Optimizing Database Performance with a Superior Query Language
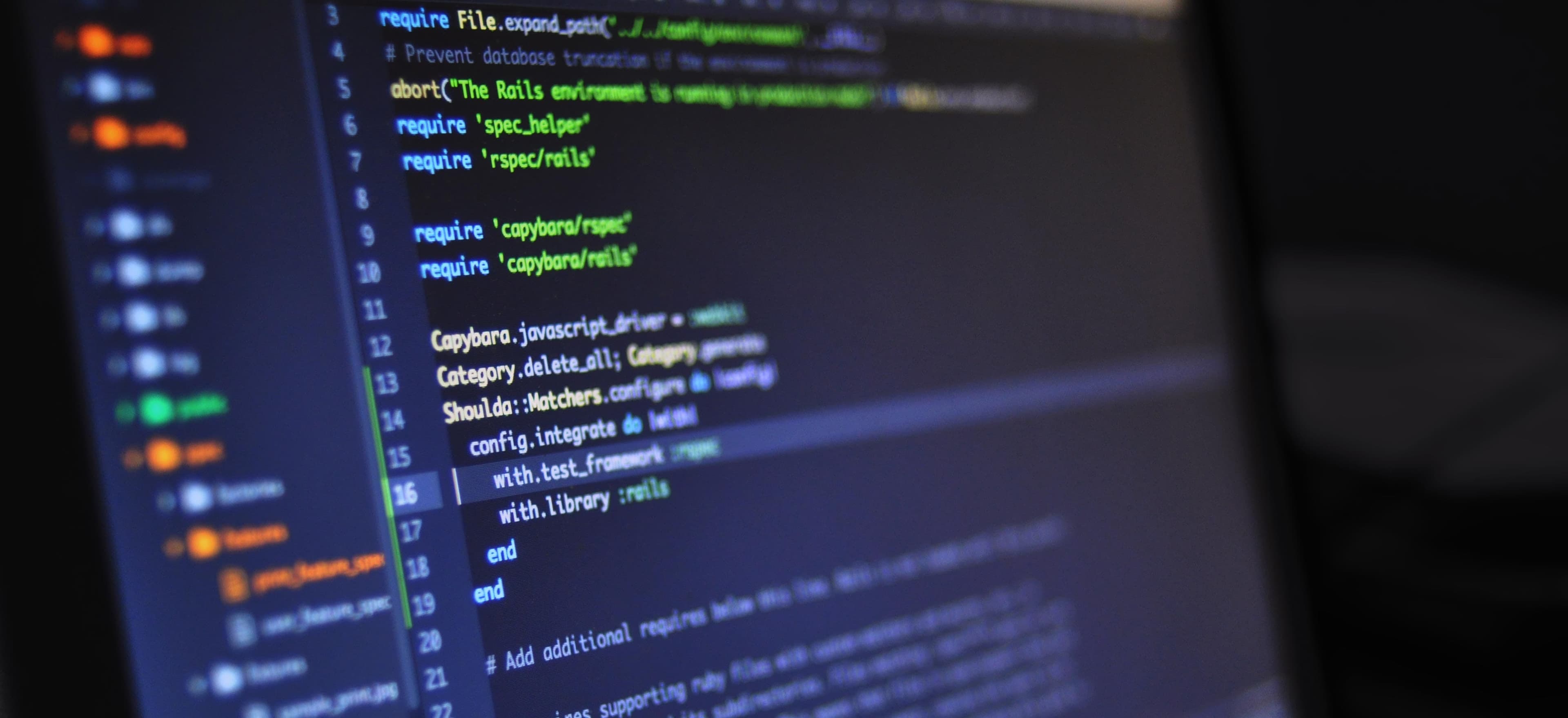
- Published on
Optimizing Database Performance with a Superior Query Language: An In-Depth Look at Java's Hibernate Query Language (HQL)
When it comes to working with databases in Java applications, optimizing performance is always a top priority. One of the key factors in achieving optimal performance is the ability to write efficient and effective database queries. In this blog post, we will explore how Java developers can leverage Hibernate Query Language (HQL) to optimize database performance.
