Optimizing Pagination for Couchbase - Best Practices
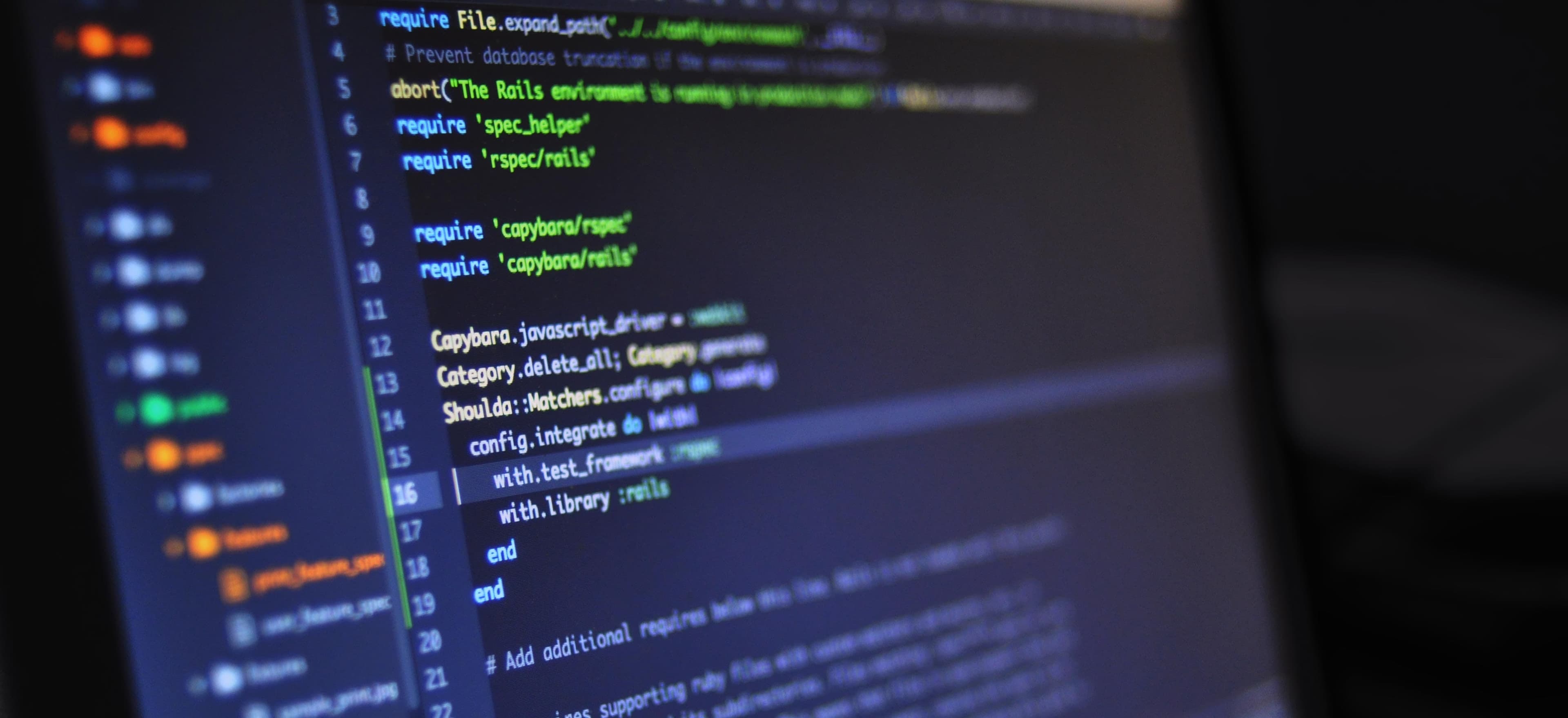
- Published on
Optimizing Pagination for Couchbase - Best Practices
When it comes to building scalable and performant applications, efficient pagination is crucial for providing a smooth user experience. In this blog post, we will explore the best practices for optimizing pagination with Couchbase, a powerful NoSQL database. We will delve into the key considerations, strategies, and code implementation to achieve optimal pagination performance in a Java environment.
Understanding Pagination
Pagination is the process of dividing content into discrete pages, making it easier for users to navigate through large datasets. In the context of databases, efficient pagination involves fetching and displaying a subset of data at a time, commonly achieved using LIMIT and OFFSET clauses in SQL-based systems. However, NoSQL databases like Couchbase require a different approach due to their distributed nature and scalability characteristics.
