Mastering Linux Commands to Enhance Java Application Performance
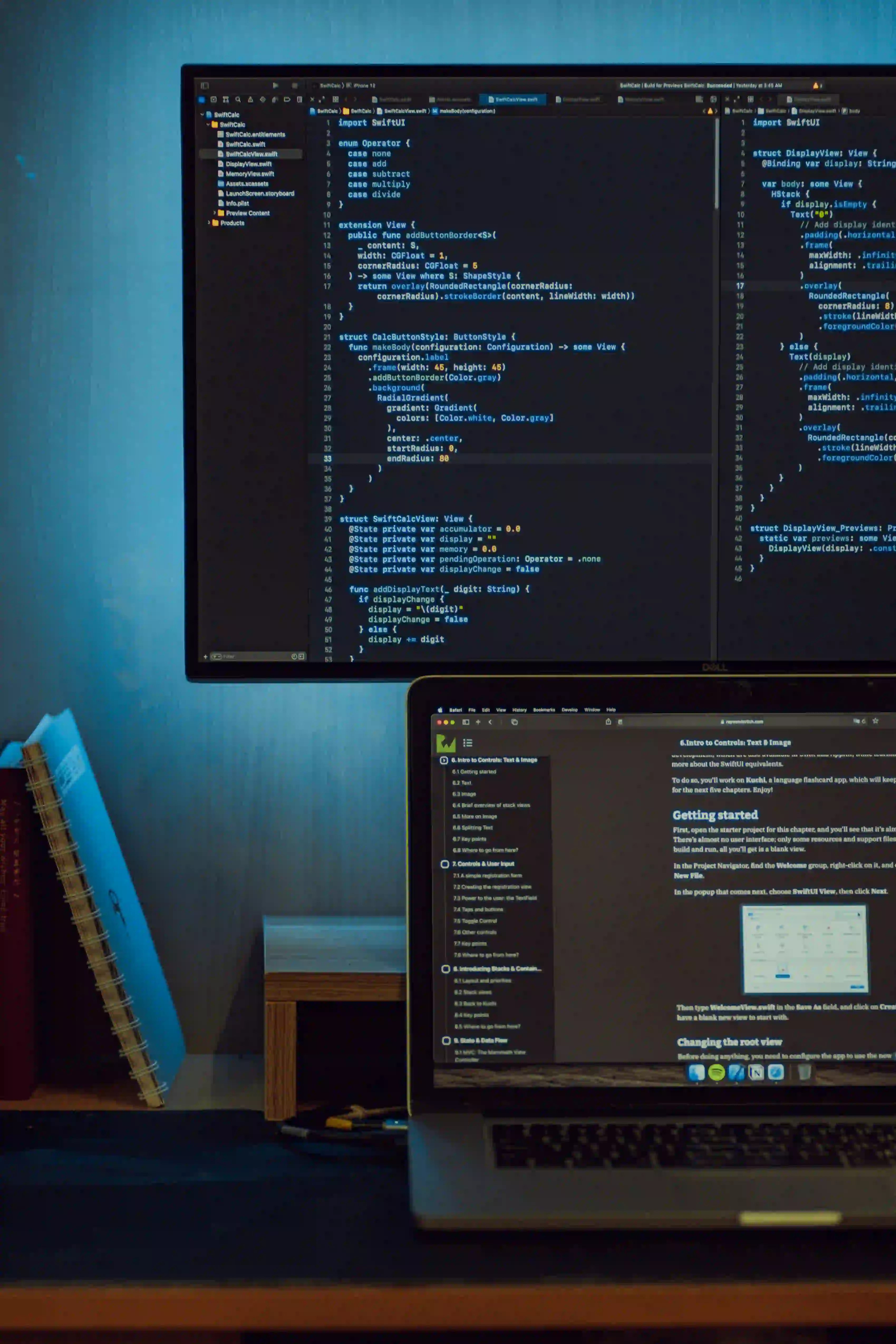
Mastering Linux Commands to Enhance Java Application Performance
In the world of software development, performance is paramount. Java developers often seek ways to optimize their applications, but many overlook the importance of the underlying operating system—in this case, Linux. Mastering Linux commands can significantly enhance Java application performance and streamline development workflows.
