Managing Data Conversion in Spring-JSF Integration
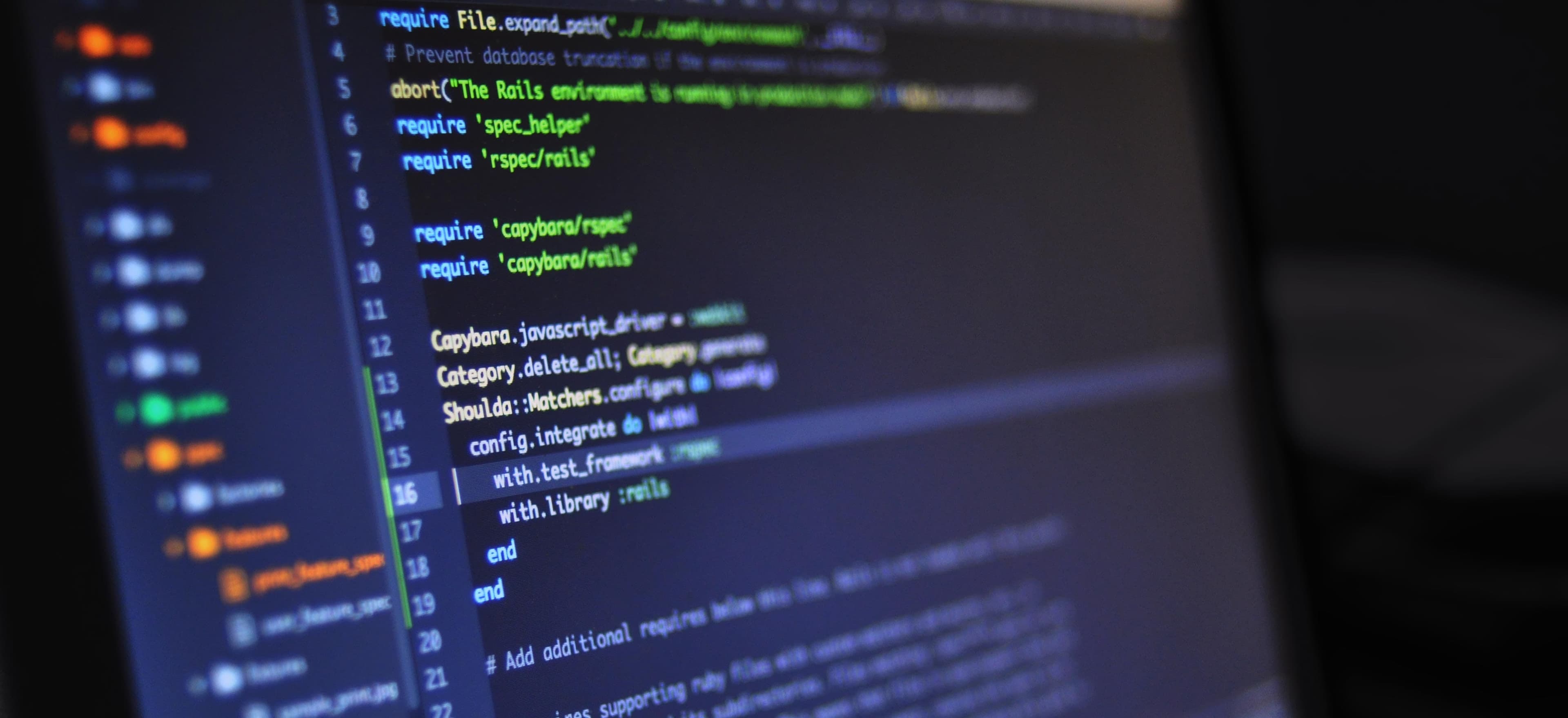
- Published on
Managing Data Conversion in Spring-JSF Integration
When working with a Spring-JSF (JavaServer Faces) integration, one of the key challenges is managing data conversion between the two frameworks. Spring and JSF have different mechanisms for managing data, and ensuring seamless data conversion is crucial for the smooth functioning of the application.
In this blog post, we will explore the best practices for managing data conversion in Spring-JSF integration, and how to handle common scenarios effectively.
Understanding Data Conversion in Spring and JSF
Before delving into the specifics of managing data conversion in the integration of Spring and JSF, it's essential to understand how each framework handles data conversion.
Spring Framework
In Spring, data conversion is typically managed using Converter
and Formatter
interfaces. These interfaces provide a way to convert between a source type and a target type. Spring automatically applies converters and formatters as needed, based on the types involved.
Let's take an example of a simple Spring converter:
@Component
public class StringToUserConverter implements Converter<String, User> {
@Override
public User convert(String source) {
// Convert String to User object
}
}
In this example, StringToUserConverter
converts a String to a User object. Spring will automatically use this converter when needed, based on the declared types.
