Preventing SQL Injection: Safeguarding Your Database
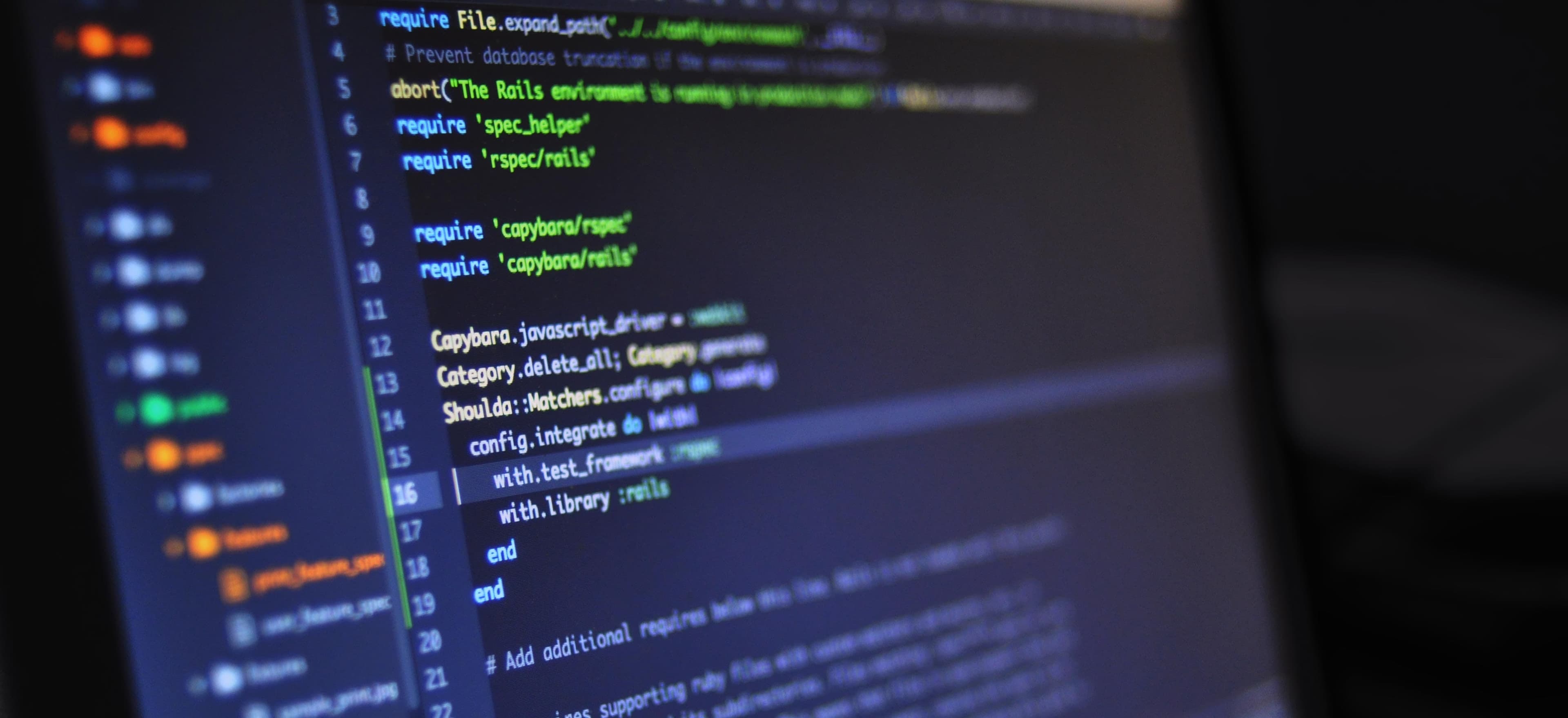
- Published on
Preventing SQL Injection: Safeguarding Your Database
When it comes to building secure and resilient applications, preventing SQL injection is of utmost importance. SQL injection attacks occur when a malicious actor inserts malicious SQL code into a query. This can lead to unauthorized access, data breaches, and potential damage to your database. As a Java developer, it is crucial to understand how to prevent SQL injection in your applications. In this article, we will explore the best practices and techniques to safeguard your database from these attacks.
