Creating Context Menus in JavaFX Without FXML
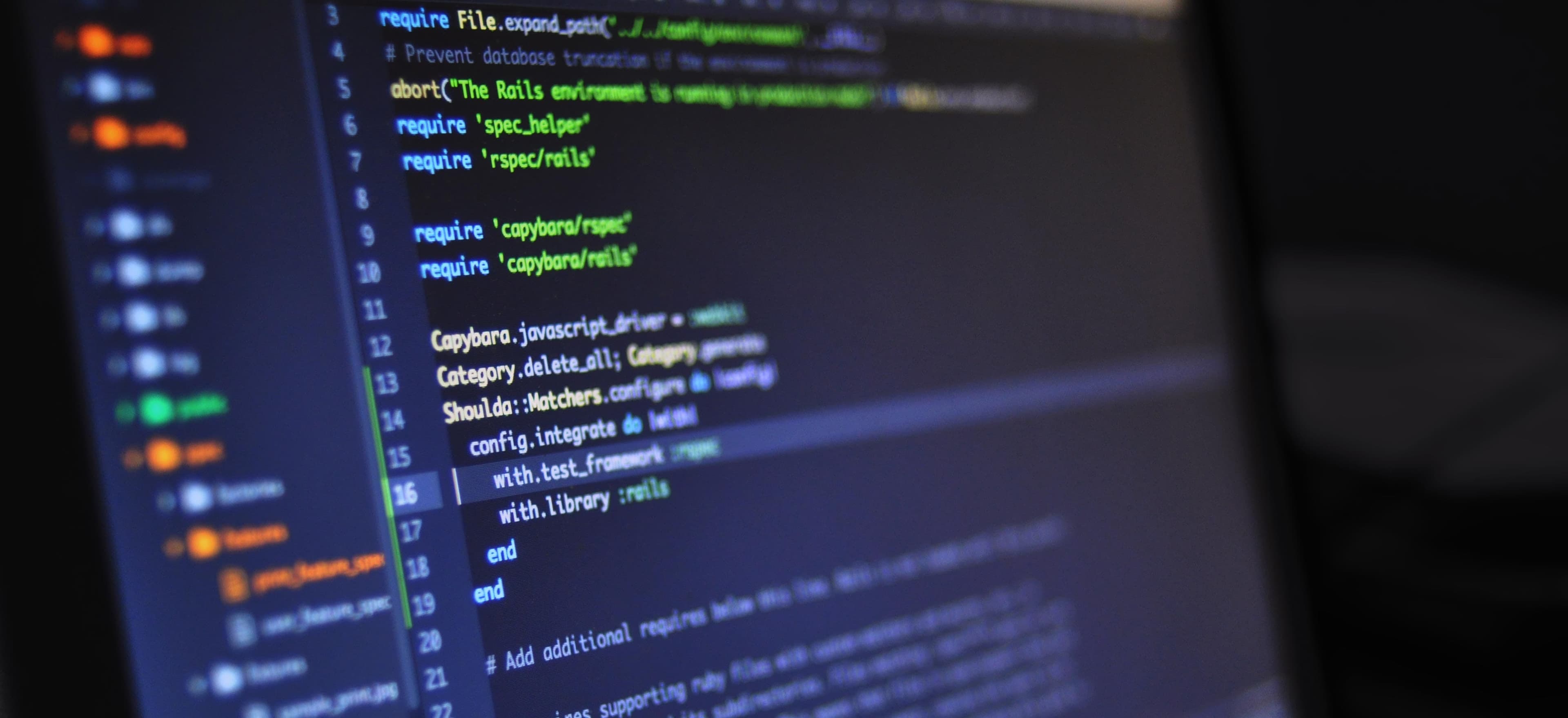
- Published on
How to Create Context Menus in JavaFX Without FXML
When working with JavaFX, creating context menus can be a powerful way to provide users with additional options and functionality. However, many tutorials focus on using FXML for JavaFX development. While FXML certainly has its benefits, it's also important to know how to achieve the same result without it.
In this post, we'll explore how to create context menus in JavaFX without using FXML. We'll cover everything from creating the context menu itself to handling actions when menu items are clicked. By the end of this tutorial, you'll have a solid understanding of how to implement context menus in JavaFX using pure Java code.
Setting Up the JavaFX Application
Before we dive into creating context menus, let's set up a simple JavaFX application to work with. First, ensure that you have the necessary JavaFX dependencies in your project. If you're using Maven, you can add the following dependencies to your pom.xml
file:
<dependencies>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>16</version>
</dependency>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-graphics</artifactId>
<version>16</version>
</dependency>
</dependencies>
Next, let's create a simple JavaFX application with a Scene
containing a Button
that will demonstrate the usage of a context menu.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class ContextMenuExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Right-click me");
StackPane layout = new StackPane();
layout.getChildren().add(button);
Scene scene = new Scene(layout, 300, 250);
primaryStage.setScene(scene);
primaryStage.setTitle("Context Menu Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Now that we have our basic JavaFX application set up, let's move on to creating a context menu for the button.
Creating the Context Menu
To create a context menu in JavaFX without using FXML, we'll instantiate a ContextMenu
and add MenuItem
instances to it. In this example, we'll create a simple context menu with a single "Delete" option.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ContextMenu;
import javafx.scene.control.MenuItem;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class ContextMenuExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Right-click me");
StackPane layout = new StackPane();
layout.getChildren().add(button);
ContextMenu contextMenu = new ContextMenu();
MenuItem deleteItem = new MenuItem("Delete");
contextMenu.getItems().add(deleteItem);
button.setOnContextMenuRequested(event -> {
contextMenu.show(button, event.getScreenX(), event.getScreenY());
});
Scene scene = new Scene(layout, 300, 250);
primaryStage.setScene(scene);
primaryStage.setTitle("Context Menu Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In the code above, we create a ContextMenu
object and a MenuItem
labeled "Delete." We then add the MenuItem
to the context menu using contextMenu.getItems().add(deleteItem)
. Whenever the button is right-clicked (setOnContextMenuRequested
), the context menu is displayed at the mouse's position using contextMenu.show(button, event.getScreenX(), event.getScreenY())
.
Handling Context Menu Actions
Now that we have a context menu appearing when the button is right-clicked, we need to handle the action when the "Delete" option is selected. To do this, we'll use the setOnAction
method of the MenuItem
class.
deleteItem.setOnAction(event -> {
// Handle the delete action here
System.out.println("Delete option selected");
});
By adding the setOnAction
handler to the MenuItem
instance, we specify the action to be taken when the item is selected. In this case, we simply print a message to the console, but you can replace this with your desired functionality.
Wrapping Up
In this tutorial, we've explored how to create context menus in JavaFX without using FXML. We've covered the basics of creating a context menu, adding menu items, and handling actions when menu items are clicked. This knowledge is essential for any JavaFX developer looking to enhance the user experience by incorporating context menus into their applications.
By understanding the process of creating context menus in JavaFX without FXML, you have expanded your JavaFX toolkit and can now leverage this knowledge in your future projects. Remember to explore JavaFX documentation and continue honing your skills to become a proficient JavaFX developer.
Now that you have a solid understanding of creating context menus in JavaFX without FXML, why not take a step further and explore JavaFX's official documentation to uncover more advanced features and best practices? Happy coding!