In this article, we'll explore how pairs of Linux commands and Java performance best practices overlap. We'll provide concrete code snippets and scenarios, along with a reference to an existing resource, "Top 10 Linux Commands for Aspiring Data Engineers".
Why Linux for Java?
Before we dive into specific commands, let's talk about why Linux is ideal for Java development:
- Stability: Linux is known for its stability and is widely used in production environments.
- Flexibility: Many Java server applications run on Linux due to its open-source nature.
- Performance Tools: Linux provides many built-in tools that can help monitor and optimize Java applications.
Now, let's explore some powerful Linux commands relevant to Java performance.
1. Monitor System Resources with top
and htop
Both top
and htop
are tools that allow you to monitor the processes and resource usage of your system. Using these tools, you can watch your Java application's CPU and memory usage in real-time.
Top
The top
command provides real-time updates and interfaces. You can identify memory-consuming processes and optimize them.
top
- Why: This command shows the Java process and helps identify high CPU usage, memory leaks, or bottlenecks.
Htop
htop
is an upgraded version of top
, offering a more user-friendly interface. You may need to install it first:
sudo apt-get install htop
Then run:
htop
- Why: Compared to
top
,htop
provides color-coded outputs and easier navigation, making it simpler to identify issues.
2. Check Disk Usage with df
and du
When your Java application runs low on space, performance can decline. The df
command displays disk space usage, while du
shows the size of directories.
Check Disk Usage with df
You can quickly check the disk space using:
df -h
- Why: Using the
-h
flag gives you a human-readable format, making it easy to see available space.
Check Directory Size with du
To check the size of a specific directory:
du -sh /path/to/your/java/app
- Why: The
-s
flag gives a summary and the-h
provides human-readable sizes. This insight helps identify large files or directories needing cleanup.
3. View Log Files Using tail
Logs can provide extensive insight into application performance. In Java applications, you might want to monitor logs continuously.
tail -f /var/log/java-application.log
- Why: The
-f
option follows the log in real time, enabling you to catch errors and performance issues as they occur.
4. Kill High CPU Processes with kill
and pkill
Occasionally, a Java process may consume excessive CPU resources. You can terminate it using the kill
or pkill
commands.
Kill a Process by PID
To kill a specific process:
kill <PID>
- Why: Replace
<PID>
with the process ID. This command is often appropriate when you want to terminate a specific instance of your Java application.
Kill a Process by Name
To kill the Java process by name:
pkill -f java
- Why: This command is straightforward, terminating all Java processes, which is useful in development and testing.
5. Network Performance with netstat
and ping
Applications often depend on network performance. You can monitor network connections using netstat
, and check connectivity with ping
.
Check Active Connections
netstat -tulnp
- Why: This command will show which ports your Java application is using and their respective states. Identifying bottlenecks can help you isolate network-related issues.
Check Connectivity
ping <hostname>
- Why: Replace
<hostname>
with your server or database. Aping
can help ensure that your application can connect to necessary services without latency.
6. Disk I/O Monitoring with iostat
Monitoring I/O operations is crucial for performance tuning. The iostat
command can provide detailed insights.
sudo apt-get install sysstat
iostat -m 1
- Why: This command will provide metrics on CPU utilization and I/O operations, with
-m
showing stats in megabytes. Use this to identify disk bottlenecks that could slow down your Java applications.
7. Profile Your Java Application with jcmd
To understand Java application performance better, you may want to profile it using the jcmd
tool that comes with the JDK.
First, identify the Java process ID (PID):
jps
Then run the following command:
jcmd <PID> GC.high_freq
- Why: This invokes a high-frequency garbage collection, allowing you to analyze memory management more effectively.
8. File Searching with find
To locate files quickly, the find
command is immensely valuable, particularly in large projects.
find . -name "*.java"
- Why: This command searches for all Java files in the current directory. Finding specific files can boost productivity during application refactoring.
Final Considerations
Understanding Linux commands can greatly improve your Java application performance by providing tools to monitor resources, troubleshoot issues, and streamline development processes.
To dive deeper, check out Top 10 Linux Commands for Aspiring Data Engineers, which goes further into essential commands every developer should know.
By mastering these commands and their applications, you can ensure that your Java applications run not only smoothly but also efficiently, thereby providing a better experience for users and stakeholders alike. So fire up your terminal, and get ready to enhance your Java performance game!