Why Incorrect hashCode() Can Break Your Java App
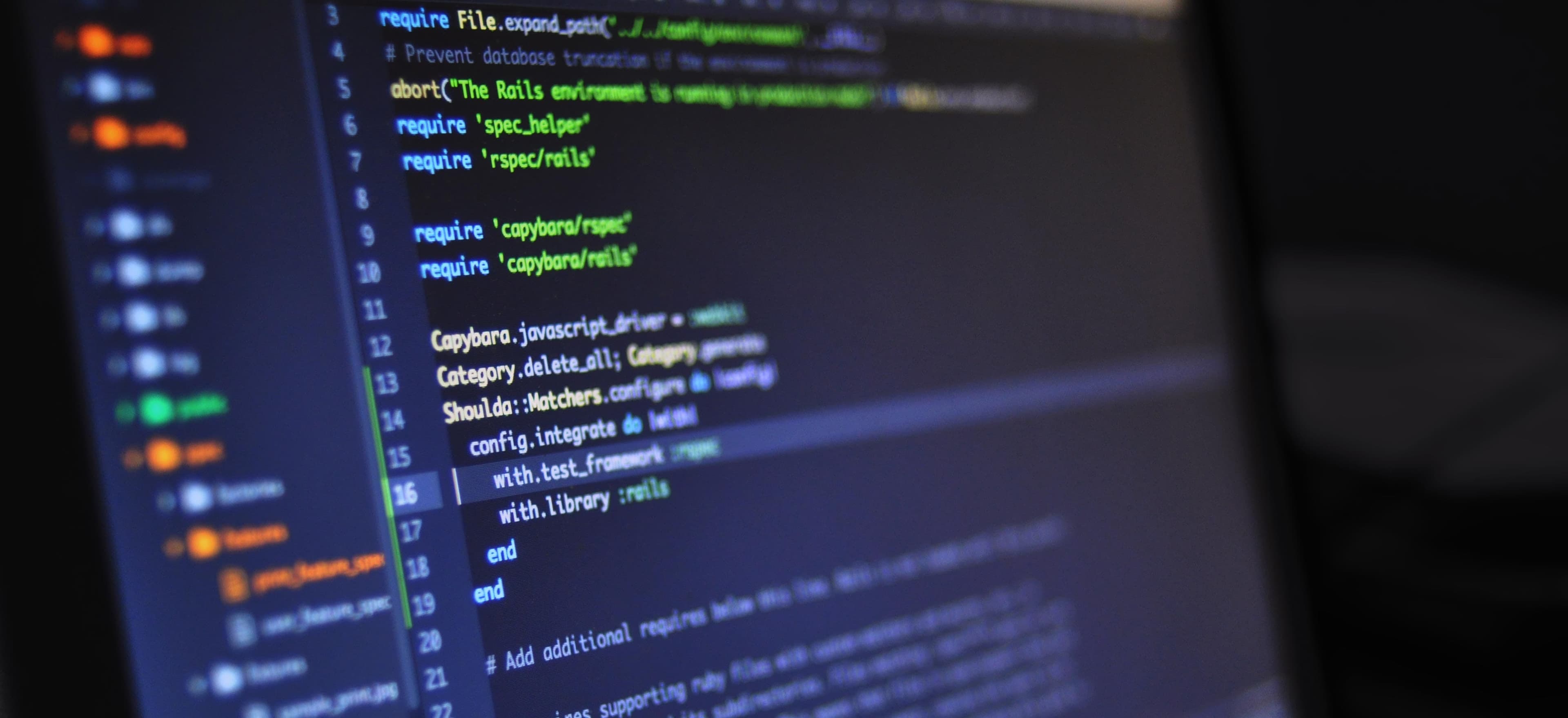
- Published on
Why Incorrect hashCode() Can Break Your Java App
In the world of Java programming, understanding the workings of the hashCode()
method is crucial. It's a simple idea, yet its implications can be profound, significantly affecting the performance and correctness of your program. In this blog post, we will delve into the importance of correctly implementing the hashCode()
method. We will look at how an incorrect implementation can lead to unexpected behaviors, particularly when using hash-based collections like HashSet
, HashMap
, and Hashtable
.
