Efficient Random Date Generation in Java Applications
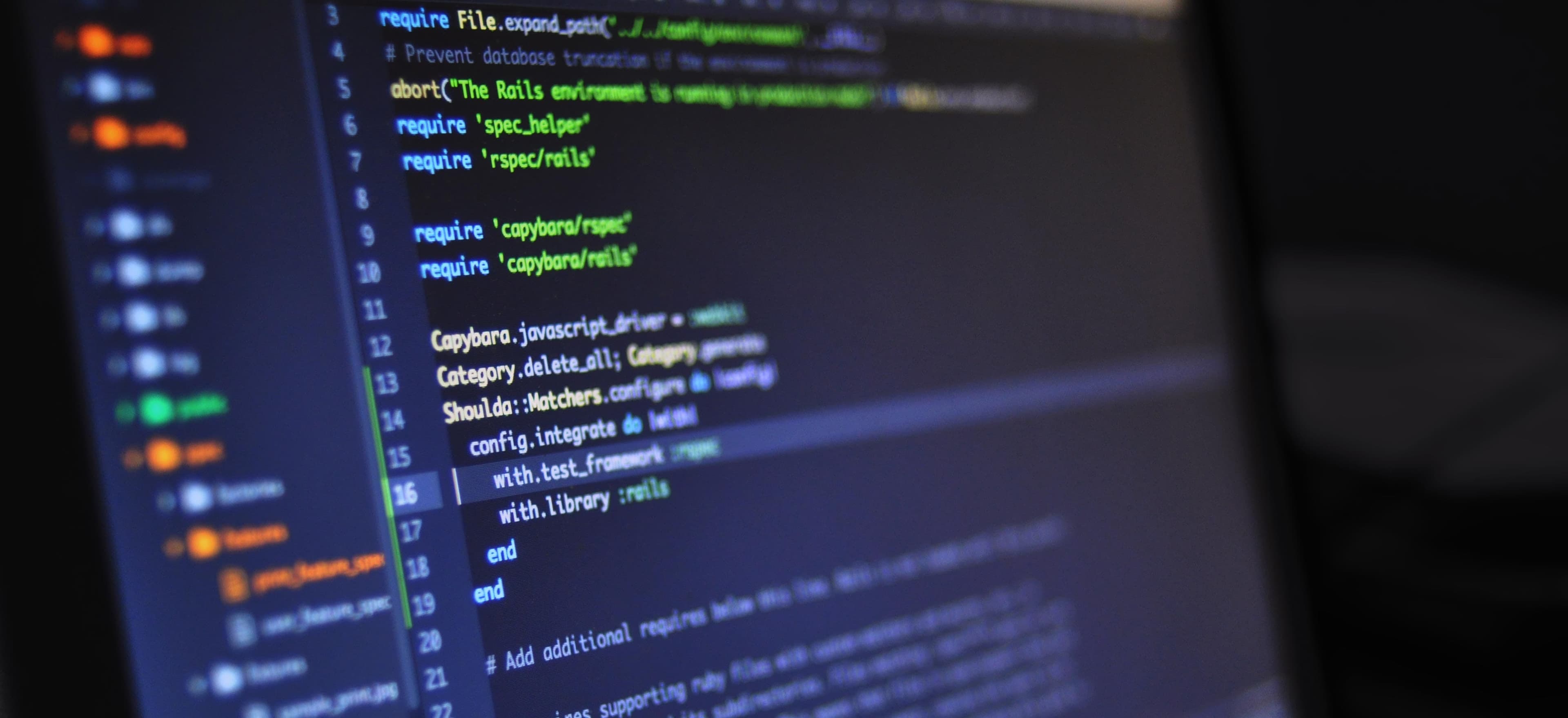
- Published on
Efficient Random Date Generation in Java Applications
In the age of big data and rapidly changing technology, generating random dates for testing and simulation purposes has become increasingly important. Java, one of the most popular programming languages, offers various libraries and methods to create random dates efficiently. Today, we will delve into how to generate random dates using Java, why you would want to do this, and what best practices you should adopt.
The Relevance of Random Dates
Whether you're testing a software application, performing data analysis, or creating pseudo-random data for simulations, generating random dates can have a huge impact. Random dates allow developers and analysts to test functionalities, validate workflows, and simulate various time-sensitive scenarios.
For a deeper understanding of the importance of generating random dates and the challenges involved, check out the excellent article titled Generating Random Dates: Overcoming Time Constraints.
Overview of Java Date Classes
Java provides several classes for handling date and time through the java.time
package introduced in Java 8. This new API is more comprehensive and easy to use compared to the older java.util.Date
and java.util.Calendar
classes. The most relevant classes you'll be working with include:
- LocalDate: This represents a date without a time-zone.
