How Java Frameworks Foster Originality in Web Development
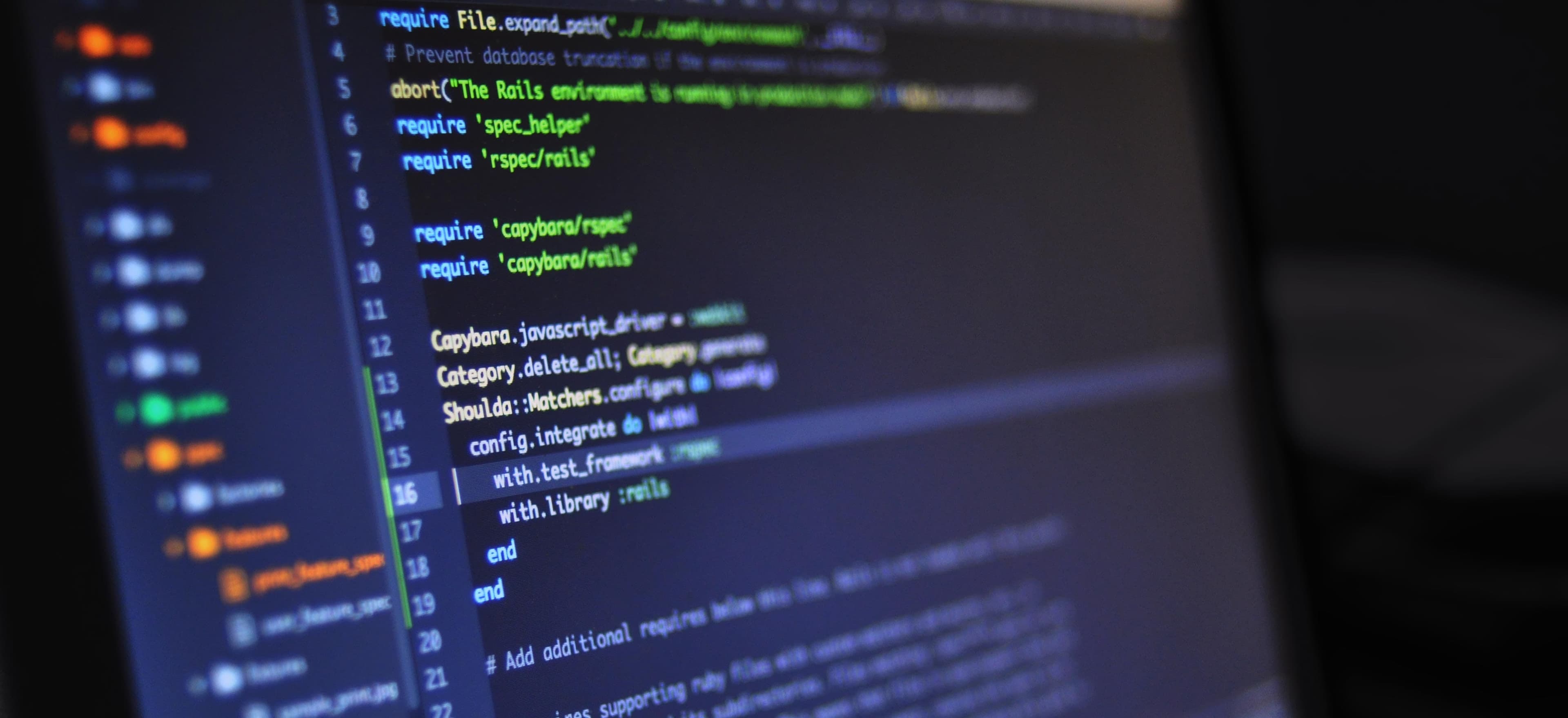
- Published on
How Java Frameworks Foster Originality in Web Development
In the fast-evolving landscape of web development, originality is often overshadowed by the need for speed and efficiency. With numerous frameworks available, developers often find themselves leaning toward established patterns and solutions, resulting in a sea of similar-looking websites. However, Java frameworks present unique opportunities to inject originality into web development. In this post, we will explore how Java frameworks can not only streamline your coding process but also encourage creative approaches to building web applications.
Why Java for Web Development?
Java has been a staple in the programming community for decades. Known for its portability, scalability, and robust security protocols, it remains a go-to choice for enterprise-level applications. Java’s versatility is greatly enhanced by various frameworks that offer built-in functionalities to support developing dynamic web applications. Additionally, Java benefits from an extensive ecosystem of tools, libraries, and a supportive community—forging a perfect storm of creativity and efficiency.
