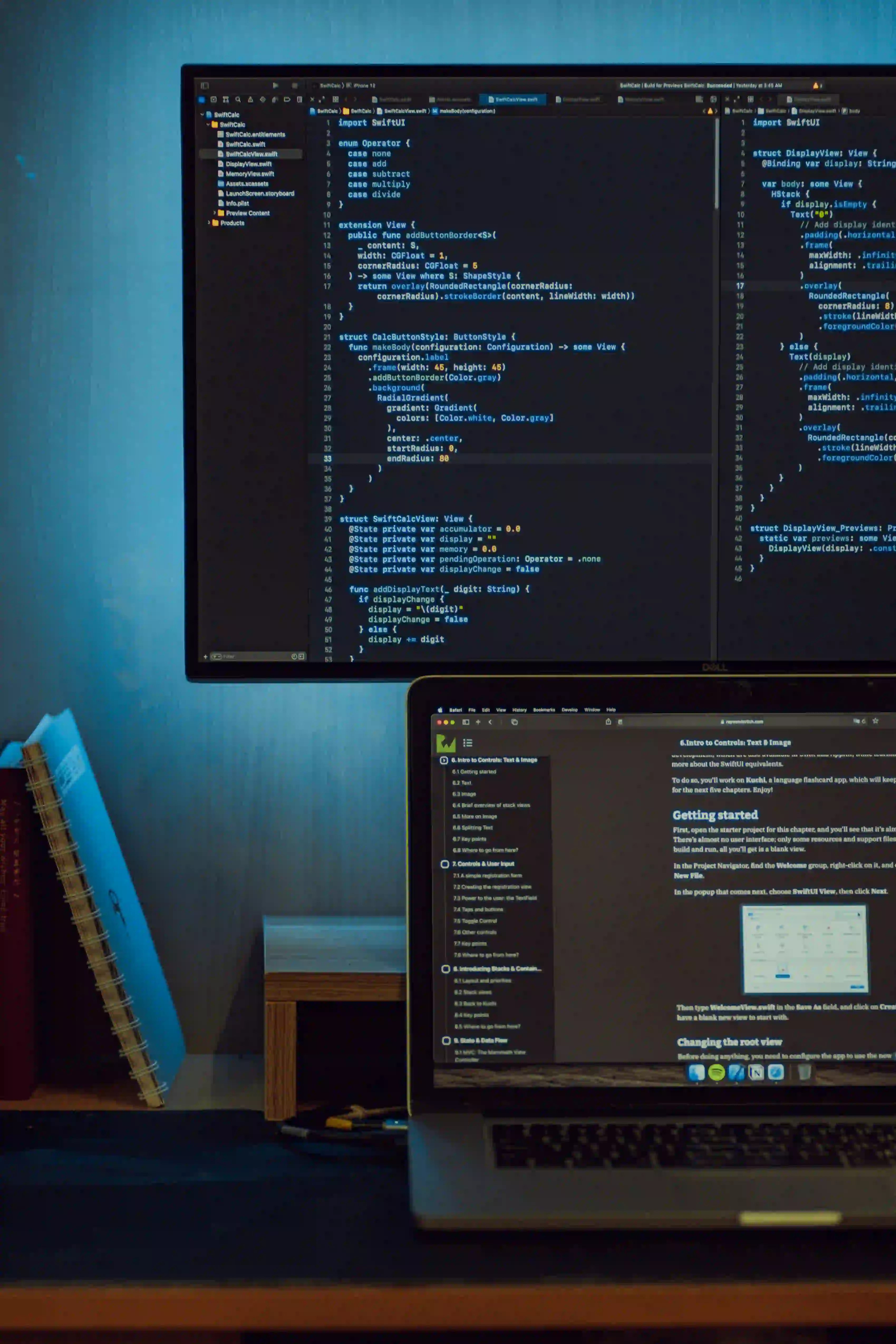
Implementing HMAC Authentication for Secure API Access
Security is paramount when it comes to API access. In this blog post, we will dive into the world of Hash-based Message Authentication Code (HMAC) authentication for securing API access using Java. HMAC adds an additional layer of security by requiring the sender and the receiver to both have access to a secret key. Let's explore how to implement HMAC authentication in Java for securing API access.
