Troubleshooting Common Errors in JavaParser Code Modification
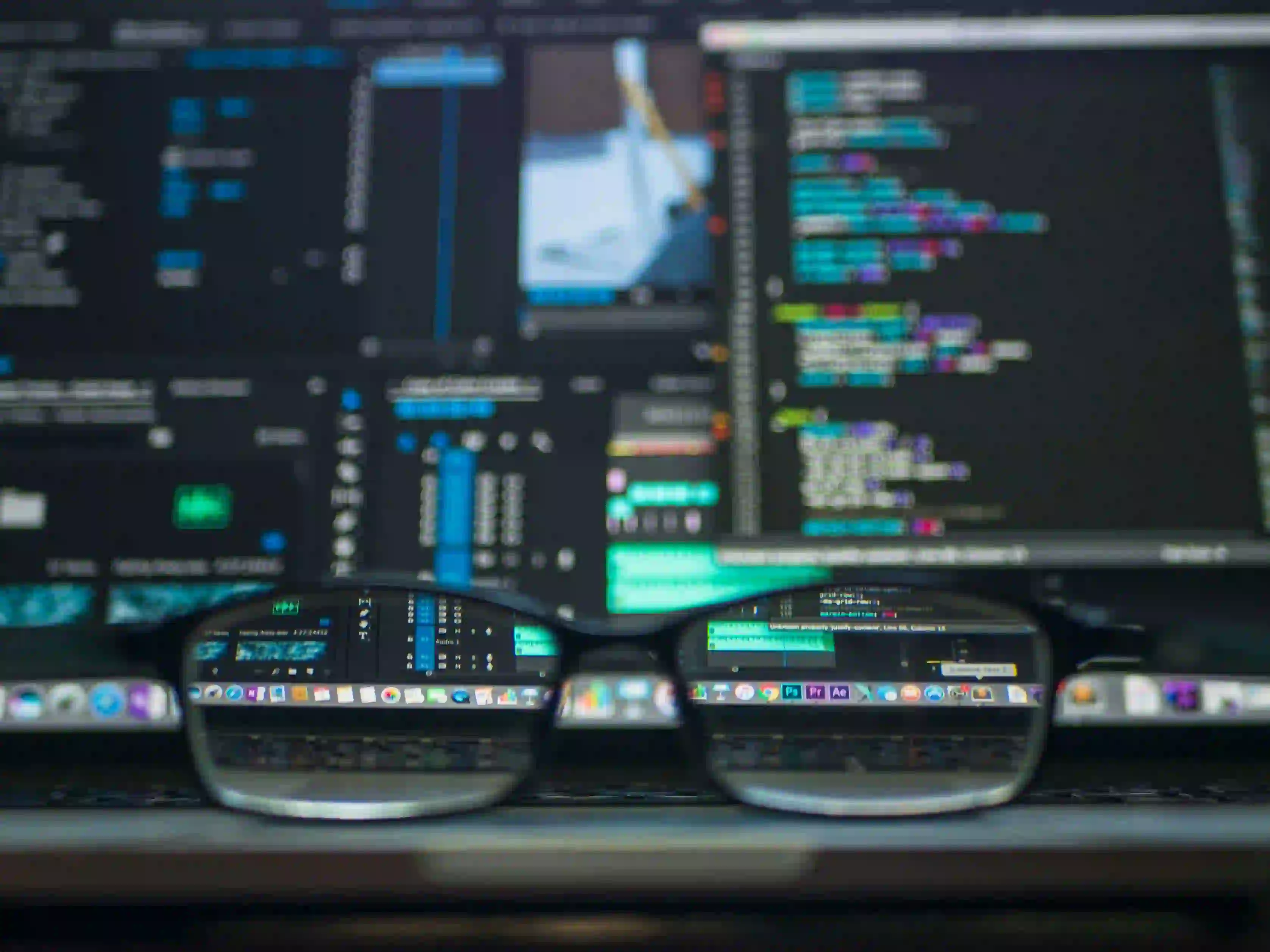
Troubleshooting Common Errors in JavaParser Code Modification
JavaParser is a powerful library that allows developers to parse, analyze, and modify Java code. However, working with JavaParser can sometimes lead to common errors that can be frustrating for developers. In this article, we will discuss some of these common errors and how to troubleshoot them effectively.
1. Error: Parsing code with JavaParser
When working with JavaParser, the first step is usually to parse the existing Java code. The following snippet of code demonstrates how to parse a Java file using JavaParser:
try {
CompilationUnit cu = JavaParser.parse(new File("Example.java"));
// Perform operations on the CompilationUnit
} catch (FileNotFoundException | ParseProblemException e) {
// Handle the exception
}
Here, we use the JavaParser.parse
method to parse the Java file "Example.java." However, it's important to handle any potential exceptions that may occur during the parsing process. The ParseProblemException
can be thrown if there are any issues with parsing the code.
2. Error: Modifying the parsed code
Once the code is parsed, developers often need to modify it in various ways. For example, let's consider the task of adding a new method to the parsed CompilationUnit
. The following code demonstrates how this can be achieved:
MethodDeclaration newMethod = cu.addMethod("public void newMethod() {}");
// Perform additional operations with the newMethod
This code adds a new method to the CompilationUnit
object cu
. However, it's worth noting that the addMethod
method may throw a NullPointerException
if the cu
object is not initialized properly.
3. Error: Navigating the AST
When modifying Java code using JavaParser, developers often need to navigate the Abstract Syntax Tree (AST) to identify specific elements, such as methods, classes, or variables. Consider the following code, which demonstrates how to retrieve all the method declarations from the parsed CompilationUnit
:
List<MethodDeclaration> methods = cu.findAll(MethodDeclaration.class);
// Iterate through the list of methods and perform operations
While this code seems straightforward, developers may encounter a NullPointerException
if the cu
object does not contain any method declarations.
4. Error: Code Generation
In some cases, developers may need to generate Java code using JavaParser. This can be done using the toString
method of various AST elements. For example, the following code snippet generates the String representation of a CompilationUnit
:
String code = cu.toString();
// Use the generated code as needed
However, it's important to note that the toString
method may not always produce the desired code representation, especially when working with complex and deeply nested AST structures.
5. Best Practices for Troubleshooting
To effectively troubleshoot common errors in JavaParser code modification, developers can follow these best practices:
- Proper error handling: Always use try-catch blocks to handle potential exceptions, such as
ParseProblemException
andNullPointerException
. - Defensive programming: Check for null references before performing any operations on AST elements to avoid
NullPointerException
errors. - Understanding the AST structure: Familiarize yourself with the structure of the AST to navigate it effectively and retrieve the desired elements without encountering errors.
My Closing Thoughts on the Matter
In conclusion, JavaParser is a valuable tool for parsing and modifying Java code. However, developers may encounter common errors when working with the library. By understanding these errors and following best practices for troubleshooting, developers can effectively overcome these challenges and utilize JavaParser to its full potential.
For more in-depth insights into JavaParser and its capabilities, you can refer to the official JavaParser documentation and the JavaParser GitHub repository.
By addressing common errors and providing best practices for troubleshooting, this post aims to empower developers to leverage JavaParser effectively in their code modification endeavors.