Boosting Java Frameworks: Tackling Originality Challenges
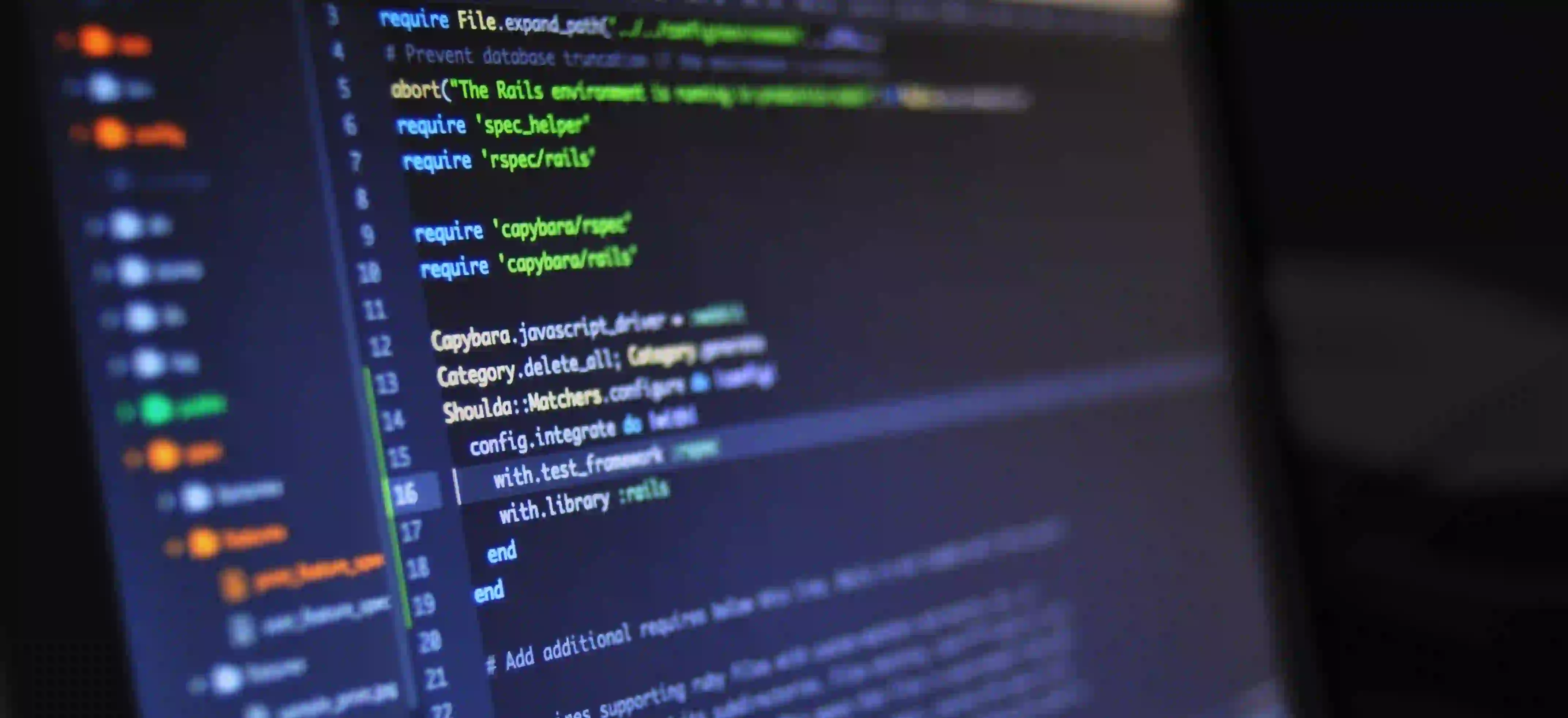
Boosting Java Frameworks: Tackling Originality Challenges
In the ever-evolving world of web development, originality stands as a cornerstone for creativity and innovation. However, developers often face challenges in creating unique solutions. The consequences of a lack of originality can manifest in repetitive patterns and generic applications. A previous article titled Overcoming Lack of Originality in Web Development discusses these issues in-depth. In this post, we will explore how Java frameworks can help developers overcome these challenges while promoting originality.
Understanding the Role of Java Frameworks
Java frameworks streamline application development by providing pre-built structures and functionalities. They reduce boilerplate code and promote best practices, so developers can concentrate on what truly matters: crafting unique features.
Popular Java frameworks include:
- Spring
- JavaServer Faces (JSF)
- Hibernate
- Vaadin
These frameworks each bring unique advantages, and knowing when to utilize them can make a significant difference in the originality of your projects.
Spring Framework: Fostering Innovation
The Spring framework is particularly revered for its flexibility and modular approach. This allows developers to pick the components they need without being confined to a monolithic structure. Here's how you can leverage Spring to enhance creativity in your applications.