What is HMAC Authentication?
HMAC is a type of message authentication code (MAC) involving a cryptographic hash function and a secret key. It is used to verify the integrity and authenticity of a message. When applied to API access, HMAC requires the sender to create a hash of the request and send it along with the request. The receiver then recreates the hash using the same algorithm, secret key, and message, and compares it with the hash received from the sender. If the hashes match, the request is considered authentic.
Setting Up the Project
To begin, let's set up a new Java project using a build tool like Maven or Gradle. We'll use the javax.crypto
package, which provides the required classes for HMAC authentication. Additionally, we'll use a commonly used HTTP client library like OkHttp to make API requests.
// pom.xml (Maven)
<dependencies>
<!-- Other dependencies -->
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>4.9.0</version>
</dependency>
</dependencies>
Implementing HMAC Authentication
We'll create a class HmacAuth
that handles the HMAC authentication logic.
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.util.Base64;
public class HmacAuth {
private final String algorithm = "HmacSHA256";
private final String secretKey;
public HmacAuth(String secretKey) {
this.secretKey = secretKey;
}
public String calculateHmac(String message) throws NoSuchAlgorithmException, InvalidKeyException {
Mac mac = Mac.getInstance(algorithm);
SecretKeySpec keySpec = new SecretKeySpec(secretKey.getBytes(), algorithm);
mac.init(keySpec);
byte[] hmacBytes = mac.doFinal(message.getBytes());
return Base64.getEncoder().encodeToString(hmacBytes);
}
}
In the HmacAuth
class, we use the javax.crypto.Mac
class to calculate the HMAC. We provide the algorithm (in this case, HmacSHA256
) and the secret key. The calculateHmac
method takes the message, initializes the MAC with the secret key, and then calculates the HMAC, encoding it to Base64 before returning the result.
Making Authenticated API Requests
Now, let's make authenticated API requests using our HmacAuth
class and the OkHttp client.
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class ApiClient {
private final String baseUrl = "https://api.example.com";
private final String apiKey = "your_api_key";
private final String apiSecret = "your_api_secret";
private final HmacAuth hmacAuth;
public ApiClient() {
this.hmacAuth = new HmacAuth(apiSecret);
}
public String makeAuthenticatedRequest(String endpoint) throws Exception {
OkHttpClient client = new OkHttpClient();
String url = baseUrl + endpoint;
Request request = new Request.Builder()
.url(url)
.addHeader("X-API-KEY", apiKey)
.addHeader("X-SIGNATURE", hmacAuth.calculateHmac(endpoint))
.build();
Response response = client.newCall(request).execute();
return response.body().string();
}
}
In the ApiClient
class, we initialize the HmacAuth
class with the API secret key. When making the request, we add custom headers X-API-KEY
with the API key and X-SIGNATURE
with the calculated HMAC for the endpoint. This ensures that the request is authenticated using HMAC.
Wrapping Up
In this blog post, we've explored the implementation of HMAC authentication for securing API access in Java. By using HMAC, we add a layer of security by requiring both the sender and receiver to have access to a secret key. This ensures that the API requests are authentic and secure.
It's important to note that the secret key should be well protected and not exposed in client-side code to maintain the security of the HMAC authentication. Additionally, consider using a secure key management system to store and manage the secret keys.
By following the implementation discussed in this post, you can enhance the security of your API access by incorporating HMAC authentication into your Java-based applications.
Incorporating HMAC authentication provides a robust layer of security for API access in Java, a crucial aspect of modern application development. By following the steps outlined in this post, you can implement HMAC authentication with confidence, ensuring the safety and integrity of your API interactions.