Understanding Hibernate Query Language (HQL)
Hibernate is a popular object-relational mapping (ORM) framework for Java applications. It provides a powerful way to map Java objects to database tables and vice versa. With Hibernate, developers can write queries using Hibernate Query Language (HQL), which is an object-oriented query language similar to SQL.
Why Use HQL?
While SQL is a powerful and widely used query language, HQL offers several advantages when working with Hibernate and Java applications:
-
Object-Oriented Approach: HQL allows developers to write queries in a more object-oriented manner, working with entities and their properties directly.
-
Database Independence: HQL provides a database-independent way to perform database operations, allowing developers to write queries that can be executed on different database management systems without modification.
-
Type Safety: HQL queries are type-safe, which means that potential errors in query syntax can be caught at compile time rather than at runtime.
Writing Efficient Queries with HQL
Now, let's delve into some best practices for writing efficient and optimized queries using HQL.
1. Retrieving Specific Columns
When querying the database, it's best to select only the columns that are needed. This reduces the amount of data transferred between the database and the application, leading to improved performance.
Query query = session.createQuery("SELECT e.firstName, e.lastName FROM Employee e");
List<Object[]> results = query.list();
for (Object[] row : results) {
System.out.println("First Name: " + row[0] + ", Last Name: " + row[1]);
}
In this example, the query retrieves only the first name and last name of employees, optimizing the data retrieval process.
2. Avoiding N+1 Select Issue
The N+1 select issue occurs when an application makes N additional database queries to retrieve related entities, leading to poor performance. HQL provides ways to address this issue using fetch joins.
Query query = session.createQuery("FROM Department d JOIN FETCH d.employees");
List<Department> departments = query.list();
for (Department department : departments) {
System.out.println("Department: " + department.getName());
for (Employee employee : department.getEmployees()) {
System.out.println("Employee: " + employee.getFirstName() + " " + employee.getLastName());
}
}
In this example, the fetch join ensures that both the department and its employees are retrieved in a single query, avoiding the N+1 select problem.
3. Using Parameters in Queries
HQL supports parameterized queries, which can help prevent SQL injection attacks and improve query caching.
String lastName = "Smith";
Query query = session.createQuery("FROM Employee e WHERE e.lastName = :lastName");
query.setParameter("lastName", lastName);
List<Employee> employees = query.list();
for (Employee employee : employees) {
System.out.println("Employee: " + employee.getFirstName() + " " + employee.getLastName());
}
By using parameters, the query becomes more secure and can be reused with different values, leading to better performance through query caching.
Additional Tips for Optimizing HQL Queries
In addition to the aforementioned best practices, there are some additional tips for optimizing HQL queries:
-
Indexing: Ensure that the database tables being queried are properly indexed to improve query performance.
-
Batch Fetching: Consider using batch fetching to reduce the number of database round trips when loading associations.
-
Query Cache: Leverage the query cache to cache the results of frequently executed queries, reducing the workload on the database.
Lessons Learned
In conclusion, optimizing database performance in Java applications is crucial for delivering a responsive and scalable user experience. By utilizing Hibernate Query Language (HQL) and adhering to best practices such as retrieving specific columns, addressing the N+1 select issue, and using parameterized queries, developers can significantly enhance the efficiency and effectiveness of database operations. Additionally, following additional tips such as indexing, batch fetching, and query caching can further improve database performance.
As a Java developer, mastering HQL empowers you to fine-tune database interactions, ultimately leading to faster, more efficient, and scalable applications.
By implementing these best practices and tips, Java developers can achieve superior database performance, contributing to the overall success of their applications.
So, leverage the power of Hibernate Query Language and take your database performance optimization to the next level!