Resolving RabbitMQ Configuration Challenges in Java Apps
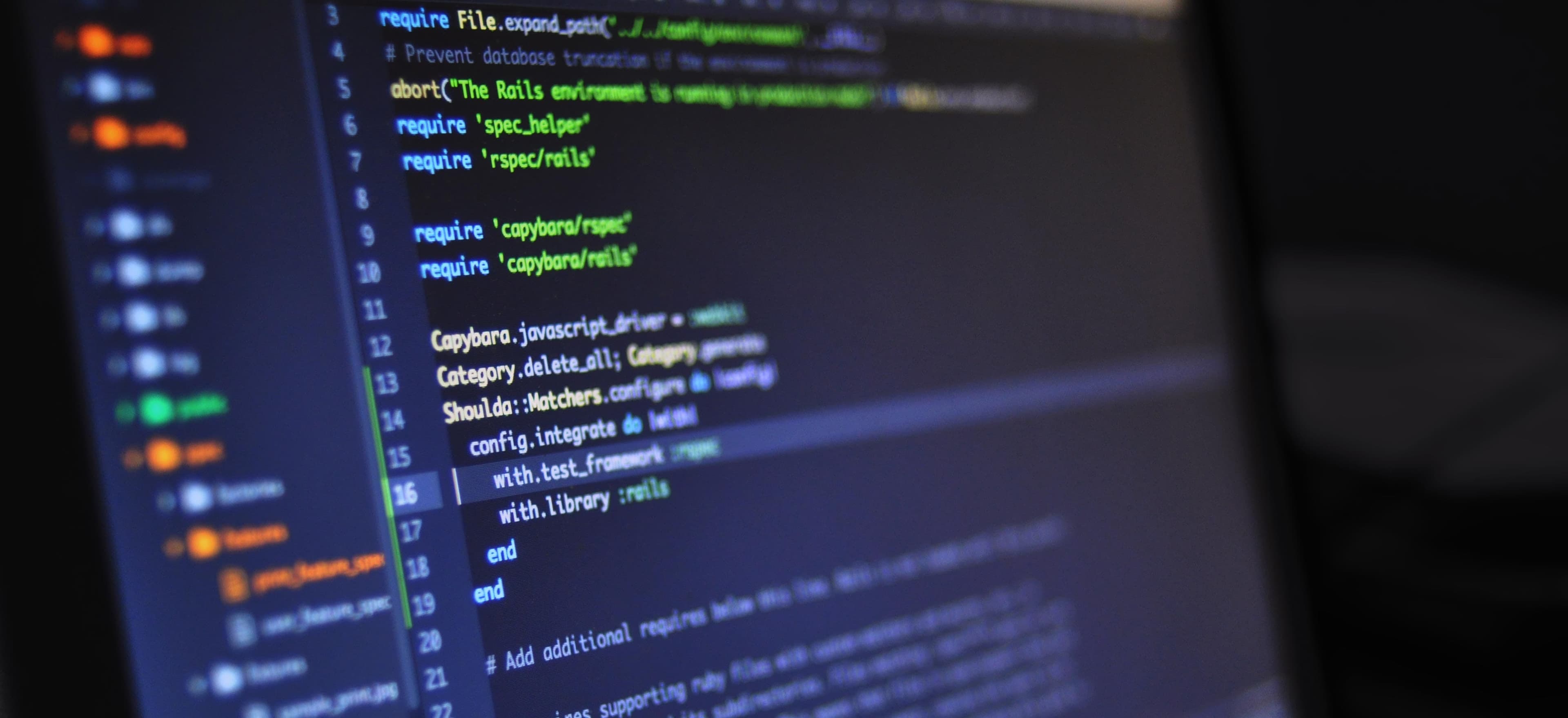
- Published on
Resolving RabbitMQ Configuration Challenges in Java Apps
Getting Started
In today's interconnected world, message brokers like RabbitMQ play a crucial role in enabling asynchronous communication between different parts of an application. This is especially important for Java applications where scalability and performance are key. However, configuring RabbitMQ can sometimes lead to challenges, especially when dealing with nuances in message configurations or connectivity issues.
In this article, we'll dive into common RabbitMQ configuration challenges in Java applications, explore how to resolve them, and provide code snippets to illustrate effective solutions. You’ll also find links to external resources, including an article detailing "Common Installation Issues for RabbitMQ on Rocky Linux," which can serve as a helpful reference during your setup.
What is RabbitMQ?
RabbitMQ is an open-source message broker that facilitates the communication between different applications. It helps to decouple system components, allowing developers to build scalable and efficient systems. With its support for various protocols like AMQP, it can accommodate various messaging patterns, making it a popular choice among developers.
Why Use RabbitMQ with Java?
