Troubleshooting Latency Issues in Scaling Java Microservices
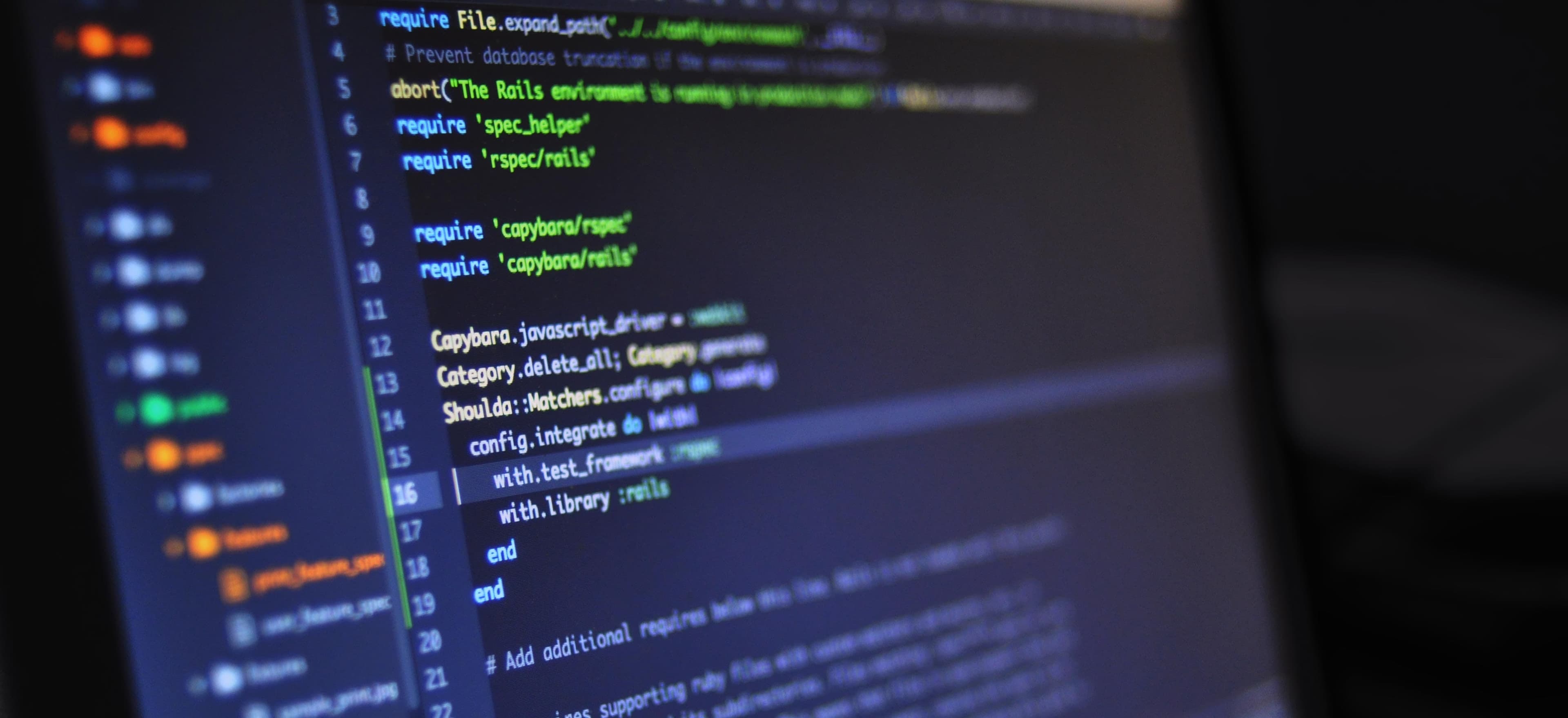
- Published on
Troubleshooting Latency Issues in Scaling Java Microservices
In the world of microservices, where applications are composed of small, independent processes communicating with each other over a network, latency issues can often crop up, especially when scaling the services. In this blog post, we will delve into the realm of troubleshooting latency issues in scaling Java microservices. We will explore various strategies and best practices to identify and address latency problems in a scalable Java microservices architecture.
