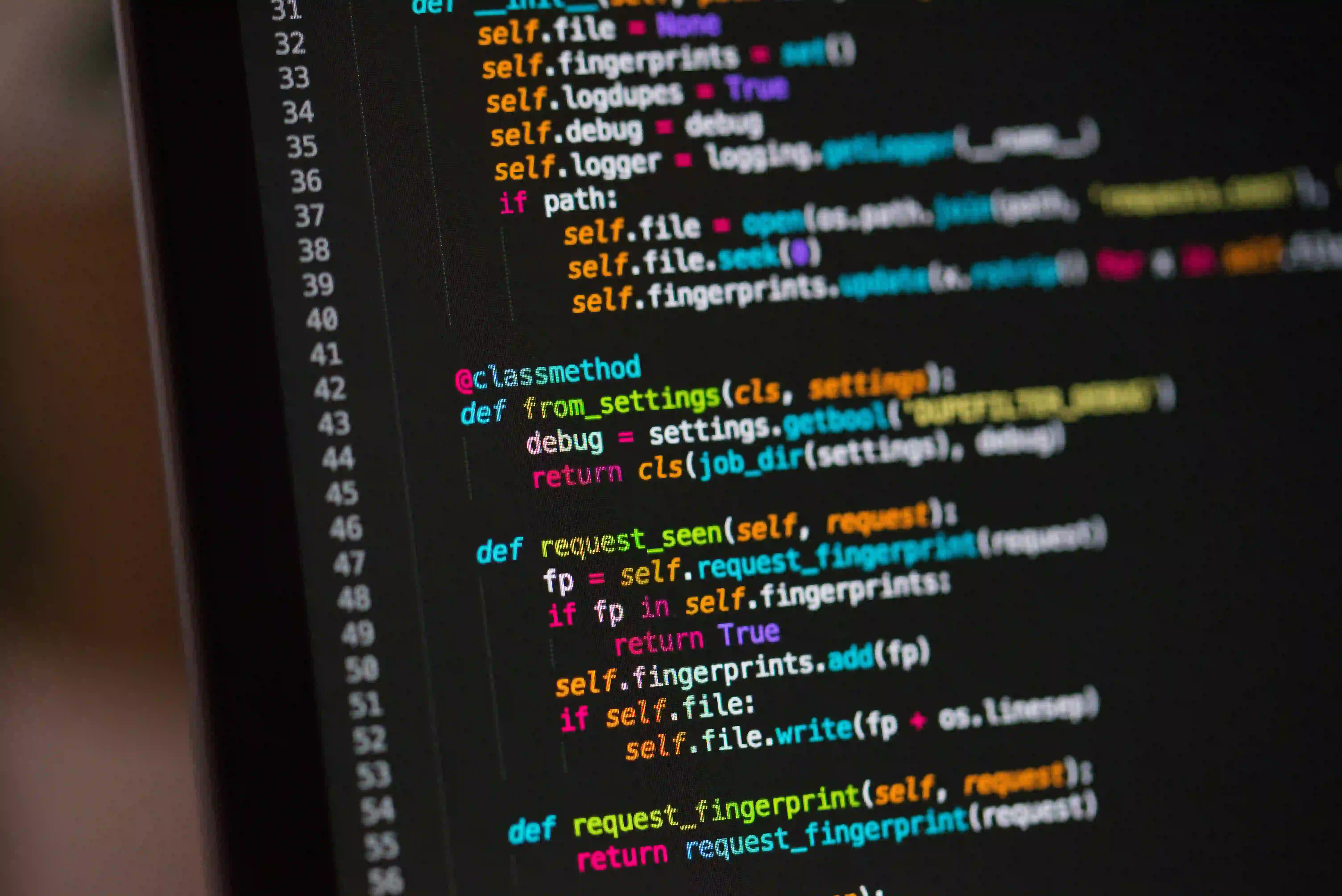
Mastering Date Manipulation in Java for Dynamic Solutions
Date manipulation is a crucial aspect of software development, particularly when working on applications that require dynamic data handling, such as event scheduling, logging, and temporal queries. Java offers a robust set of libraries to help developers navigate these complexities efficiently. In this blog post, we will delve into various techniques for manipulating dates in Java, provide practical examples, and discuss why these methods are essential in real-world applications.