Java's ecosystem supports multiple frameworks and libraries that interact seamlessly with RabbitMQ. Tools like Spring AMQP provide an easy and intuitive way to connect Java applications with RabbitMQ, allowing you to focus on building features rather than wiring the infrastructure.
Common Configuration Challenges
While working with RabbitMQ in Java applications, developers often encounter several configuration challenges:
- Connection Issues
- Channel Management
- Message Serialization
- Handling Message Acknowledgment
- Error Handling
Let's take a closer look at each issue and provide practical solutions to help you get your Java applications communicating with RabbitMQ effectively.
1. Connection Issues
The first challenge is often establishing a connection to the RabbitMQ server. You need to ensure that your RabbitMQ server is running and that your application can connect to it.
Example Code Snippet:
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.ConnectionFactory;
public class RabbitMQConnector {
private String hostname;
private int port;
public RabbitMQConnector(String hostname, int port) {
this.hostname = hostname;
this.port = port;
}
public Connection createConnection() throws Exception {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost(hostname);
factory.setPort(port);
return factory.newConnection();
}
}
Commentary:
- This code snippet demonstrates how to create a connection to RabbitMQ with a specified hostname and port. By using
ConnectionFactory
, we set up the connection parameters crucial for establishing communication. - Ensure that the RabbitMQ server is accessible and running on the specified hostname and port. It’s important to handle exceptions properly for a more resilient application.
2. Channel Management
After establishing a connection, the next step is to create a channel. Channels are virtual connections that facilitate communication, and managing them correctly is paramount.
Example Code Snippet:
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.Connection;
public class RabbitMQChannel {
private Connection connection;
public RabbitMQChannel(Connection connection) {
this.connection = connection;
}
public Channel createChannel() throws Exception {
return connection.createChannel();
}
}
Commentary:
- The
createChannel
method is essential for establishing communication between the producer and consumer. By using virtual channels, we ensure that our application can handle multiple streams of data without the overhead of creating multiple connections.
3. Message Serialization
Message serialization can be a stumbling block when sending complex objects. Using JSON is a common practice to serialize and deserialize messages.
Example Code Snippet:
import com.fasterxml.jackson.databind.ObjectMapper;
public class MessageSerializer {
private static final ObjectMapper objectMapper = new ObjectMapper();
public static String serialize(Object obj) throws Exception {
return objectMapper.writeValueAsString(obj);
}
public static <T> T deserialize(String json, Class<T> clazz) throws Exception {
return objectMapper.readValue(json, clazz);
}
}
Commentary:
- The
MessageSerializer
class provides methods to convert Java objects to JSON strings and vice versa. This serialization approach facilitates interoperability between different programming environments, ensuring that your Java application can communicate effectively with other services.
4. Handling Message Acknowledgment
Message acknowledgment is a critical aspect of RabbitMQ to ensure that messages are processed successfully. Implementing acknowledgment is crucial for reliability.
Example Code Snippet:
import com.rabbitmq.client.Channel;
public class MessageReceiver {
private Channel channel;
public MessageReceiver(Channel channel) {
this.channel = channel;
}
public void receiveMessages(String queueName) throws Exception {
channel.basicConsume(queueName, false, (consumerTag, delivery) -> {
try {
String message = new String(delivery.getBody(), "UTF-8");
// process the message
System.out.println("Received: " + message);
// Manually acknowledge the message
channel.basicAck(delivery.getEnvelope().getDeliveryTag(), false);
} catch (Exception e) {
// Handle exceptions by possibly re-queuing the message
System.out.println("Error processing message: " + e.getMessage());
channel.basicNack(delivery.getEnvelope().getDeliveryTag(), false, true);
}
}, consumerTag -> {});
}
}
Commentary:
- In this example, the
receiveMessages
method handles message consumption. We usebasicConsume
to read messages from the specified queue and acknowledge them only after they have been processed. - By implementing manual acknowledgment, we can control the flow of messages significantly, which increases the reliability of message processing.
5. Error Handling
Finally, error handling in messaging systems is vital. In case of an error, you might want to retry sending messages or log the error for auditing.
Example Code Snippet:
public class RabbitMQErrorHandler {
public static void handleError(Exception e) {
// Log or send the error to an external system
System.err.println("Error: " + e.getMessage());
}
}
Commentary:
- Implement an error handler to centralize error management. Not only does this maintain cleaner code, but also ensures that you have a single point to manage error responses.
Conclusion
Effective RabbitMQ configuration is crucial for the success of your Java applications. By understanding common challenges such as connection issues, channel management, message serialization, acknowledgment handling, and errors, you can construct a robust messaging system.
If you're facing any installations issues on your server, I highly recommend checking out Common Installation Issues for RabbitMQ on Rocky Linux for additional insights.
By implementing the strategies discussed in this article, you can resolve most RabbitMQ configuration challenges and set the foundation for a scalable, maintainable, and efficient Java application. Happy coding!
Checkout our other articles