Key Considerations for Pagination in Couchbase
1. Use Keyset Pagination
In Couchbase, keyset pagination is preferred over the traditional OFFSET method. Instead of skipping a fixed number of records, keyset pagination relies on the use of indexed keys to navigate through the dataset. This approach ensures predictable and consistent query performance, especially when dealing with large result sets.
2. Utilize Covered Queries
To minimize the amount of data fetched from disk, leverage covered queries where the index includes all the fields needed for pagination. This helps in avoiding extra disk reads, resulting in improved query response times.
3. Handle Concurrent Data Modifications
When paginating through a dataset that is being actively modified, it is essential to handle the possibility of new items being inserted or existing ones being deleted or updated. This ensures the stability and consistency of the paginated results.
4. Optimize Indexes
Creating and maintaining appropriate indexes is critical for efficient pagination. Analyze the query patterns for pagination and design indexes that support those queries. Additionally, consider using composite indexes to cover multiple query conditions.
Implementing Pagination in Java with Couchbase
Now, let's dive into the Java code implementation for optimized pagination using the Couchbase Java SDK. We will walk through the steps to perform keyset pagination and address the considerations outlined earlier.
Setting Up the Couchbase Java SDK
First, ensure that you have added the Couchbase Java SDK as a dependency in your project. You can include the SDK using Maven by adding the following dependency to your pom.xml
file:
<dependency>
<groupId>com.couchbase.client</groupId>
<artifactId>java-client</artifactId>
<version>3.1.2</version>
</dependency>
Connecting to Couchbase Cluster
Establish a connection to the Couchbase cluster using the following code snippet:
Cluster cluster = Cluster.connect("your-cluster-url", "username", "password");
Bucket bucket = cluster.bucket("your-bucket-name");
Collection collection = bucket.defaultCollection();
Replace your-cluster-url
, username
, password
, and your-bucket-name
with your actual Couchbase cluster details.
Implementing Keyset Pagination
To implement keyset pagination in Couchbase, we can utilize the QueryOptions
class provided by the Couchbase Java SDK. Here's an example of how to perform keyset pagination with Java:
public List<Document> fetchNextPage(String lastKey, int pageSize) {
String query = "SELECT * FROM `your-bucket-name` WHERE condition AND meta().id > $lastKey ORDER BY meta().id LIMIT $pageSize";
JsonObject parameters = JsonObject.create().put("lastKey", lastKey).put("pageSize", pageSize);
QueryOptions options = QueryOptions.queryOptions().parameters(parameters);
QueryResult queryResult = cluster.query(query, options);
List<Document> documents = new ArrayList<>();
for (JsonObject row : queryResult.rowsAsObject()) {
// Map JsonObject to Document or custom POJO
Document document = mapToDocument(row);
documents.add(document);
}
return documents;
}
In this code snippet, we utilize the meta().id
field for keyset pagination, ensuring that we retrieve documents with IDs greater than the last key from the previous page. The fetchNextPage
method takes the last key from the previous page and the desired page size as parameters.
Handling Concurrent Data Modifications
To address concurrent data modifications during pagination, consider using techniques such as snapshot isolation or consistent scanning. These approaches ensure that the pagination results remain consistent, even when data changes are occurring concurrently.
My Closing Thoughts on the Matter
Optimizing pagination for Couchbase involves leveraging keyset pagination, covered queries, and efficient indexing strategies. By considering these best practices and implementing them in Java using the Couchbase Java SDK, developers can achieve high-performance pagination while efficiently handling concurrent data modifications.
In summary, when working with Couchbase and Java, prioritize keyset pagination, use covered queries, optimize indexes, and handle concurrent data modifications to ensure efficient and consistent pagination performance.
By following these best practices and utilizing the features of the Couchbase Java SDK, developers can build robust and responsive applications that scale seamlessly with large datasets.
Incorporating these pagination best practices will not only enhance the user experience but also contribute to the overall performance and scalability of Couchbase-powered applications.
Now that you're equipped with the best practices for optimizing pagination with Couchbase in a Java environment, it's time to apply these principles to your own projects and unlock the full potential of efficient and scalable pagination.
Remember, understanding the underlying mechanisms of Couchbase, coupled with effective Java code implementation, is key to achieving optimal pagination performance.
For further insights into Couchbase and Java development, explore the Couchbase Documentation and Java Development Best Practices.
Feel free to share your thoughts, experiences, and any additional tips for optimizing pagination with Couchbase in the comments below!