JavaServer Faces (JSF)
In JSF, data conversion is managed through converters and validators. Converters are responsible for converting the string representation of a value to a Java object, and vice versa. Validators, on the other hand, validate the input values.
A simple JSF converter looks like this:
@FacesConverter("userConverter")
public class UserConverter implements Converter {
@Override
public Object getAsObject(FacesContext context, UIComponent component, String value) {
// Convert String to User object
}
@Override
public String getAsString(FacesContext context, UIComponent component, Object value) {
// Convert User object to String
}
}
In this example, UserConverter
is responsible for converting a String to a User object and vice versa.
Integrating Spring Converters with JSF Converters
Now that we have an understanding of how data conversion is managed in Spring and JSF, let's explore how we can integrate Spring converters with JSF converters effectively.
Using Spring Converters in JSF
When integrating Spring with JSF, it's common to use Spring data converters within JSF managed beans. To achieve this, we can leverage the @ManagedProperty
annotation in JSF managed beans to inject Spring beans.
Let's look at an example:
@ManagedBean
@RequestScoped
public class UserBean {
@ManagedProperty("#{stringToUserConverter}")
private Converter<String, User> stringToUserConverter;
// Getter and setter for stringToUserConverter
// JSF-specific logic using Spring converter
}
Here, we inject the Spring StringToUserConverter
into a JSF managed bean UserBean
, allowing us to seamlessly use the Spring converter within JSF components.
Using JSF Converters in Spring
Conversely, it might be necessary to use a JSF converter within Spring components. While JSF converters are not Spring managed beans, we can still make use of them in Spring components.
We can achieve this by creating a Spring component that acts as a bridge between JSF converters and Spring components, allowing for seamless integration.
@Component
public class JSFConverterBridge {
@ManagedProperty("#{userConverter}")
private Converter userConverter;
// Getter and setter for userConverter
// Spring-specific logic using JSF converter
}
In this example, JSFConverterBridge
serves as a Spring beans that wraps the JSF converter, enabling its usage within Spring components.
Handling Complex Data Conversion Scenarios
In real-world applications, data conversion scenarios can become more complex. It's essential to have a robust strategy for managing these scenarios effectively.
Conditional Data Conversion
There might be cases where the data conversion logic needs to be conditional based on certain factors. For example, a converter might need to handle different conversion logic based on the user's role or the current application state.
To handle conditional data conversion, we can use a combination of Spring's Converter
and Formatter
interfaces along with conditional checks to determine the appropriate conversion logic.
@Component
public class ConditionalConverter implements Converter<String, Object> {
@Autowired
private UserConverter userConverter;
@Autowired
private AdminConverter adminConverter;
@Override
public Object convert(String source) {
if (/* conditional check */) {
return userConverter.convert(source);
} else {
return adminConverter.convert(source);
}
}
}
In this example, ConditionalConverter
demonstrates conditional data conversion based on a certain condition, leveraging other converters as needed.
Bi-Directional Data Conversion
In some scenarios, it's necessary to support bi-directional data conversion, where a value needs to be converted from one type to another and back. This is common in forms where the user inputs a value, which then needs to be converted for display, and vice versa.
To handle bi-directional data conversion, we can utilize both the getAsObject
and getAsString
methods in JSF converters, ensuring seamless conversion in both directions.
@FacesConverter("biDirectionalConverter")
public class BiDirectionalConverter implements Converter {
@Override
public Object getAsObject(FacesContext context, UIComponent component, String value) {
// Convert String to Object
}
@Override
public String getAsString(FacesContext context, UIComponent component, Object value) {
// Convert Object to String
}
}
In this example, BiDirectionalConverter
showcases bi-directional data conversion, where the getAsObject
method handles conversion from String to Object, and the getAsString
method handles conversion from Object to String.
Final Considerations
Managing data conversion in Spring-JSF integration is a critical aspect of building robust and efficient applications. By understanding the data conversion mechanisms in both frameworks and effectively integrating Spring and JSF converters, we can ensure seamless data handling.
Handling complex data conversion scenarios, such as conditional and bi-directional conversion, further enhances the capabilities of our applications, providing a smooth user experience.
Understanding these principles and best practices ensures that our Spring-JSF integrated applications perform optimally, with well-managed data conversion at the core of their functionality.
In conclusion, effective data conversion management is a key aspect of successful Spring-JSF integration, and mastering it will result in robust and efficient applications.
To explore more about Spring and JSF integration, check out this detailed guide that sheds light on the integration and how to leverage the best of both frameworks.
Feel free to share your thoughts and experiences on managing data conversion in Spring-JSF integration. Thank you for reading!