Troubleshooting RabbitMQ: Java Framework Installation Woes
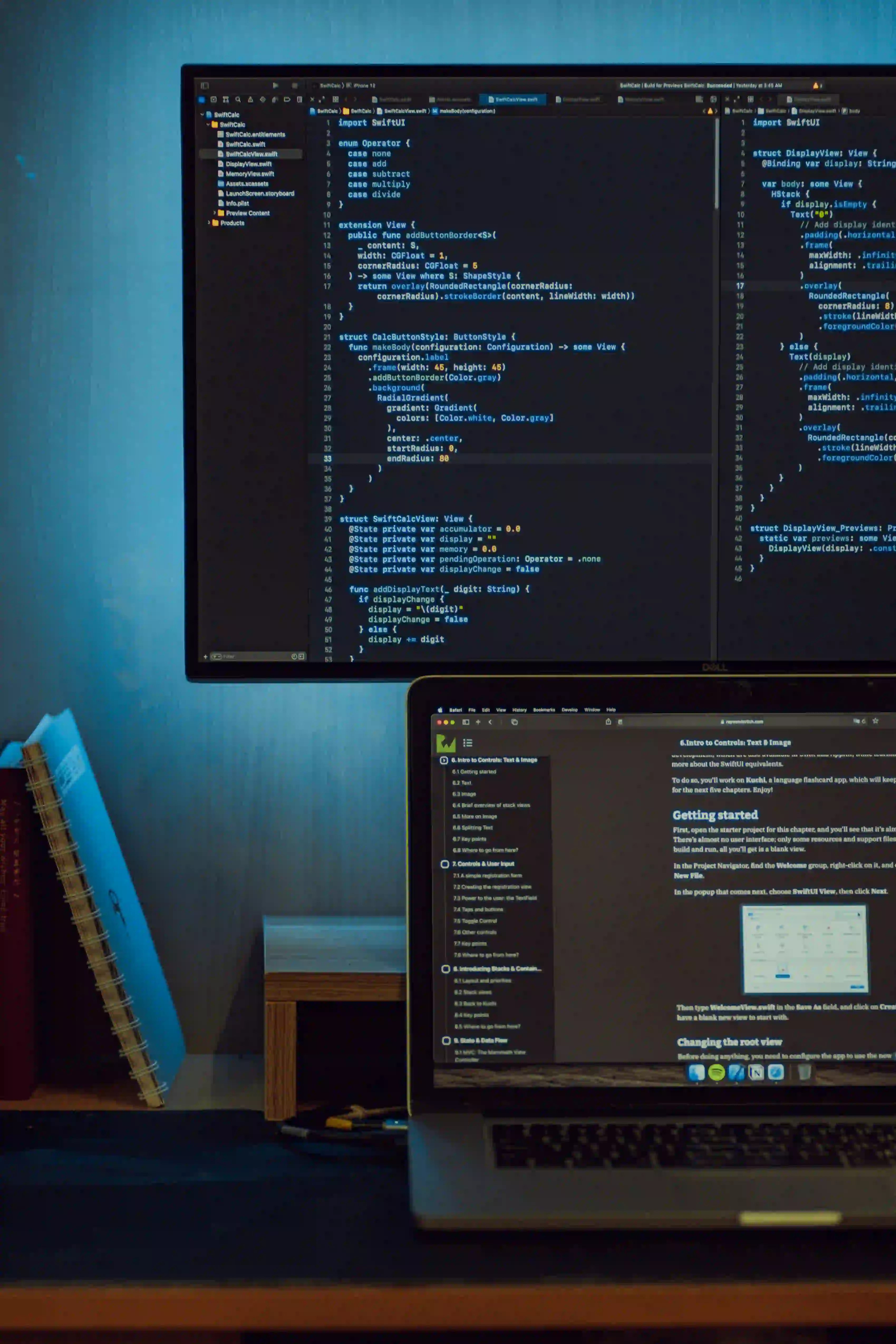
Troubleshooting RabbitMQ: Java Framework Installation Woes
As a Java developer, dealing with message brokers like RabbitMQ can significantly enhance your application's ability to scale and manage asynchronous tasks. However, just like any other technology, integrating RabbitMQ into your Java project can sometimes lead to challenges and installation issues. This blog post will walk you through common hurdles while integrating RabbitMQ with Java, troubleshoot these problems, and look at how to resolve them effectively.
Understanding RabbitMQ and Java Integration
RabbitMQ is an open-source message broker that facilitates communication between your applications via messages. It supports various messaging protocols such as AMQP, MQTT, and STOMP, making it versatile for different project requirements. Java developers commonly use RabbitMQ for building scalable and resilient microservices architectures.
In this post, we’ll discuss the installation issues you might encounter while setting up RabbitMQ in a Java environment and provide solutions to switch from frustration to seamless integration.
Why Use RabbitMQ in Java?
- Decoupling: RabbitMQ allows separate components of your application to communicate without being tightly coupled.
- Asynchronous Processing: You can push tasks to a queue and let worker threads process them asynchronously, which enhances performance.
- Load Balancing: RabbitMQ can distribute workloads evenly across multiple consumers, improving resource utilization.
- Reliability: With features like message acknowledgment and persistence, RabbitMQ ensures secure message delivery.
