Troubleshooting memory issues in Java applications
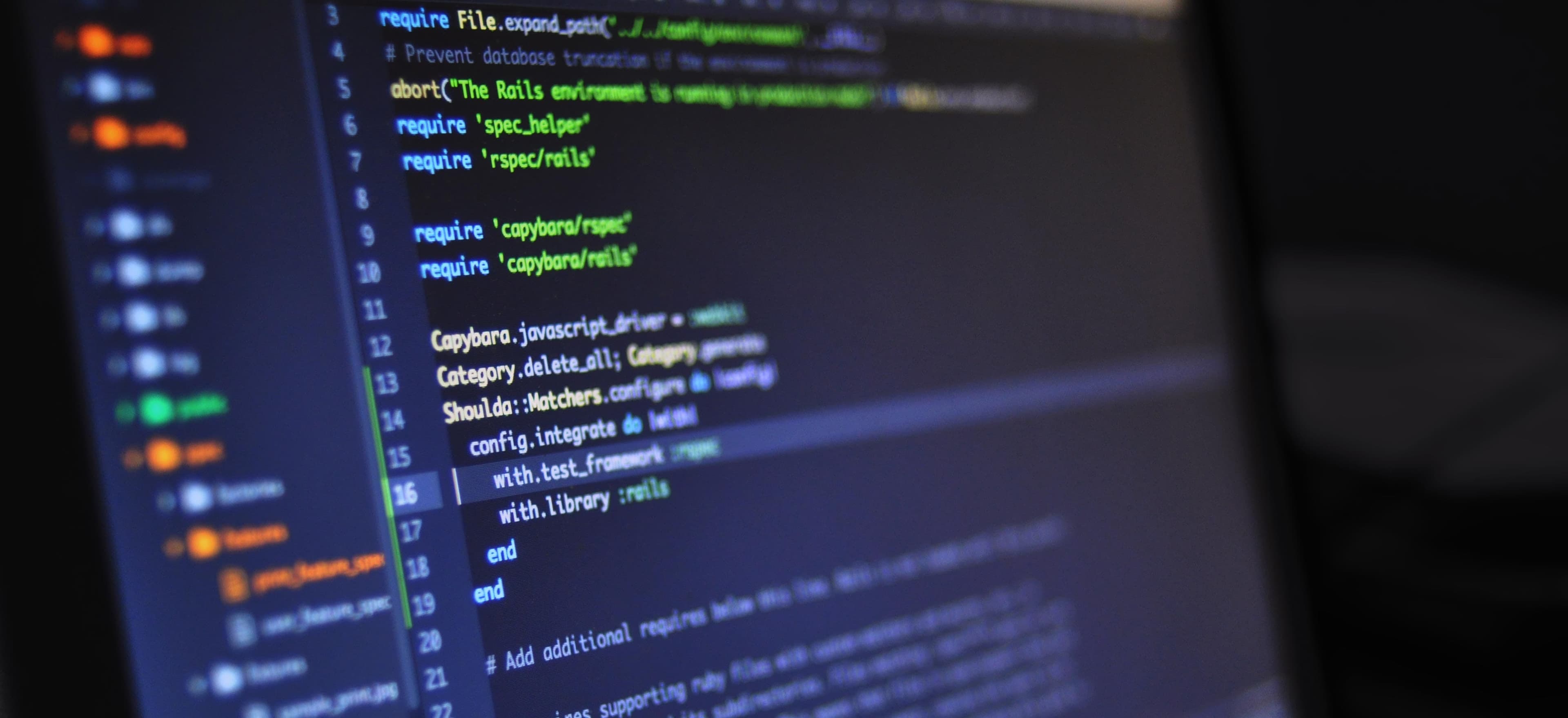
- Published on
Troubleshooting Memory Issues in Java Applications
Memory management is a critical aspect of developing Java applications. The Java Virtual Machine (JVM) manages memory allocation and deallocation automatically, but memory-related issues can still arise, impacting the application's performance and stability. In this blog post, we will delve into common memory-related problems in Java applications and how to troubleshoot them effectively.