Dependency Injection
Spring's core functionality is Dependency Injection (DI). DI allows developers to focus on writing modular code. This can lead to innovative design patterns. Here's a simple code snippet that demonstrates DI in Spring:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class GreetingService {
public String greet() {
return "Hello, World!";
}
}
@Service
public class GreetingController {
private final GreetingService greetingService;
@Autowired
public GreetingController(GreetingService greetingService) {
this.greetingService = greetingService;
}
public String getGreeting() {
return greetingService.greet();
}
}
In this example, GreetingService
provides a simple greeting message. The GreetingController
class uses DI to access GreetingService
. This approach encourages separation of concerns, facilitating more creative solutions as developers can mix and match different services.
Aspect-Oriented Programming (AOP)
Spring also includes AOP features, which enhance modularity by separating cross-cutting concerns from business logic. For example, logging can be managed independently, leaving the main code clean and focused on its core functionality. Here’s a quick example:
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@Aspect
public class LoggingAspect {
private static final Logger logger = LoggerFactory.getLogger(LoggingAspect.class);
@Before("execution(* com.example..*(..))")
public void logBeforeMethod() {
logger.info("A method is about to be executed.");
}
}
This code snippet uses Spring AOP to log messages before method execution. By handling logging through AOP, developers maintain focus on their primary code, fostering originality in logic rather than boilerplate tasks.
Hibernate: Simplifying Data Management
Hibernate is another powerful Java framework that enables developers to manage data with ease. Its Object-Relational Mapping (ORM) capabilities allow developers to work with Java objects rather than SQL code, paving the way for more creative database interactions.
Seamless Data Mapping
With Hibernate, you can focus on your domain model and let Hibernate handle the complexity of database operations. Here's a basic example:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
// Constructors, getters, and setters omitted for brevity
}
In this code, the User
class represents an entity in the database. By mapping Java objects to database tables, Hibernate allows developers to focus on building unique features instead of wading through SQL syntax.
Criteria API
Another powerful feature of Hibernate is its Criteria API, which provides a programmatic way to build database queries. This is beneficial in promoting originality since you can customize queries without writing lengthy SQL code. For instance:
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.criterion.Restrictions;
// Assuming we have a sessionFactory bean configuration
public List<User> findUsersByUsername(String username) {
Session session = sessionFactory.openSession();
Criteria criteria = session.createCriteria(User.class);
criteria.add(Restrictions.eq("username", username));
return criteria.list();
}
Here, the Criteria API is used to locate users based on their username. This fluent API enables developers to express complex queries in a simple way, further enhancing the application's originality.
JavaServer Faces (JSF): Building UI with Creativity
JavaServer Faces is a robust framework for building user interfaces for Java EE applications. JSF uses a component-based structure, promoting a rich client experience.
Managed Beans
JSF employs managed beans to bind UI components to back-end logic. This encourages original designs while ensuring the application stays maintainable. An example of a managed bean is as follows:
import javax.faces.bean.ManagedBean;
@ManagedBean
public class UserBean {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String submit() {
return "success"; // Navigates to success page
}
}
This UserBean
managed bean links the UI with the business logic, promoting clean and modular development. By reusing managed beans across various views, developers can create unique interfaces without reinventing the wheel.
Vaadin: Modern UI Development Made Easy
Vaadin provides an intuitive way to build modern web applications with Java. It abstracts the complexity of server-client communication, allowing developers to create rich web UIs quickly.
Server-Side Architecture
In Vaadin, UI components are created in Java. This server-side architecture allows developers to focus on business logic without worrying about HTML and JavaScript. Here's an example of creating a simple UI:
import com.vaadin.flow.component.button.Button;
import com.vaadin.flow.component.orderedlayout.VerticalLayout;
import com.vaadin.flow.router.Route;
@Route("")
public class MainView extends VerticalLayout {
public MainView() {
Button button = new Button("Click me", event -> {
System.out.println("Button clicked!");
});
add(button);
}
}
This code generates a straightforward button in a Vaadin application. The developer can seamlessly integrate server-side logic while preserving a unique user experience.
Closing Remarks: Embrace Originality with Java Frameworks
As developers navigate the intricate web of originality in web development, Java frameworks stand out as powerful allies. By utilizing frameworks such as Spring, Hibernate, and JSF, developers can enhance modularity, streamline design, and focus on creating unique solutions.
Originality is not merely about reinventing the wheel; it’s about using existing tools to think differently. By understanding these frameworks and how they can simplify and inspire your work, you can significantly elevate your applications.
For more insights into overcoming the challenges of originality in web development, refer to the article Overcoming Lack of Originality in Web Development.
By fully embracing what Java frameworks offer, the obstacles of lack of originality can be transformed into opportunities for creativity. Remember, the key is to use these frameworks as tools to enhance your innovative ideas, not as constraints. The future of web development lies in your hands; let creativity be your guide.