Common Installation Issues
Before diving into troubleshooting, let’s briefly touch on common installation issues developers may face:
- Incompatible versions of Java and RabbitMQ.
- Configuration problems within the RabbitMQ server.
- Missing Java libraries such as amqp-client.
- Firewall and network issues interfering with RabbitMQ connections.
For those specifically using Rocky Linux, I would highly recommend referring to the article “Common Installation Issues for RabbitMQ on Rocky Linux” available at configzen.com for more insights into platform-specific concerns.
Pre-Installation Checks
Before proceeding with installation, ensure you have the following prerequisites in place:
- Java Installed: Ensure Java 8 or higher is installed and properly configured.
- RabbitMQ Server: Download the server from the official RabbitMQ website.
- Permissions: Run the installation under user permissions with the necessary rights to avoid permission-related issues.
You can verify Java installation using:
java -version
Step-by-Step RabbitMQ Installation
- Download RabbitMQ Server
Visit the RabbitMQ downloads page to find the correct package for your operating system, or use:
sudo yum install rabbitmq-server
- Install Dependencies
Make sure that Erlang is also installed as RabbitMQ relies on it:
sudo yum install epel-release
sudo yum install erlang
- Start RabbitMQ
After installation, use the following command to start RabbitMQ:
sudo systemctl start rabbitmq-server
- Enable RabbitMQ Service
To ensure RabbitMQ starts on boot:
sudo systemctl enable rabbitmq-server
- Configure RabbitMQ
You can configure RabbitMQ using the rabbitmq.config
file usually located under /etc/rabbitmq/
.
Example configuration file:
[
{rabbit, [
{loopback_users, []}, % Allow non-local connections
{listeners, [{clustering, 25672}, {amqp, 5672}]}
]}
].
This customized configuration allows external connections and configures the default listener ports.
Integrating RabbitMQ with Java
Now that RabbitMQ is installed, it's time to integrate it into a Java application. The following steps illustrate creating a simple producer-consumer setup.
Add Required Dependencies
For Maven users, add the RabbitMQ Java client dependency to your pom.xml
:
<dependency>
<groupId>com.rabbitmq</groupId>
<artifactId>amqp-client</artifactId>
<version>5.14.0</version>
</dependency>
Producer Code Example
Here’s a simple producer code snippet that sends messages to RabbitMQ:
import com.rabbitmq.client.ConnectionFactory;
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.Channel;
public class Producer {
private final static String QUEUE_NAME = "hello";
public static void main(String[] argv) throws Exception {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost"); // Target RabbitMQ server
try (Connection connection = factory.newConnection();
Channel channel = connection.createChannel()) {
channel.queueDeclare(QUEUE_NAME, false, false, false, null);
String message = "Hello World!";
// Send the message to the queue
channel.basicPublish("", QUEUE_NAME, null, message.getBytes("UTF-8"));
System.out.println(" [x] Sent '" + message + "'");
}
}
}
Why This Works:
- The
ConnectionFactory
creates a connection to the RabbitMQ server. - Using channels allows to declare a queue and send messages efficiently.
Consumer Code Example
And here’s how a consumer might look:
import com.rabbitmq.client.*;
public class Consumer {
private final static String QUEUE_NAME = "hello";
public static void main(String[] argv) throws Exception {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
try (Connection connection = factory.newConnection();
Channel channel = connection.createChannel()) {
channel.queueDeclare(QUEUE_NAME, false, false, false, null);
System.out.println(" [*] Waiting for messages. To exit press CTRL+C");
DeliverCallback deliverCallback = (consumerTag, delivery) -> {
String message = new String(delivery.getBody(), "UTF-8");
System.out.println(" [x] Received '" + message + "'");
};
channel.basicConsume(QUEUE_NAME, true, deliverCallback, consumerTag -> { });
}
}
}
Why This Works:
- The consumer waits for messages on the declared queue.
- It processes messages through a callback when they arrive, allowing real-time response functionalities.
Troubleshooting Common Issues
-
Connection Refused: If you encounter a "Connection refused" issue, ensure RabbitMQ is running. Use:
🔧snippet.shsudo systemctl status rabbitmq-server
If it’s not active, start it with
sudo systemctl start rabbitmq-server
. -
Authorization Errors: If you see authorization errors, review your RabbitMQ configuration to ensure user permissions are properly set.
-
Firewall Settings: Rules on your firewall might block RabbitMQ traffic. Confirm that port 5672 (AMQP) is open:
🔧snippet.shsudo iptables -L | grep 5672
Final Considerations
Integrating RabbitMQ into a Java application can yield tremendous benefits, including improved communication and performance management. However, you may encounter various installation issues throughout the process, especially when using a specific OS such as Rocky Linux.
To smooth your setup, be aware of common difficulties and follow the steps described above. For more information, consider checking out the earlier mentioned article, "Common Installation Issues for RabbitMQ on Rocky Linux," to equip yourself with best practices.
Understanding how to effectively manage and troubleshoot your RabbitMQ installation is crucial for building resilient Java applications. By adhering to the recommendations outlined in this post, you will have the tools to navigate any installation woes and leverage RabbitMQ to serve your application needs optimally.
If you have any other patterns or experiences with RabbitMQ that weren't covered, feel free to share in the comments. Happy coding!