Understanding Memory Management in Java
Before delving into troubleshooting, it's crucial to understand how memory management works in Java. Java uses a combination of stack and heap memory for managing data and method calls. The heap memory is where objects are stored, while the stack memory is used for method calls and local variables.
Common Memory-Related Issues
1. OutOfMemoryError
One of the most common memory-related issues in Java is the OutOfMemoryError
. This error occurs when the JVM cannot allocate enough memory for new objects. It can be caused by memory leaks, excessive garbage collection, or simply insufficient memory allocation for the application.
2. Memory Leaks
Memory leaks occur when objects are no longer needed by the application but are still referenced, preventing the garbage collector from reclaiming their memory. Over time, this can lead to an OutOfMemoryError as the heap becomes full of unreferenced objects.
Troubleshooting Memory Issues
1. Analyzing Heap Dumps
When encountering memory-related issues, analyzing heap dumps can provide valuable insights into the application's memory usage. Tools like VisualVM or Java Mission Control can be used to generate and analyze heap dumps. These tools can help identify memory leaks, large object instances, and the overall memory usage of the application.
2. Profiling the Application
Profiling the application using tools like YourKit or JProfiler can help identify memory hotspots, memory leaks, and excessive object creation. Profilers provide detailed information about memory allocation, object creation, and garbage collection, enabling developers to pinpoint memory-intensive areas of the code.
3. Monitoring Garbage Collection
Monitoring garbage collection can reveal insights into how the JVM is managing memory. Tools like JVisualVM or Java Mission Control can be used to monitor garbage collection activity, including the frequency of garbage collection cycles, the duration of each cycle, and the amount of memory reclaimed.
4. Using Memory Analyzers
Memory analyzers such as Eclipse Memory Analyzer (MAT) can be invaluable in identifying memory leaks and analyzing heap dumps. These tools can help identify potential sources of memory leaks, such as dangling references or excessively retained objects.
Best Practices for Memory Management
1. Efficient Data Structures and Algorithms
Using efficient data structures and algorithms can significantly reduce memory usage. For example, using a HashMap
instead of a HashTable
can provide better performance and lower memory overhead due to differences in synchronization.
// Example of using HashMap
Map<String, String> hashMap = new HashMap<>();
hashMap.put("key", "value");
2. Managing Large Data Sets
When working with large data sets, consider using streaming APIs or pagination to process data in smaller chunks, reducing memory pressure. Additionally, using libraries like Guava's Iterators
can help iterate over large collections without loading the entire data set into memory.
// Example of using Guava's Iterators
Iterator<String> iterator = Iterators.forArray("one", "two", "three");
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
3. Proper Resource Management
Ensure proper resource management by releasing resources such as file handles, database connections, and network sockets after use. Using try-with-resources in Java can help automatically close resources when they are no longer needed, preventing resource leaks.
// Example of using try-with-resources for file handling
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
String line = reader.readLine();
// Process the file data
} catch (IOException e) {
// Handle the exception
}
The Last Word
Effective memory management is crucial for the performance and stability of Java applications. By understanding common memory-related issues, leveraging troubleshooting techniques, and following best practices for memory management, developers can effectively identify and resolve memory problems in their Java applications.
Remember, monitoring, analyzing, and optimizing memory usage should be an ongoing process, especially for long-running or memory-intensive applications.
Incorporating tools, such as profilers and memory analyzers, can provide valuable insights into memory utilization, while adhering to best practices, such as efficient data structures and proper resource management, can help mitigate memory-related issues before they impact the application's performance.
By staying vigilant and proactive in memory management, developers can ensure their Java applications are efficient, stable, and performant, even under demanding workloads.
Now, armed with knowledge and best practices, tackle memory issues in your Java applications with confidence and finesse.
Keeping your applications running smoothly is not just a goal, but an achievable reality with the right tools and techniques at your disposal.
Checkout our other articles