Debunking Common Java Myths
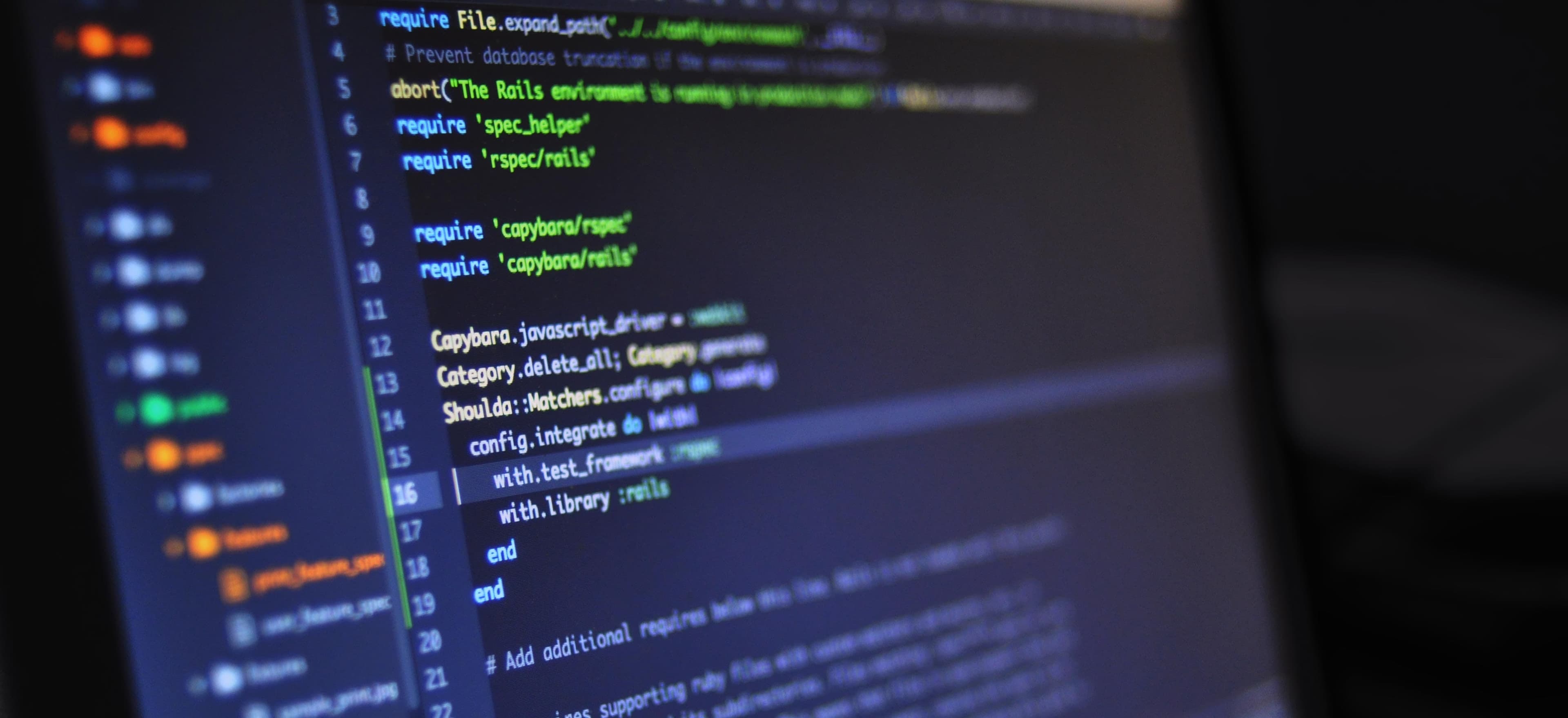
- Published on
Debunking Common Java Myths
Java is a powerful and versatile programming language that has been around for decades. However, like any popular technology, it has its fair share of myths and misconceptions. In this article, we'll debunk some of the most common myths surrounding Java and shed light on the truth behind this widely used language.