Understanding Latency Issues in Scaling Java Microservices
When scaling Java microservices, latency can become a significant concern. As the number of service instances grows, the interactions between these instances can introduce network latency, leading to slower response times and degraded performance. Latency can be caused by a multitude of factors, such as network congestion, inefficient communication protocols, or poor resource allocation.
Monitoring and Profiling
The first step in troubleshooting latency issues is to have proper monitoring and profiling mechanisms in place. Tools such as Spring Boot Actuator and Micrometer can provide valuable insights into the performance of your Java microservices. By monitoring key metrics such as response times, throughput, and error rates, you can pinpoint the services or components experiencing latency.
Code Profiling with VisualVM
Let's take a look at how code profiling can help in identifying latency hotspots. Using VisualVM, a powerful profiling tool included in the JDK, we can analyze the performance of our Java microservices. The following code snippet illustrates how to enable JMX monitoring in a Spring Boot application:
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
MBeanServer mBeanServer = ManagementFactory.getPlatformMBeanServer();
ManagementFactory.getPlatformMBeanServer().registerMBean(new SomeClass(), new ObjectName("com.example:type=SomeClass"));
}
}
By enabling JMX monitoring, we can connect VisualVM to our running application and analyze CPU and memory usage, thread behavior, and method-level profiling to identify performance bottlenecks leading to latency issues.
Load Balancing and Circuit Breakers
Another aspect to consider when troubleshooting latency in scalable Java microservices is load balancing and circuit breaking. Load balancing distributes incoming network traffic across multiple service instances, preventing any single instance from becoming overwhelmed. This can help in mitigating latency issues by efficiently distributing the load across the service instances.
Implementing Load Balancing with Ribbon
In a microservices architecture, Netflix Ribbon provides client-side load balancing that can be seamlessly integrated into Java applications. By configuring a load balancer using Ribbon, we can ensure that requests are evenly distributed across the available instances, thus reducing the likelihood of latency due to overloaded services.
@RestClient
public interface MyServiceClient {
@GetMapping("/endpoint")
String getData();
}
@Configuration
public class MyServiceClientConfiguration {
@Bean
public MyServiceClient myServiceClient() {
return WebClient.builder()
.baseUrl("http://my-service")
.filter(RibbonLoadBalancedFilterFunction.create(myLoadBalancerClient()))
.build()
.build(MyServiceClient.class);
}
}
Circuit breakers also play a crucial role in mitigating latency issues. By using circuit breakers such as Hystrix, we can prevent cascading failures in a microservices environment. If a service instance is experiencing latency or unresponsiveness, the circuit breaker can open, diverting traffic away from the problematic instance until it has recovered.
Caching and Database Optimization
In a scaled microservices architecture, optimizing data access can significantly impact latency. Caching frequently accessed data can reduce the need for repeated database queries, thereby improving overall performance and reducing latency. Implementing caching strategies, such as using Redis or Memcached, can alleviate the burden on backend database systems and contribute to lower response times.
Implementing Caching with Spring's Cache Abstraction
Spring provides a powerful caching abstraction that can be easily integrated into Java microservices. By annotating methods with @Cacheable
and configuring a caching provider, such as Caffeine or Ehcache, we can seamlessly introduce caching to alleviate database load and reduce latency.
@Service
public class MyDataService {
@Cacheable("myDataCache")
public String getDataById(Long id) {
// Database query to fetch data
}
}
Database optimization also plays a vital role in addressing latency in scaled microservices. Techniques such as indexing frequently queried fields, optimizing database queries, and implementing proper database sharding can contribute to improved response times and reduced latency.
The Last Word
Troubleshooting latency issues in scaling Java microservices requires a comprehensive approach that encompasses monitoring, load balancing, caching, and database optimization. By leveraging the right tools and strategies, such as code profiling, load balancing with Ribbon, implementing circuit breakers, caching, and database optimization, you can effectively identify and address latency issues in a scalable Java microservices architecture.
In conclusion, optimizing performance and mitigating latency in scaled microservices can lead to enhanced user experience, improved system reliability, and overall efficient utilization of resources.
Remember, in the ever-evolving landscape of microservices, continuous monitoring and optimization are key to ensuring that latency issues are promptly identified and addressed, paving the way for a seamless and responsive microservices ecosystem.