Resolving RabbitMQ Installation Hurdles in Java Applications
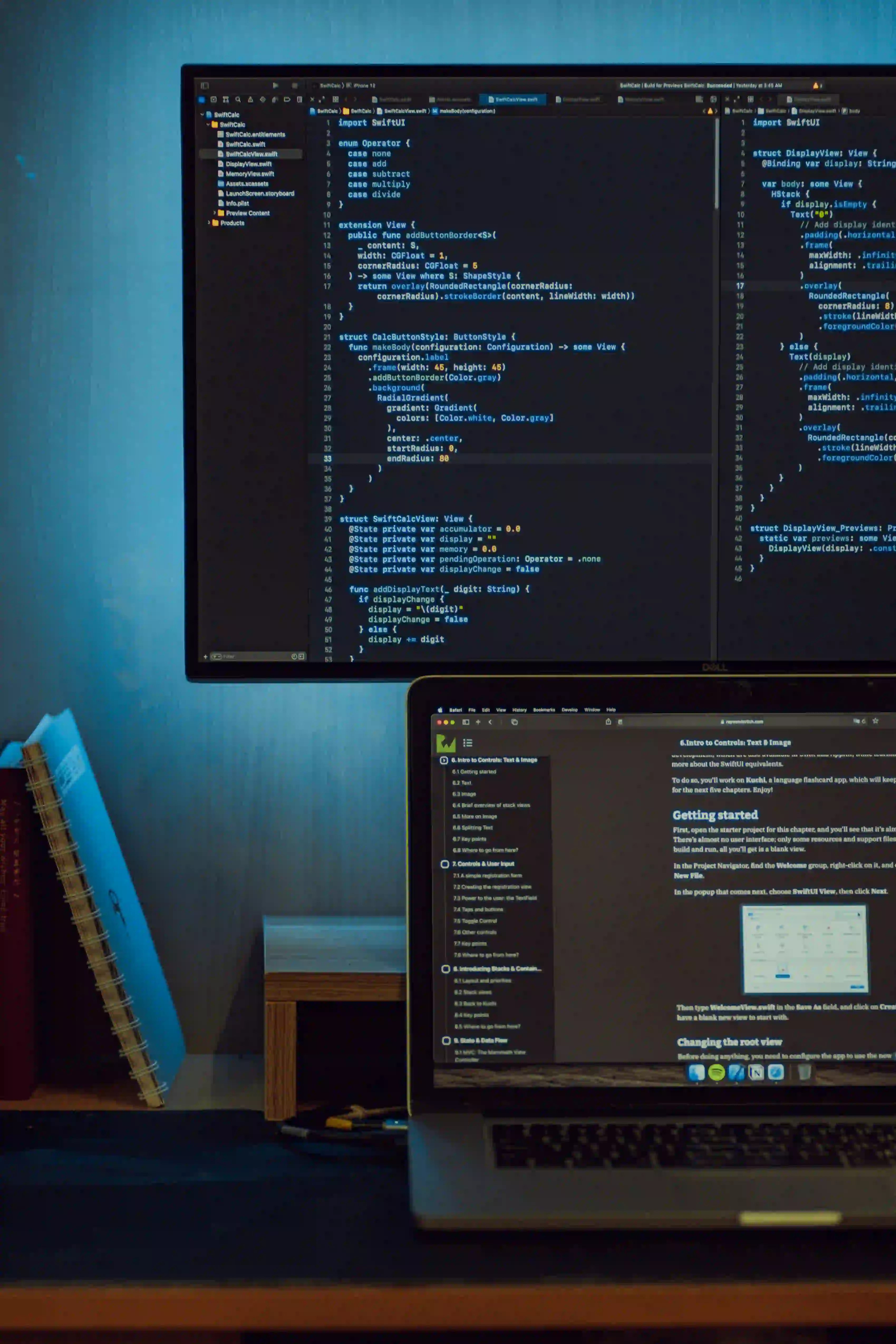
Resolving RabbitMQ Installation Hurdles in Java Applications
In the world of modern applications, message brokers like RabbitMQ play a critical role in facilitating reliable communication between services. However, the installation process can present challenges, especially when deploying on specific operating systems, such as Rocky Linux. If you're a Java developer navigating these waters, it's essential to understand potential issues and how to resolve them efficiently. This blog will provide a road map to successfully install RabbitMQ, along with relevant code snippets to guide your development journey.
Understanding RabbitMQ and Its Importance
RabbitMQ is an open-source message broker that implements the Advanced Message Queuing Protocol (AMQP). It enables applications to communicate with each other by sending messages through queues. This decouples application components, making them easier to scale and maintain. Common use cases include:
- Microservices Communication: In a microservices architecture, RabbitMQ allows disparate services to exchange information without direct dependencies.
- Load Balancing: RabbitMQ can distribute workloads evenly across multiple service instances.
- Asynchronous Processing: Tasks can be processed at a later time, which helps in managing high loads gracefully.