- LocalDateTime: This represents a date-time without a time-zone.
- Instant: This represents a specific moment on the time-line in UTC.
Generating Random Dates
Step 1: Understand Time Constraints
When generating random dates, it is crucial to define the range of dates from which the random date will be selected. This ensures the generated dates are relevant for your testing scenarios.
Step 2: Implementation
Here's a basic implementation demonstrating how to generate random dates using Java's LocalDate
class.
import java.time.LocalDate;
import java.time.temporal.ChronoUnit;
import java.util.Random;
public class RandomDateGenerator {
private static final Random random = new Random();
/**
* Generates a random LocalDate between the given start and end dates.
*
* @param startInclusive The start date (inclusive).
* @param endInclusive The end date (inclusive).
* @return A random LocalDate.
*/
public static LocalDate generateRandomDate(LocalDate startInclusive, LocalDate endInclusive) {
long startEpochDay = startInclusive.toEpochDay();
long endEpochDay = endInclusive.toEpochDay();
long randomEpochDay = startEpochDay + random.nextInt((int) (endEpochDay - startEpochDay + 1));
return LocalDate.ofEpochDay(randomEpochDay);
}
public static void main(String[] args) {
LocalDate startDate = LocalDate.of(2020, 1, 1);
LocalDate endDate = LocalDate.of(2023, 12, 31);
LocalDate randomDate = generateRandomDate(startDate, endDate);
System.out.println("Random Date: " + randomDate);
}
}
Why This Code Works
- Epoch Day Conversion: The code converts the
LocalDate
objects to epoch days. This numeric representation simplifies random number generation. - Random Selection Logic: A random integer is generated based on the difference between the epoch days of the start and end dates. This keeps all generated dates within bounds.
- Efficient Execution: It effectively utilizes Java's built-in capabilities to ensure performance efficiency.
Step 3: Expanding the Functionality
Suppose you also want to incorporate time along with dates. This can be achieved with LocalDateTime
. Below is an example that extends the previous random date generation by incorporating random hours, minutes, and seconds.
import java.time.LocalDateTime;
import java.util.Random;
public class RandomDateTimeGenerator {
private static final Random random = new Random();
/**
* Generates a random LocalDateTime between the given start and end datetimes.
*
* @param startInclusive The start datetime (inclusive).
* @param endInclusive The end datetime (inclusive).
* @return A random LocalDateTime.
*/
public static LocalDateTime generateRandomDateTime(LocalDateTime startInclusive, LocalDateTime endInclusive) {
long startEpochSecond = startInclusive.toEpochSecond(LocalDateTime.now().getOffset());
long endEpochSecond = endInclusive.toEpochSecond(LocalDateTime.now().getOffset());
long randomEpochSecond = startEpochSecond + random.nextInt((int) (endEpochSecond - startEpochSecond + 1));
return LocalDateTime.ofEpochSecond(randomEpochSecond, 0, LocalDateTime.now().getOffset());
}
public static void main(String[] args) {
LocalDateTime startDateTime = LocalDateTime.of(2020, 1, 1, 0, 0);
LocalDateTime endDateTime = LocalDateTime.of(2023, 12, 31, 23, 59);
LocalDateTime randomDateTime = generateRandomDateTime(startDateTime, endDateTime);
System.out.println("Random DateTime: " + randomDateTime);
}
}
Why Is This Code Important?
- Dynamic Range: It uses the epoch seconds to ensure the random time falls within the specified range.
- Combining Date and Time: This caters to scenarios where both date and time are critical, providing more granular testing scenarios.
- Simple to Read: The code is simple and straightforward, making it easy for new developers to understand and modify for their needs.
Best Practices for Random Date Generation
- Limit the Range: Always define a reasonable start and end date to prevent irrelevant or out-of-bound dates.
- Thread Safety: If you’re working in a multi-threaded environment, make sure to use thread-safe random number generation to avoid conflicts.
- Seeding: Consider using a seeded random generator for reproducibility when needed.
- Testing: Always validate the generated dates to ensure they meet your requirements.
In Conclusion, Here is What Matters
Generating random dates in Java is a relatively straightforward task, but implementing it correctly can greatly improve the robustness of your applications. The provided examples illustrate the power of Java's java.time
package in generating both LocalDate
and LocalDateTime
. By following the tips outlined and understanding the code, developers can easily adapt these methods to their specific use cases.
If you want to learn more about random date generation and its challenges, you can revisit the article titled Generating Random Dates: Overcoming Time Constraints.
With these insights and examples, you're now equipped to efficiently generate random dates for your Java applications! Happy coding!
Checkout our other articles