Optimizing Oracle ADF JET Architecture
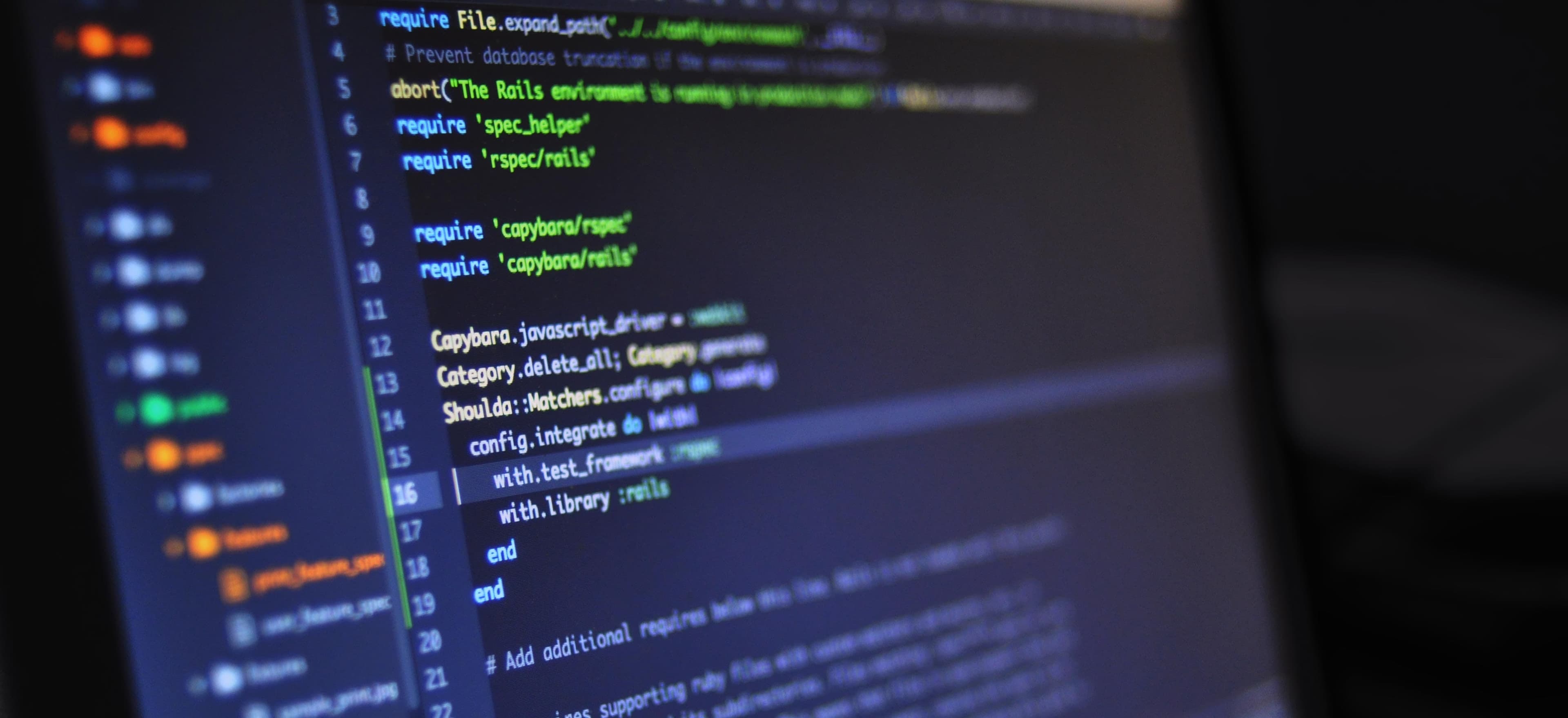
- Published on
Optimizing Oracle ADF JET Architecture for Performance
When it comes to building modern, responsive, and feature-rich web applications, Oracle Application Development Framework (ADF) and Oracle JavaScript Extension Toolkit (JET) provide a powerful combination. However, as with any technology stack, there are always opportunities for optimization to ensure the best performance. In this article, we'll dive into some key strategies for optimizing the architecture of Oracle ADF JET applications for improved performance.
Understanding Oracle ADF JET Architecture
Oracle ADF JET is designed to provide a modular, structured approach to building web applications using a combination of JavaScript, HTML, and CSS. The architecture of an ADF JET application typically includes components such as view models, templates, and modules, all of which contribute to the overall structure and behavior of the application.
Leveraging Lazy Loading
One important aspect of optimizing the architecture of an Oracle ADF JET application is to leverage lazy loading of resources. By deferring the loading of non-critical resources until they are actually needed, you can improve the initial load time of the application and provide a more responsive user experience.
define(['ojs/ojmodule'], function (ojModule) {
function loadModule(locator) {
return ojModule.createAsync({
url: locator.route,
params: locator.parameters
});
}
});
In the above code snippet, we use createAsync
to asynchronously load modules when needed, thereby improving the initial load time of the application.
Minification and Compression
Another crucial optimization technique is the minification and compression of JavaScript, HTML, and CSS resources. By reducing the size of these resources, you can significantly improve the download speed and overall performance of the application.
// Before minification
function calculateTotal(price, quantity) {
return price * quantity;
}
// After minification
function calculateTotal(c,b){return c*b}
The above example illustrates the reduction in size achieved through minification, which ultimately leads to faster download times.
Caching and Bundle Optimization
Caching and bundle optimization play a key role in enhancing the performance of Oracle ADF JET applications. By intelligently caching resources on the client side and optimizing resource bundles, you can reduce the number of round trips to the server and improve overall application speed.
// Define resource bundles for commonly used resources
var resources = {
'common': {
'js': ['jquery.min.js', 'knockout.min.js'],
'css': ['common.css']
},
'module1': {
'js': ['module1.js'],
'css': ['module1.css']
}
};
The above code snippet demonstrates how defining resource bundles can help optimize the loading and caching of resources in an ADF JET application.
Responsive Design and Image Optimization
In the context of Oracle ADF JET, incorporating responsive design principles and optimizing images are essential for delivering a fast and visually appealing user experience across various devices and screen sizes.
// Example of responsive design using media queries
@media only screen and (max-width: 600px) {
body {
font-size: 12px;
}
}
Additionally, optimizing images by resizing and compressing them can significantly reduce load times, especially on bandwidth-constrained connections.
Key Takeaways
Optimizing the architecture of Oracle ADF JET applications is crucial for delivering high-performance web applications. By leveraging techniques such as lazy loading, minification, caching, and responsive design, developers can ensure that their applications are fast, efficient, and provide a seamless user experience. As technologies continue to evolve, staying abreast of best practices and optimizing strategies will be essential for maintaining optimal performance in Oracle ADF JET applications.
To delve deeper into Oracle ADF JET optimization, refer to the official Oracle ADF JET documentation.
In conclusion, optimizing Oracle ADF JET architecture is not only about performance but also about delivering a top-notch user experience. By implementing the strategies outlined in this article, you can ensure that your Oracle ADF JET applications are finely tuned for speed, efficiency, and a delightful user experience.