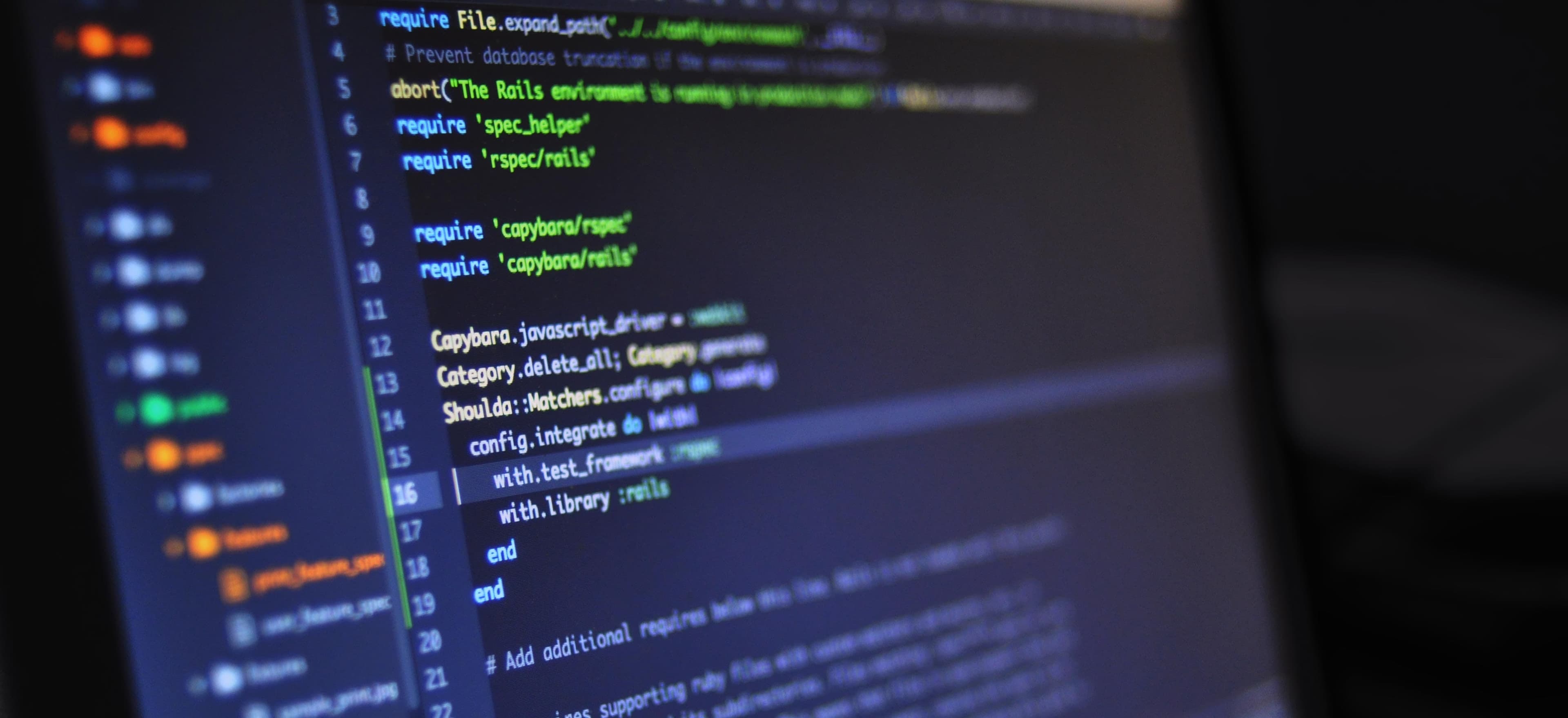
- Published on
Celebrate Your Fellow Developers with Unique Greeting Cards
As a Java developer, you're well aware of the power, elegance, and flexibility that the language offers. However, have you ever thought about expressing your appreciation for your fellow developers through a creative avenue like greeting cards? If not, then this blog post is for you! We'll explore the art of creating memorable developer greeting cards using Java programming language. So, let's dive in and explore how you can infuse your passion for Java into creating unique and personalized greeting cards for your tech-savvy friends and colleagues.
Crafting a Personalized Greeting Card in Java
Step 1: Choose Your Java Library
When it comes to creating graphics and working with images in Java, the Java Graphics2D library is a powerful tool. It allows you to draw and manipulate shapes, text, and images with precision.
Step 2: Setting Up the Project
Before diving into the code, ensure that you have the necessary tools in place. If you haven't already installed Java, you can refer to the official Java SE Development Kit for installation instructions.
Step 3: Writing the Java Code
Now, let's take a look at a simple example of how you can create a personalized greeting card in Java. Below is a snippet of code that demonstrates how to draw a birthday cake using Java Graphics2D library.
import java.awt.*;
import javax.swing.*;
public class GreetingCard extends JPanel {
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
// Draw the cake
g2d.setColor(Color.YELLOW);
g2d.fillRect(100, 150, 200, 150);
g2d.setColor(Color.ORANGE);
g2d.fillRect(130, 100, 140, 50);
g2d.setColor(Color.WHITE);
g2d.fillRect(170, 100, 10, 50);
// Add birthday message
g2d.setColor(Color.BLACK);
g2d.setFont(new Font("Arial", Font.BOLD, 20));
g2d.drawString("Happy Birthday!", 120, 80);
}
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.add(new GreetingCard());
frame.setSize(400, 400);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
In this code, we use the Graphics2D
class to draw shapes and text to create a simple birthday card with a cake and a birthday message.
Step 4: Running the Program
Compile and run the Java code to see the personalized greeting card come to life. You should see a window displaying the birthday cake greeting card with the message "Happy Birthday!"
By following these steps, you can create personalized greeting cards for various occasions using Java. With a bit of creativity and Java programming skills, you can tailor the greeting cards to suit the preferences of your fellow developers.
Adding Interactivity with JavaFX
To take your greeting cards to the next level, consider leveraging JavaFX to add interactive elements such as buttons, animations, and user input. JavaFX provides a rich set of features for creating modern and engaging user interfaces.
Below is an example of how you can enhance the previously created greeting card using JavaFX:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class GreetingCardFX extends Application {
@Override
public void start(Stage stage) {
StackPane root = new StackPane();
Scene scene = new Scene(root, 300, 250);
Button sendButton = new Button("Send Greeting");
root.getChildren().add(sendButton);
stage.setTitle("Interactive Greeting Card");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this JavaFX example, we create an interactive greeting card with a "Send Greeting" button. JavaFX enables you to design a more dynamic and engaging user experience compared to traditional Java graphics.
The Bottom Line
In this blog post, we explored how you can combine your Java programming skills with creativity to craft personalized greeting cards for developers. Whether it's a birthday, a milestone, or a simple thank-you gesture, showcasing your technical capabilities through unique greeting cards can truly resonate with your fellow coders.
So, why not roll up your sleeves, fire up your IDE, and start creating memorable developer greeting cards using the power of Java? Show your appreciation to the developers in your life in a way that's both creative and tech-savvy! Cheers to spreading joy through Java-powered greeting cards!