The Basics of hashCode()
Before diving deeper, let's clarify what the hashCode()
method does. Every Java object inherits this method from the Object
class, which is designed to return an integer hash code representation of the object. The crucial aspect is that this hash code is used to place the object in hash-based collections.
Here is a basic example of the hashCode()
method:
@Override
public int hashCode() {
return this.id; // assuming id is a unique identifier for each instance
}
In this example, we're using a simple property (id
) to generate the hash code. However, generating a hash code is not as straightforward as it seems.
Why hashCode() is Important
The hashCode()
method mainly serves two purposes:
-
Optimizing Search Operations: Hash-based collections like
HashMap
use the hash code of an object to determine where to store it in the underlying structure. This makes lookups, insertions, and deletions average O(1) time complexity. -
Maintaining Object Equality: The contract of
hashCode()
states that if two objects are equal according to theequals()
method, then callinghashCode()
on both objects must produce the same integer result.
This means the following two statements are true:
a.equals(b) ==> a.hashCode() == b.hashCode()
If this condition is violated, the behavior of hash-based collections can become unpredictable.
Consequences of an Incorrect hashCode() Implementation
1. Inconsistent Behavior in Collections
If hashCode()
is not consistent with equals()
, you may face scenarios where two equal objects cannot be found in a collection. Look at this example:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Person)) return false;
Person person = (Person) o;
return age == person.age && name.equals(person.name);
}
@Override
public int hashCode() {
return age; // Incorrect! Should consider 'name' too
}
}
In this implementation, two Person
objects that have the same name
and age
are equal, but if they have different names, their hash codes will still be the same (since they will be equal). This leads to inconsistencies when these objects are stored in a HashSet
or as keys in a HashMap
.
2. Performance Degradation
When objects generate the same hash code, they collide in the hash table. A good hash code ensures that each bucket in the hash table is used somewhat evenly. If hash codes aren’t distributed properly, it can cause all elements to fall into the same bucket, leading to a worst-case performance of O(n) instead of the average O(1).
Here's a quick fix to the above example:
@Override
public int hashCode() {
return Objects.hash(name, age); // Using both name and age
}
This revision ensures a better distribution of hash codes among the objects, enhancing performance in collections.
Best Practices for Implementing hashCode()
-
Use All Relevant Fields: Include all fields that are used in the
equals()
method within thehashCode()
method. -
Consistent Implementation: Ensure that the
hashCode()
method is consistent withequals()
. -
Avoid Frequent Changes: If your object’s fields used in
hashCode()
change, it could break its contract with hash-based collections. Consider using immutable objects or making fields final where possible. -
Use Java’s Built-In Functions: Use
Java.util.Objects.hash(...)
for generating hash codes, as it’s designed to handle null cases and gives a more balanced distribution.
Example of a Correct Implementation
Let's rewrite the Person
class using best practices:
import java.util.Objects;
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Person)) return false;
Person person = (Person) o;
return age == person.age && name.equals(person.name);
}
@Override
public int hashCode() {
return Objects.hash(name, age); // Uses both fields for better distribution
}
}
Key Takeaways
In summary, the hashCode()
method in Java is essential for the functionality of hash-based collections. An incorrect implementation can lead to severe performance issues and logical errors, disrupting the whole application's flow. Whenever you override equals()
, remember to override hashCode()
, ensuring they adhere to their contract.
If you're interested in more comprehensive Java practices, consider reading Effective Java by Joshua Bloch, which covers the topic of hashCode()
in more detail along with many other best practices.
By understanding and implementing hashCode()
correctly, you can enhance the robustness and efficiency of your Java applications. So, the next time you write a class, do not forget to consider how you're using it with collections!
Feel free to ask questions or share comments below! Happy coding!
Checkout our other articles