Myth 1: Java is Slow
One of the most enduring myths about Java is its perceived slowness. While it's true that Java was once criticized for its performance, the reality is that modern Java runtime environments have made significant strides in optimizing performance. The introduction of the Just-In-Time (JIT) compiler and runtime bytecode optimization has greatly improved Java's speed. Additionally, the underlying hardware has also advanced, making the impact of Java's performance overhead negligible in most cases.
Code Example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
In this simple "Hello, World!" example, the Java program executes efficiently, dispelling the myth of Java's slowness.
Myth 2: Java is Outdated
Another common misconception is that Java is outdated and no longer relevant in today's fast-paced technology landscape. However, Java remains a cornerstone of enterprise software development and is actively maintained and updated by Oracle and the Java community. The release of Java 16 in March 2021 demonstrates the continuous evolution of the language with new features and enhancements, reaffirming its contemporary relevance.
Code Example:
import java.util.List;
public class Example {
public static void main(String[] args) {
List<String> languages = List.of("Java", "Python", "JavaScript");
System.out.println("Popular programming languages: " + languages);
}
}
The usage of the List.of
method, introduced in Java 9, showcases the language's modern features, debunking the myth of Java being outdated.
Myth 3: Java is Only for Enterprise Applications
While Java has long been associated with enterprise software, it is not limited to that domain. Java's versatility allows it to be used in a wide range of applications, including mobile app development (Android), scientific computing, financial services, and more. Moreover, with the rise of microservices architecture and cloud-native applications, Java remains a popular choice due to its robustness, scalability, and mature ecosystem.
Code Example:
public class Calculator {
public static int add(int a, int b) {
return a + b;
}
}
This simple Calculator
class demonstrates that Java is not exclusively for enterprise use and can be applied in various contexts.
Myth 4: Java is Verbos
While Java has a reputation for being verbose compared to some modern languages, this perception is often exaggerated. With the introduction of features like lambdas, streams, and the var keyword in recent Java releases, the language has become more expressive and concise. These enhancements reduce boilerplate code and improve readability without compromising the underlying robustness and static typing that Java is known for.
Code Example:
import java.util.stream.IntStream;
public class Example {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
int sum = IntStream.of(numbers).sum();
System.out.println("Sum: " + sum);
}
}
The use of the IntStream and lambda expression for calculating the sum exemplifies Java's improved conciseness, debunking the myth of its verbosity.
Myth 5: Java is Insecure
There is a misconception that Java is inherently insecure due to its historical security vulnerabilities. However, the security landscape has evolved, and modern Java implementations incorporate robust security features. With built-in mechanisms such as the Security Manager, Secure Socket Extension (JSSE), and regular security updates from Oracle, Java is as secure as any other mature programming language.
Code Example:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class HashingExample {
public static void main(String[] args) {
String input = "SecretPassword123";
try {
MessageDigest digest = MessageDigest.getInstance("SHA-256");
byte[] hashedBytes = digest.digest(input.getBytes());
System.out.println("Hashed result: " + bytesToHex(hashedBytes));
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
}
private static String bytesToHex(byte[] bytes) {
StringBuilder result = new StringBuilder();
for (byte b : bytes) {
result.append(String.format("%02x", b));
}
return result.toString();
}
}
The usage of the MessageDigest class for hashing demonstrates Java's security capabilities, refuting the myth of its inherent insecurity.
Bringing It All Together
In conclusion, Java has transcended many of the myths and misconceptions that have surrounded it. With continuous advancements in performance, modern features, diverse application domains, improved conciseness, and robust security, Java remains a powerhouse in the world of programming languages. It is essential to revisit and update our understanding of Java to appreciate its enduring relevance and evolution in today's technology landscape.
In the dynamic realm of technology, it's vital to distinguish between myths and reality, especially when it comes to a foundational language like Java.
So, the next time you come across these myths, remember the truth behind Java's capabilities!