Understanding SQL Injection
SQL injection is a type of security exploit in which an attacker executes malicious SQL statements. These statements are often injected into an entry field for execution (e.g., login form, search box) to gain unauthorized access to the underlying data. Consider the following vulnerable Java code:
String query = "SELECT * FROM users WHERE username='" + username + "' AND password='" + password + "'";
In this example, if the username
or password
input fields are manipulated with malicious SQL code, it can lead to a successful attack.
Using Prepared Statements
One of the most effective methods to prevent SQL injection in Java is using prepared statements. Instead of dynamically inserting user input directly into the SQL query, prepared statements utilize parameterized queries to separate the SQL code from the user input. This way, the database engine can distinguish between the actual SQL code and the user input, thus preventing any possibility of malicious SQL injection.
Here's an example of using prepared statements with JDBC:
String query = "SELECT * FROM users WHERE username=? AND password=?";
PreparedStatement preparedStatement = connection.prepareStatement(query);
preparedStatement.setString(1, username);
preparedStatement.setString(2, password);
ResultSet resultSet = preparedStatement.executeQuery();
In this code snippet, the ?
acts as a placeholder for the user input, which is then bound to the prepared statement using the setString
method.
Input Validation and Sanitization
Input validation plays a critical role in preventing SQL injection attacks. By validating and sanitizing user input, developers can ensure that only expected and safe data is used in SQL queries. There are libraries such as Apache Commons Text that provide convenient methods for input sanitization, including escaping special characters and ensuring the integrity of the input data.
String sanitizedUsername = StringEscapeUtils.escapeJava(username);
String sanitizedPassword = StringEscapeUtils.escapeJava(password);
Through input validation, potentially harmful characters or patterns can be detected and filtered out before the data is used in a SQL query. This serves as an additional layer of defense against SQL injection attacks.
Least Privilege Principle
Adhering to the least privilege principle is crucial in enhancing the security of your database. When configuring database access for the application, it is imperative to grant only the necessary privileges to the database user. Limiting the privileges reduces the potential impact of a successful SQL injection attack. For example, if the application does not require write access to certain tables, the database user associated with the application should not be granted such privileges.
Escaping Special Characters
In some cases, escaping special characters before using the input in SQL queries can provide an added layer of protection. While this approach is not as robust as using prepared statements, it can serve as a supplemental safeguard, especially when dealing with legacy codebases or frameworks where the migration to prepared statements is not immediately feasible.
String sanitizedInput = input.replace("'", "''");
However, it's important to note that relying solely on escaping characters may leave room for oversight and human error, making prepared statements the preferred method for preventing SQL injection attacks.
ORM Frameworks and Object Relational Mapping
Using Object Relational Mapping (ORM) frameworks such as Hibernate can contribute to preventing SQL injection. ORM frameworks abstract the interactions with the database, providing an additional layer of security by automatically sanitizing and escaping user input. By leveraging ORM frameworks, the risk of accidental SQL injection vulnerabilities is significantly reduced.
Here's an example of using Hibernate to perform parameterized queries:
String hql = "FROM User U WHERE U.username = :username AND U.password = :password";
Query query = session.createQuery(hql);
query.setParameter("username", username);
query.setParameter("password", password);
List results = query.list();
ORM frameworks not only enhance security but also offer improved maintainability and readability of the codebase.
Regular Security Audits
Conducting regular security audits and code reviews is essential in identifying and addressing potential SQL injection vulnerabilities. By performing thorough security assessments, developers can proactively eliminate any weak points in the application code that might lead to SQL injection attacks. Additionally, staying informed about the latest security best practices and vulnerabilities is crucial in ensuring the robustness of your defenses against SQL injection.
Key Takeaways
In conclusion, safeguarding your database from SQL injection attacks is an ongoing responsibility for Java developers. By employing best practices such as using prepared statements, input validation, least privilege principle, and ORM frameworks, the risk of SQL injection can be significantly mitigated. It is essential to prioritize security at every stage of application development and adhere to the principle of defense in depth. By staying vigilant and proactive, developers can build secure and resilient applications that protect sensitive data from malicious exploitation.
Preventing SQL injection is not an isolated task but a continuous commitment to ensuring the integrity and security of your application's database. As the threat landscape evolves, so should your defensive measures against SQL injection and other security vulnerabilities. Stay informed, stay proactive, and keep your database safe from harm.