This article assumes familiarity with RabbitMQ and Java Spring Boot applications, specifically how to integrate RabbitMQ for messaging.
Installing RabbitMQ on Rocky Linux: A Quick Overview
While installing RabbitMQ on Rocky Linux is generally straightforward, you might encounter specific issues. For a detailed exploration of common installation problems, refer to the article Common Installation Issues for RabbitMQ on Rocky Linux. Here we will touch on common pitfalls and provide solutions tailored for Java applications.
Prerequisites
Before diving into RabbitMQ installation, make sure you have the following:
- Rocky Linux System: Ensure your server is updated and running.
- Erlang Dependency: RabbitMQ is built on Erlang, and hence it is a prerequisite.
- Java: A JDK (Java Development Kit) installed on your machine, preferably Java 11 or higher for best practices.
Step 1: Installing Erlang
RabbitMQ relies on Erlang to function, so start with its installation. If you already have Erlang installed, jump to the next step.
sudo dnf install epel-release
sudo dnf install erlang
Step 2: Installing RabbitMQ
Now that Erlang is set up, you can proceed with RabbitMQ installation.
# Add the RabbitMQ repository
echo "[rabbitmq]
name=rabbitmq
baseurl=https://dl.bintray.com/rabbitmq/rabbitmq-server/erlang/24/rabbitmq/management/3.9.0/$(uname -m)
gpgcheck=0" | sudo tee /etc/yum.repos.d/rabbitmq.repo
# Install RabbitMQ
sudo dnf install rabbitmq-server
Step 3: Start RabbitMQ Server
After installation, enable and start the RabbitMQ server. The following commands will do just that:
# Enable RabbitMQ service to start on boot
sudo systemctl enable rabbitmq-server
# Start RabbitMQ service
sudo systemctl start rabbitmq-server
# Check status
sudo systemctl status rabbitmq-server
Common Installation Issues and Resolutions
Installation errors can derail your project, but many common mistakes have known solutions. For an exhaustive list of issues, please consider the article mentioned above. Here are a few critical checks:
- Firewalls and Ports: Ensure that ports 5672 (AMQP protocol) and 15672 (Management Plugin) are open. Use the following command to check:
sudo firewall-cmd --list-all
If needed, open the ports:
sudo firewall-cmd --add-port=5672/tcp --permanent
sudo firewall-cmd --add-port=15672/tcp --permanent
sudo firewall-cmd --reload
-
Erlang Version Compatibility: Make sure the installed Erlang version is compatible with your version of RabbitMQ. You can check the compatibility chart on the RabbitMQ official website.
-
Disk Space: Ensure your server has adequate disk space. Insufficient disk space can result in failure to start the service.
Integrating RabbitMQ with Java
Once RabbitMQ is running, you can integrate it with your Java applications. We'll use Spring Boot for a seamless integration experience.
Swagger Configuration
Add the following dependencies in your pom.xml
file to include RabbitMQ and Spring AMQP:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
Configuring RabbitMQ Connection
In your Spring Boot application, you can configure RabbitMQ as follows:
import org.springframework.amqp.core.Queue;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class RabbitConfig {
// Define queue
@Bean
public Queue myQueue() {
return new Queue("myQueue", false);
}
}
Why this code? The configuration creates a Queue bean that can be injected wherever needed in your application. This encapsulates the queue creation logic to centralize RabbitMQ setups.
Sending Messages
To send a message to the queue, you can use the following code in a service class:
import org.springframework.amqp.rabbit.core.RabbitTemplate;
import org.springframework.stereotype.Service;
@Service
public class MessageSender {
private final RabbitTemplate rabbitTemplate;
public MessageSender(RabbitTemplate rabbitTemplate) {
this.rabbitTemplate = rabbitTemplate;
}
public void sendMessage(String message) {
this.rabbitTemplate.convertAndSend("myQueue", message);
}
}
Why this code? The RabbitTemplate
is the main API for sending messages to RabbitMQ, enabling easy communication with the broker.
Receiving Messages
On the receiver side, create a listener to consume messages from the queue:
import org.springframework.amqp.rabbit.annotation.RabbitListener;
import org.springframework.stereotype.Service;
@Service
public class MessageReceiver {
@RabbitListener(queues = "myQueue")
public void receiveMessage(String message) {
System.out.println("Received: " + message);
}
}
Why this code? The @RabbitListener
annotation indicates that this method should listen to messages from the specified queue, allowing asynchronous processing of messages.
The Bottom Line
Integrating RabbitMQ with your Java applications can significantly improve communication and manageability across your services. However, installation issues can arise, particularly on operating systems like Rocky Linux. By understanding these complications and implementing solutions outlined here, along with insights from Common Installation Issues for RabbitMQ on Rocky Linux, you can avoid common pitfalls and ensure a smooth flow of messages in your applications.
As you continue your journey with RabbitMQ and Java, remember that thorough testing and ongoing monitoring are crucial. With the guidance provided in this article, you're now equipped to tackle RabbitMQ integration challenges effectively. Happy coding!