Understanding Java Date Libraries
Java has undergone significant changes in date and time handling since the introduction of the java.time
package in Java 8. This package provides a more comprehensive framework for date and time manipulation compared to its predecessor, java.util.Date
. For instance:
- Improved API: The new API is immutable, thread-safe, and more expressive.
- Better handling of time zones: The API allows easy manipulation of time zones with classes like
ZonedDateTime
.
These improvements enable developers to create dynamic applications that can handle various date-related requirements effortlessly.
Getting Started
Importing Necessary Packages
To use the new date and time API, you need to import the relevant classes at the beginning of your Java program:
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
These classes allow you to create, manipulate, and format dates and times in different scenarios.
Creating Date Instances
Creating date instances in Java has never been easier. Here’s how you can create different types of date objects:
LocalDate today = LocalDate.now(); // Current date
LocalDateTime now = LocalDateTime.now(); // Current date and time
ZonedDateTime zoned = ZonedDateTime.now(); // Current date and time with timezone
System.out.println("Today: " + today);
System.out.println("Current DateTime: " + now);
System.out.println("Zoned DateTime: " + zoned);
Why Use These Classes?
Using LocalDate
, LocalDateTime
, and ZonedDateTime
makes your code cleaner and less error-prone. These classes eliminate ambiguity associated with time zones and daylight saving time, ensuring your applications behave predictably across different geographical locations.
Parsing and Formatting Dates
Another key area in date manipulation is parsing and formatting. Java provides the DateTimeFormatter
class for this purpose.
Example: Parsing a String to LocalDate
Suppose we have a date string that we want to convert to a LocalDate
object:
String dateStr = "2023-10-25";
LocalDate parsedDate = LocalDate.parse(dateStr);
System.out.println("Parsed Date: " + parsedDate);
Formatting Dates for Display
You might often want to display dates in a specific format. Here is how you can format a LocalDate
using DateTimeFormatter
:
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy");
String formattedDate = today.format(formatter);
System.out.println("Formatted Today: " + formattedDate);
The Importance of Formatting
Properly formatted dates enhance the user experience. Displaying dates in a way that's familiar to your audience reduces cognitive load and increases usability.
Adding and Subtracting Dates
Manipulating dates by adding or subtracting a specific period is a common requirement. The LocalDate
and LocalDateTime
classes offer several methods to handle this effectively.
Example: Adding Days
LocalDate tenDaysLater = today.plusDays(10);
System.out.println("Ten Days Later: " + tenDaysLater);
Example: Subtracting Months
LocalDate lastMonth = today.minusMonths(1);
System.out.println("Last Month: " + lastMonth);
Why Manipulate Dates?
Being able to dynamically add or subtract dates is critical in business applications that rely on deadlines, expirations, or recurring events. This flexibility allows you to build intelligent systems that can manage tasks effectively.
Handling Time Zones
Time zones can complicate date and time manipulation significantly. The ZonedDateTime
class is specifically designed to tackle these challenges.
Example: Storing and Displaying Time Zones
ZonedDateTime newYorkTime = ZonedDateTime.now(ZoneId.of("America/New_York"));
ZonedDateTime londonTime = ZonedDateTime.now(ZoneId.of("Europe/London"));
System.out.println("New York Time: " + newYorkTime);
System.out.println("London Time: " + londonTime);
Why Use ZonedDateTime?
For applications intended for a global audience, handling time zones correctly ensures that users see the correct date and time for their location. This aspect is vital for systems that rely on event scheduling or user-generated timestamps.
Real-world Application and Integration
An excellent example of date manipulation in action is generating random dates. This feature can be crucial for testing and simulating data in applications. If you're interested in generating random dates, I recommend checking out our article titled Generating Random Dates: Overcoming Time Constraints, where we explore various strategies for managing time constraints.
Final Considerations
Mastering date manipulation in Java opens up countless opportunities for building dynamic applications. From creating and formatting dates to adding and subtracting time periods, the Java java.time
package provides powerful tools for developers. By understanding how to leverage these tools effectively, you can create applications that are not only responsive but also intuitive.
Date manipulation may seem trivial at first glance, but the attention to detail you apply can impact user experience significantly. As you continue your Java programming journey, keep these principles in mind to streamline the date-handling process in your applications. Whether you are creating a scheduling app or a simple timer, the right techniques can make all the difference.