Some of the most popular Java frameworks include:
- Spring
- JavaServer Faces (JSF)
- Struts
- Hibernate
Each of these frameworks offers different strategies for web development, encouraging developers to experiment and innovate.
The Role of Frameworks in Fostering Originality
While frameworks often come with conventions and best practices, they also provide a foundation upon which developers can build custom solutions. Here’s how Java frameworks help foster originality:
1. Modular Architecture
Java frameworks like Spring embrace modularity. This allows developers to create applications as a series of interconnected components, enabling the flexibility to innovate without overhauling the entire system.
@Configuration
public class AppConfig {
@Bean
public MessageService messageService() {
return new EmailService(); // Dependency Injection at work
}
}
Explanation: Here, the AppConfig
class defines a Java configuration for a Spring application. The use of @Bean
allows for easier management of dependencies within a modular setup. Developers can switch out EmailService
for another service like SMSService
without affecting other parts of the application, thus fostering innovation.
2. Flexible Data Handling
Many Java frameworks provide ORM (Object-Relational Mapping) capabilities, like Hibernate. This lets developers interact with databases using Java objects, opening the door for creative ways of structuring data.
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String userName;
// Getter and setter methods omitted for brevity
}
Explanation: In this Hibernate example, the @Entity
annotation indicates that the User
class will be mapped to a database table. Customizing how users are stored, updated, or retrieved allows developers to explore unique data structures, enhancing originality.
3. Rapid Prototyping
Frameworks like Spring Boot enable developers to prototype applications quickly, which is particularly helpful in brainstorming sessions. The fast feedback loop allows for testing out various features or designs without significant upfront investment.
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Explanation: This code snippet demonstrates a basic Spring Boot initializer. The simplicity of setup means that engineers can rapidly implement features, promoting an agile development cycle. This quick iteration can lead to unexpected, innovative functionalities emerging by chance!
4. Custom Widgets and Components
Frameworks such as JavaServer Faces (JSF) allow developers to create reusable UI components. These custom components can lead to novel interfaces that differentiate a web application from competitors.
<ui:composition template="/templates/master.xhtml">
<h:form>
<h:outputLabel value="Username:" for="username" />
<h:inputText id="username" value="#{userBean.username}" />
<h:commandButton value="Submit" action="#{userBean.submit}" />
</h:form>
</ui:composition>
Explanation: This JSF composition creates a simple form using custom components. The separation of logic from presentation encourages developers to think outside of the box, creating unique user experiences.
5. Seamless Integration with Modern Technologies
Java frameworks easily integrate with various modern technologies, such as RESTful APIs and microservices architecture. This interoperability encourages developers to implement diverse functionalities tailored to their specific use cases.
@RestController
@RequestMapping("/api/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
User user = userService.findById(id);
return ResponseEntity.ok(user);
}
}
Explanation: The above Spring Controller responds to HTTP GET requests for user data. By allowing integration with REST APIs, developers can create unique services leveraging external data sources and machine-learning models, thereby enhancing the originality of their applications.
Challenges and The Importance of Originality
While Java frameworks certainly encourage innovation, developers must avoid falling into the trap of over-reliance on predefined solutions. Originality in web development helps maintain user engagement, makes products memorable, and can lead to unique business propositions. As pointed out in the article "Overcoming Lack of Originality in Web Development", developers should strive to create unique experiences rather than simply replicating existing platforms.
Key Takeaways
-
Adaptability: Frameworks like Spring and JSF enable developers to adapt their web applications to meet specific user needs.
-
Modularity: The modular architecture encourages creative addition and interchange of components, minimizing disruption.
-
Rapid Development: Fast prototyping fosters innovative thinking while minimizing the constraints of traditional development cycles.
-
Unique Entities: The ability to create custom components encourages individualized and memorable user experiences.
-
Integration Possibilities: The seamless integration with modern technologies fosters numerous creative possibilities.
To Wrap Things Up
Java frameworks not only provide invaluable tools for web development but also cultivate an ecosystem of originality. By leveraging the unique features of frameworks like Spring, Hibernate, and JSF, developers can create innovative web applications that stand out in a crowded marketplace. To maintain your edge as a developer, embrace the creativity made possible by these frameworks while mindfully avoiding the pitfalls of over-reliance on repetitive solutions.
By fostering this balance of structure and creativity, you’ll not only enhance your skills but also create web applications that are both functional and original. So dive into the world of Java frameworks and let your creativity flow!
Are you ready to push the boundaries of originality in your web applications? Share your thoughts or experiences in the comments below!
Checkout our other articles